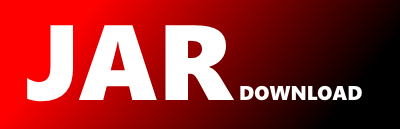
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.GcpMySqlSettings.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Settings in JSON format for the source GCP MySQL endpoint.
*/
public class GcpMySqlSettings private constructor(builder: Builder) {
/**
* Specifies a script to run immediately after DMS connects to the endpoint. The migration task continues running regardless if the SQL statement succeeds or fails.
*
* For this parameter, provide the code of the script itself, not the name of a file containing the script.
*/
public val afterConnectScript: kotlin.String? = builder.afterConnectScript
/**
* Cleans and recreates table metadata information on the replication instance when a mismatch occurs. For example, in a situation where running an alter DDL on the table could result in different information about the table cached in the replication instance.
*/
public val cleanSourceMetadataOnMismatch: kotlin.Boolean? = builder.cleanSourceMetadataOnMismatch
/**
* Database name for the endpoint. For a MySQL source or target endpoint, don't explicitly specify the database using the `DatabaseName` request parameter on either the `CreateEndpoint` or `ModifyEndpoint` API call. Specifying `DatabaseName` when you create or modify a MySQL endpoint replicates all the task tables to this single database. For MySQL endpoints, you specify the database only when you specify the schema in the table-mapping rules of the DMS task.
*/
public val databaseName: kotlin.String? = builder.databaseName
/**
* Specifies how often to check the binary log for new changes/events when the database is idle. The default is five seconds.
*
* Example: `eventsPollInterval=5;`
*
* In the example, DMS checks for changes in the binary logs every five seconds.
*/
public val eventsPollInterval: kotlin.Int? = builder.eventsPollInterval
/**
* Specifies the maximum size (in KB) of any .csv file used to transfer data to a MySQL-compatible database.
*
* Example: `maxFileSize=512`
*/
public val maxFileSize: kotlin.Int? = builder.maxFileSize
/**
* Improves performance when loading data into the MySQL-compatible target database. Specifies how many threads to use to load the data into the MySQL-compatible target database. Setting a large number of threads can have an adverse effect on database performance, because a separate connection is required for each thread. The default is one.
*
* Example: `parallelLoadThreads=1`
*/
public val parallelLoadThreads: kotlin.Int? = builder.parallelLoadThreads
/**
* Endpoint connection password.
*/
public val password: kotlin.String? = builder.password
/**
* Endpoint TCP port.
*/
public val port: kotlin.Int? = builder.port
/**
* The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants the required permissions to access the value in `SecretsManagerSecret.` The role must allow the `iam:PassRole` action. `SecretsManagerSecret` has the value of the Amazon Web Services Secrets Manager secret that allows access to the MySQL endpoint.
*
* You can specify one of two sets of values for these permissions. You can specify the values for this setting and `SecretsManagerSecretId`. Or you can specify clear-text values for `UserName`, `Password`, `ServerName`, and `Port`. You can't specify both. For more information on creating this `SecretsManagerSecret` and the `SecretsManagerAccessRoleArn` and `SecretsManagerSecretId` required to access it, see [Using secrets to access Database Migration Service resources](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Security.html#security-iam-secretsmanager) in the Database Migration Service User Guide.
*/
public val secretsManagerAccessRoleArn: kotlin.String? = builder.secretsManagerAccessRoleArn
/**
* The full ARN, partial ARN, or friendly name of the `SecretsManagerSecret` that contains the MySQL endpoint connection details.
*/
public val secretsManagerSecretId: kotlin.String? = builder.secretsManagerSecretId
/**
* The MySQL host name.
*/
public val serverName: kotlin.String? = builder.serverName
/**
* Specifies the time zone for the source MySQL database.
*
* Example: `serverTimezone=US/Pacific;`
*
* Note: Do not enclose time zones in single quotes.
*/
public val serverTimezone: kotlin.String? = builder.serverTimezone
/**
* Specifies where to migrate source tables on the target, either to a single database or multiple databases.
*
* Example: `targetDbType=MULTIPLE_DATABASES`
*/
public val targetDbType: aws.sdk.kotlin.services.databasemigrationservice.model.TargetDbType? = builder.targetDbType
/**
* Endpoint connection user name.
*/
public val username: kotlin.String? = builder.username
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.GcpMySqlSettings = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GcpMySqlSettings(")
append("afterConnectScript=$afterConnectScript,")
append("cleanSourceMetadataOnMismatch=$cleanSourceMetadataOnMismatch,")
append("databaseName=$databaseName,")
append("eventsPollInterval=$eventsPollInterval,")
append("maxFileSize=$maxFileSize,")
append("parallelLoadThreads=$parallelLoadThreads,")
append("password=*** Sensitive Data Redacted ***,")
append("port=$port,")
append("secretsManagerAccessRoleArn=$secretsManagerAccessRoleArn,")
append("secretsManagerSecretId=$secretsManagerSecretId,")
append("serverName=$serverName,")
append("serverTimezone=$serverTimezone,")
append("targetDbType=$targetDbType,")
append("username=$username")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = afterConnectScript?.hashCode() ?: 0
result = 31 * result + (cleanSourceMetadataOnMismatch?.hashCode() ?: 0)
result = 31 * result + (databaseName?.hashCode() ?: 0)
result = 31 * result + (eventsPollInterval ?: 0)
result = 31 * result + (maxFileSize ?: 0)
result = 31 * result + (parallelLoadThreads ?: 0)
result = 31 * result + (password?.hashCode() ?: 0)
result = 31 * result + (port ?: 0)
result = 31 * result + (secretsManagerAccessRoleArn?.hashCode() ?: 0)
result = 31 * result + (secretsManagerSecretId?.hashCode() ?: 0)
result = 31 * result + (serverName?.hashCode() ?: 0)
result = 31 * result + (serverTimezone?.hashCode() ?: 0)
result = 31 * result + (targetDbType?.hashCode() ?: 0)
result = 31 * result + (username?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GcpMySqlSettings
if (afterConnectScript != other.afterConnectScript) return false
if (cleanSourceMetadataOnMismatch != other.cleanSourceMetadataOnMismatch) return false
if (databaseName != other.databaseName) return false
if (eventsPollInterval != other.eventsPollInterval) return false
if (maxFileSize != other.maxFileSize) return false
if (parallelLoadThreads != other.parallelLoadThreads) return false
if (password != other.password) return false
if (port != other.port) return false
if (secretsManagerAccessRoleArn != other.secretsManagerAccessRoleArn) return false
if (secretsManagerSecretId != other.secretsManagerSecretId) return false
if (serverName != other.serverName) return false
if (serverTimezone != other.serverTimezone) return false
if (targetDbType != other.targetDbType) return false
if (username != other.username) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.GcpMySqlSettings = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Specifies a script to run immediately after DMS connects to the endpoint. The migration task continues running regardless if the SQL statement succeeds or fails.
*
* For this parameter, provide the code of the script itself, not the name of a file containing the script.
*/
public var afterConnectScript: kotlin.String? = null
/**
* Cleans and recreates table metadata information on the replication instance when a mismatch occurs. For example, in a situation where running an alter DDL on the table could result in different information about the table cached in the replication instance.
*/
public var cleanSourceMetadataOnMismatch: kotlin.Boolean? = null
/**
* Database name for the endpoint. For a MySQL source or target endpoint, don't explicitly specify the database using the `DatabaseName` request parameter on either the `CreateEndpoint` or `ModifyEndpoint` API call. Specifying `DatabaseName` when you create or modify a MySQL endpoint replicates all the task tables to this single database. For MySQL endpoints, you specify the database only when you specify the schema in the table-mapping rules of the DMS task.
*/
public var databaseName: kotlin.String? = null
/**
* Specifies how often to check the binary log for new changes/events when the database is idle. The default is five seconds.
*
* Example: `eventsPollInterval=5;`
*
* In the example, DMS checks for changes in the binary logs every five seconds.
*/
public var eventsPollInterval: kotlin.Int? = null
/**
* Specifies the maximum size (in KB) of any .csv file used to transfer data to a MySQL-compatible database.
*
* Example: `maxFileSize=512`
*/
public var maxFileSize: kotlin.Int? = null
/**
* Improves performance when loading data into the MySQL-compatible target database. Specifies how many threads to use to load the data into the MySQL-compatible target database. Setting a large number of threads can have an adverse effect on database performance, because a separate connection is required for each thread. The default is one.
*
* Example: `parallelLoadThreads=1`
*/
public var parallelLoadThreads: kotlin.Int? = null
/**
* Endpoint connection password.
*/
public var password: kotlin.String? = null
/**
* Endpoint TCP port.
*/
public var port: kotlin.Int? = null
/**
* The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants the required permissions to access the value in `SecretsManagerSecret.` The role must allow the `iam:PassRole` action. `SecretsManagerSecret` has the value of the Amazon Web Services Secrets Manager secret that allows access to the MySQL endpoint.
*
* You can specify one of two sets of values for these permissions. You can specify the values for this setting and `SecretsManagerSecretId`. Or you can specify clear-text values for `UserName`, `Password`, `ServerName`, and `Port`. You can't specify both. For more information on creating this `SecretsManagerSecret` and the `SecretsManagerAccessRoleArn` and `SecretsManagerSecretId` required to access it, see [Using secrets to access Database Migration Service resources](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Security.html#security-iam-secretsmanager) in the Database Migration Service User Guide.
*/
public var secretsManagerAccessRoleArn: kotlin.String? = null
/**
* The full ARN, partial ARN, or friendly name of the `SecretsManagerSecret` that contains the MySQL endpoint connection details.
*/
public var secretsManagerSecretId: kotlin.String? = null
/**
* The MySQL host name.
*/
public var serverName: kotlin.String? = null
/**
* Specifies the time zone for the source MySQL database.
*
* Example: `serverTimezone=US/Pacific;`
*
* Note: Do not enclose time zones in single quotes.
*/
public var serverTimezone: kotlin.String? = null
/**
* Specifies where to migrate source tables on the target, either to a single database or multiple databases.
*
* Example: `targetDbType=MULTIPLE_DATABASES`
*/
public var targetDbType: aws.sdk.kotlin.services.databasemigrationservice.model.TargetDbType? = null
/**
* Endpoint connection user name.
*/
public var username: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.GcpMySqlSettings) : this() {
this.afterConnectScript = x.afterConnectScript
this.cleanSourceMetadataOnMismatch = x.cleanSourceMetadataOnMismatch
this.databaseName = x.databaseName
this.eventsPollInterval = x.eventsPollInterval
this.maxFileSize = x.maxFileSize
this.parallelLoadThreads = x.parallelLoadThreads
this.password = x.password
this.port = x.port
this.secretsManagerAccessRoleArn = x.secretsManagerAccessRoleArn
this.secretsManagerSecretId = x.secretsManagerSecretId
this.serverName = x.serverName
this.serverTimezone = x.serverTimezone
this.targetDbType = x.targetDbType
this.username = x.username
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.GcpMySqlSettings = GcpMySqlSettings(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy