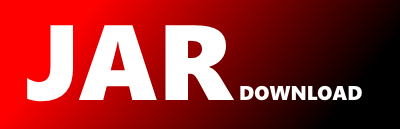
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.IbmDb2Settings.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Provides information that defines an IBM Db2 LUW endpoint.
*/
public class IbmDb2Settings private constructor(builder: Builder) {
/**
* For ongoing replication (CDC), use CurrentLSN to specify a log sequence number (LSN) where you want the replication to start.
*/
public val currentLsn: kotlin.String? = builder.currentLsn
/**
* Database name for the endpoint.
*/
public val databaseName: kotlin.String? = builder.databaseName
/**
* If true, DMS saves any .csv files to the Db2 LUW target that were used to replicate data. DMS uses these files for analysis and troubleshooting.
*
* The default value is false.
*/
public val keepCsvFiles: kotlin.Boolean? = builder.keepCsvFiles
/**
* The amount of time (in milliseconds) before DMS times out operations performed by DMS on the Db2 target. The default value is 1200 (20 minutes).
*/
public val loadTimeout: kotlin.Int? = builder.loadTimeout
/**
* Specifies the maximum size (in KB) of .csv files used to transfer data to Db2 LUW.
*/
public val maxFileSize: kotlin.Int? = builder.maxFileSize
/**
* Maximum number of bytes per read, as a NUMBER value. The default is 64 KB.
*/
public val maxKBytesPerRead: kotlin.Int? = builder.maxKBytesPerRead
/**
* Endpoint connection password.
*/
public val password: kotlin.String? = builder.password
/**
* Endpoint TCP port. The default value is 50000.
*/
public val port: kotlin.Int? = builder.port
/**
* The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants the required permissions to access the value in `SecretsManagerSecret`. The role must allow the `iam:PassRole` action. `SecretsManagerSecret` has the value of the Amazon Web Services Secrets Manager secret that allows access to the Db2 LUW endpoint.
*
* You can specify one of two sets of values for these permissions. You can specify the values for this setting and `SecretsManagerSecretId`. Or you can specify clear-text values for `UserName`, `Password`, `ServerName`, and `Port`. You can't specify both. For more information on creating this `SecretsManagerSecret` and the `SecretsManagerAccessRoleArn` and `SecretsManagerSecretId` required to access it, see [Using secrets to access Database Migration Service resources](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Security.html#security-iam-secretsmanager) in the *Database Migration Service User Guide*.
*/
public val secretsManagerAccessRoleArn: kotlin.String? = builder.secretsManagerAccessRoleArn
/**
* The full ARN, partial ARN, or friendly name of the `SecretsManagerSecret` that contains the Db2 LUW endpoint connection details.
*/
public val secretsManagerSecretId: kotlin.String? = builder.secretsManagerSecretId
/**
* Fully qualified domain name of the endpoint.
*/
public val serverName: kotlin.String? = builder.serverName
/**
* Enables ongoing replication (CDC) as a BOOLEAN value. The default is true.
*/
public val setDataCaptureChanges: kotlin.Boolean? = builder.setDataCaptureChanges
/**
* Endpoint connection user name.
*/
public val username: kotlin.String? = builder.username
/**
* The size (in KB) of the in-memory file write buffer used when generating .csv files on the local disk on the DMS replication instance. The default value is 1024 (1 MB).
*/
public val writeBufferSize: kotlin.Int? = builder.writeBufferSize
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.IbmDb2Settings = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("IbmDb2Settings(")
append("currentLsn=$currentLsn,")
append("databaseName=$databaseName,")
append("keepCsvFiles=$keepCsvFiles,")
append("loadTimeout=$loadTimeout,")
append("maxFileSize=$maxFileSize,")
append("maxKBytesPerRead=$maxKBytesPerRead,")
append("password=*** Sensitive Data Redacted ***,")
append("port=$port,")
append("secretsManagerAccessRoleArn=$secretsManagerAccessRoleArn,")
append("secretsManagerSecretId=$secretsManagerSecretId,")
append("serverName=$serverName,")
append("setDataCaptureChanges=$setDataCaptureChanges,")
append("username=$username,")
append("writeBufferSize=$writeBufferSize")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = currentLsn?.hashCode() ?: 0
result = 31 * result + (databaseName?.hashCode() ?: 0)
result = 31 * result + (keepCsvFiles?.hashCode() ?: 0)
result = 31 * result + (loadTimeout ?: 0)
result = 31 * result + (maxFileSize ?: 0)
result = 31 * result + (maxKBytesPerRead ?: 0)
result = 31 * result + (password?.hashCode() ?: 0)
result = 31 * result + (port ?: 0)
result = 31 * result + (secretsManagerAccessRoleArn?.hashCode() ?: 0)
result = 31 * result + (secretsManagerSecretId?.hashCode() ?: 0)
result = 31 * result + (serverName?.hashCode() ?: 0)
result = 31 * result + (setDataCaptureChanges?.hashCode() ?: 0)
result = 31 * result + (username?.hashCode() ?: 0)
result = 31 * result + (writeBufferSize ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as IbmDb2Settings
if (currentLsn != other.currentLsn) return false
if (databaseName != other.databaseName) return false
if (keepCsvFiles != other.keepCsvFiles) return false
if (loadTimeout != other.loadTimeout) return false
if (maxFileSize != other.maxFileSize) return false
if (maxKBytesPerRead != other.maxKBytesPerRead) return false
if (password != other.password) return false
if (port != other.port) return false
if (secretsManagerAccessRoleArn != other.secretsManagerAccessRoleArn) return false
if (secretsManagerSecretId != other.secretsManagerSecretId) return false
if (serverName != other.serverName) return false
if (setDataCaptureChanges != other.setDataCaptureChanges) return false
if (username != other.username) return false
if (writeBufferSize != other.writeBufferSize) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.IbmDb2Settings = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* For ongoing replication (CDC), use CurrentLSN to specify a log sequence number (LSN) where you want the replication to start.
*/
public var currentLsn: kotlin.String? = null
/**
* Database name for the endpoint.
*/
public var databaseName: kotlin.String? = null
/**
* If true, DMS saves any .csv files to the Db2 LUW target that were used to replicate data. DMS uses these files for analysis and troubleshooting.
*
* The default value is false.
*/
public var keepCsvFiles: kotlin.Boolean? = null
/**
* The amount of time (in milliseconds) before DMS times out operations performed by DMS on the Db2 target. The default value is 1200 (20 minutes).
*/
public var loadTimeout: kotlin.Int? = null
/**
* Specifies the maximum size (in KB) of .csv files used to transfer data to Db2 LUW.
*/
public var maxFileSize: kotlin.Int? = null
/**
* Maximum number of bytes per read, as a NUMBER value. The default is 64 KB.
*/
public var maxKBytesPerRead: kotlin.Int? = null
/**
* Endpoint connection password.
*/
public var password: kotlin.String? = null
/**
* Endpoint TCP port. The default value is 50000.
*/
public var port: kotlin.Int? = null
/**
* The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants the required permissions to access the value in `SecretsManagerSecret`. The role must allow the `iam:PassRole` action. `SecretsManagerSecret` has the value of the Amazon Web Services Secrets Manager secret that allows access to the Db2 LUW endpoint.
*
* You can specify one of two sets of values for these permissions. You can specify the values for this setting and `SecretsManagerSecretId`. Or you can specify clear-text values for `UserName`, `Password`, `ServerName`, and `Port`. You can't specify both. For more information on creating this `SecretsManagerSecret` and the `SecretsManagerAccessRoleArn` and `SecretsManagerSecretId` required to access it, see [Using secrets to access Database Migration Service resources](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Security.html#security-iam-secretsmanager) in the *Database Migration Service User Guide*.
*/
public var secretsManagerAccessRoleArn: kotlin.String? = null
/**
* The full ARN, partial ARN, or friendly name of the `SecretsManagerSecret` that contains the Db2 LUW endpoint connection details.
*/
public var secretsManagerSecretId: kotlin.String? = null
/**
* Fully qualified domain name of the endpoint.
*/
public var serverName: kotlin.String? = null
/**
* Enables ongoing replication (CDC) as a BOOLEAN value. The default is true.
*/
public var setDataCaptureChanges: kotlin.Boolean? = null
/**
* Endpoint connection user name.
*/
public var username: kotlin.String? = null
/**
* The size (in KB) of the in-memory file write buffer used when generating .csv files on the local disk on the DMS replication instance. The default value is 1024 (1 MB).
*/
public var writeBufferSize: kotlin.Int? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.IbmDb2Settings) : this() {
this.currentLsn = x.currentLsn
this.databaseName = x.databaseName
this.keepCsvFiles = x.keepCsvFiles
this.loadTimeout = x.loadTimeout
this.maxFileSize = x.maxFileSize
this.maxKBytesPerRead = x.maxKBytesPerRead
this.password = x.password
this.port = x.port
this.secretsManagerAccessRoleArn = x.secretsManagerAccessRoleArn
this.secretsManagerSecretId = x.secretsManagerSecretId
this.serverName = x.serverName
this.setDataCaptureChanges = x.setDataCaptureChanges
this.username = x.username
this.writeBufferSize = x.writeBufferSize
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.IbmDb2Settings = IbmDb2Settings(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy