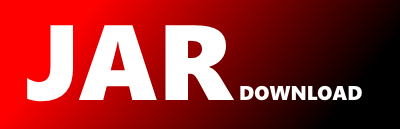
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.KinesisSettings.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Provides information that describes an Amazon Kinesis Data Stream endpoint. This information includes the output format of records applied to the endpoint and details of transaction and control table data information.
*/
public class KinesisSettings private constructor(builder: Builder) {
/**
* Shows detailed control information for table definition, column definition, and table and column changes in the Kinesis message output. The default is `false`.
*/
public val includeControlDetails: kotlin.Boolean? = builder.includeControlDetails
/**
* Include NULL and empty columns for records migrated to the endpoint. The default is `false`.
*/
public val includeNullAndEmpty: kotlin.Boolean? = builder.includeNullAndEmpty
/**
* Shows the partition value within the Kinesis message output, unless the partition type is `schema-table-type`. The default is `false`.
*/
public val includePartitionValue: kotlin.Boolean? = builder.includePartitionValue
/**
* Includes any data definition language (DDL) operations that change the table in the control data, such as `rename-table`, `drop-table`, `add-column`, `drop-column`, and `rename-column`. The default is `false`.
*/
public val includeTableAlterOperations: kotlin.Boolean? = builder.includeTableAlterOperations
/**
* Provides detailed transaction information from the source database. This information includes a commit timestamp, a log position, and values for `transaction_id`, previous `transaction_id`, and `transaction_record_id` (the record offset within a transaction). The default is `false`.
*/
public val includeTransactionDetails: kotlin.Boolean? = builder.includeTransactionDetails
/**
* The output format for the records created on the endpoint. The message format is `JSON` (default) or `JSON_UNFORMATTED` (a single line with no tab).
*/
public val messageFormat: aws.sdk.kotlin.services.databasemigrationservice.model.MessageFormatValue? = builder.messageFormat
/**
* Set this optional parameter to `true` to avoid adding a '0x' prefix to raw data in hexadecimal format. For example, by default, DMS adds a '0x' prefix to the LOB column type in hexadecimal format moving from an Oracle source to an Amazon Kinesis target. Use the `NoHexPrefix` endpoint setting to enable migration of RAW data type columns without adding the '0x' prefix.
*/
public val noHexPrefix: kotlin.Boolean? = builder.noHexPrefix
/**
* Prefixes schema and table names to partition values, when the partition type is `primary-key-type`. Doing this increases data distribution among Kinesis shards. For example, suppose that a SysBench schema has thousands of tables and each table has only limited range for a primary key. In this case, the same primary key is sent from thousands of tables to the same shard, which causes throttling. The default is `false`.
*/
public val partitionIncludeSchemaTable: kotlin.Boolean? = builder.partitionIncludeSchemaTable
/**
* The Amazon Resource Name (ARN) for the IAM role that DMS uses to write to the Kinesis data stream. The role must allow the `iam:PassRole` action.
*/
public val serviceAccessRoleArn: kotlin.String? = builder.serviceAccessRoleArn
/**
* The Amazon Resource Name (ARN) for the Amazon Kinesis Data Streams endpoint.
*/
public val streamArn: kotlin.String? = builder.streamArn
/**
* Specifies using the large integer value with Kinesis.
*/
public val useLargeIntegerValue: kotlin.Boolean? = builder.useLargeIntegerValue
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.KinesisSettings = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("KinesisSettings(")
append("includeControlDetails=$includeControlDetails,")
append("includeNullAndEmpty=$includeNullAndEmpty,")
append("includePartitionValue=$includePartitionValue,")
append("includeTableAlterOperations=$includeTableAlterOperations,")
append("includeTransactionDetails=$includeTransactionDetails,")
append("messageFormat=$messageFormat,")
append("noHexPrefix=$noHexPrefix,")
append("partitionIncludeSchemaTable=$partitionIncludeSchemaTable,")
append("serviceAccessRoleArn=$serviceAccessRoleArn,")
append("streamArn=$streamArn,")
append("useLargeIntegerValue=$useLargeIntegerValue")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = includeControlDetails?.hashCode() ?: 0
result = 31 * result + (includeNullAndEmpty?.hashCode() ?: 0)
result = 31 * result + (includePartitionValue?.hashCode() ?: 0)
result = 31 * result + (includeTableAlterOperations?.hashCode() ?: 0)
result = 31 * result + (includeTransactionDetails?.hashCode() ?: 0)
result = 31 * result + (messageFormat?.hashCode() ?: 0)
result = 31 * result + (noHexPrefix?.hashCode() ?: 0)
result = 31 * result + (partitionIncludeSchemaTable?.hashCode() ?: 0)
result = 31 * result + (serviceAccessRoleArn?.hashCode() ?: 0)
result = 31 * result + (streamArn?.hashCode() ?: 0)
result = 31 * result + (useLargeIntegerValue?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as KinesisSettings
if (includeControlDetails != other.includeControlDetails) return false
if (includeNullAndEmpty != other.includeNullAndEmpty) return false
if (includePartitionValue != other.includePartitionValue) return false
if (includeTableAlterOperations != other.includeTableAlterOperations) return false
if (includeTransactionDetails != other.includeTransactionDetails) return false
if (messageFormat != other.messageFormat) return false
if (noHexPrefix != other.noHexPrefix) return false
if (partitionIncludeSchemaTable != other.partitionIncludeSchemaTable) return false
if (serviceAccessRoleArn != other.serviceAccessRoleArn) return false
if (streamArn != other.streamArn) return false
if (useLargeIntegerValue != other.useLargeIntegerValue) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.KinesisSettings = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Shows detailed control information for table definition, column definition, and table and column changes in the Kinesis message output. The default is `false`.
*/
public var includeControlDetails: kotlin.Boolean? = null
/**
* Include NULL and empty columns for records migrated to the endpoint. The default is `false`.
*/
public var includeNullAndEmpty: kotlin.Boolean? = null
/**
* Shows the partition value within the Kinesis message output, unless the partition type is `schema-table-type`. The default is `false`.
*/
public var includePartitionValue: kotlin.Boolean? = null
/**
* Includes any data definition language (DDL) operations that change the table in the control data, such as `rename-table`, `drop-table`, `add-column`, `drop-column`, and `rename-column`. The default is `false`.
*/
public var includeTableAlterOperations: kotlin.Boolean? = null
/**
* Provides detailed transaction information from the source database. This information includes a commit timestamp, a log position, and values for `transaction_id`, previous `transaction_id`, and `transaction_record_id` (the record offset within a transaction). The default is `false`.
*/
public var includeTransactionDetails: kotlin.Boolean? = null
/**
* The output format for the records created on the endpoint. The message format is `JSON` (default) or `JSON_UNFORMATTED` (a single line with no tab).
*/
public var messageFormat: aws.sdk.kotlin.services.databasemigrationservice.model.MessageFormatValue? = null
/**
* Set this optional parameter to `true` to avoid adding a '0x' prefix to raw data in hexadecimal format. For example, by default, DMS adds a '0x' prefix to the LOB column type in hexadecimal format moving from an Oracle source to an Amazon Kinesis target. Use the `NoHexPrefix` endpoint setting to enable migration of RAW data type columns without adding the '0x' prefix.
*/
public var noHexPrefix: kotlin.Boolean? = null
/**
* Prefixes schema and table names to partition values, when the partition type is `primary-key-type`. Doing this increases data distribution among Kinesis shards. For example, suppose that a SysBench schema has thousands of tables and each table has only limited range for a primary key. In this case, the same primary key is sent from thousands of tables to the same shard, which causes throttling. The default is `false`.
*/
public var partitionIncludeSchemaTable: kotlin.Boolean? = null
/**
* The Amazon Resource Name (ARN) for the IAM role that DMS uses to write to the Kinesis data stream. The role must allow the `iam:PassRole` action.
*/
public var serviceAccessRoleArn: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) for the Amazon Kinesis Data Streams endpoint.
*/
public var streamArn: kotlin.String? = null
/**
* Specifies using the large integer value with Kinesis.
*/
public var useLargeIntegerValue: kotlin.Boolean? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.KinesisSettings) : this() {
this.includeControlDetails = x.includeControlDetails
this.includeNullAndEmpty = x.includeNullAndEmpty
this.includePartitionValue = x.includePartitionValue
this.includeTableAlterOperations = x.includeTableAlterOperations
this.includeTransactionDetails = x.includeTransactionDetails
this.messageFormat = x.messageFormat
this.noHexPrefix = x.noHexPrefix
this.partitionIncludeSchemaTable = x.partitionIncludeSchemaTable
this.serviceAccessRoleArn = x.serviceAccessRoleArn
this.streamArn = x.streamArn
this.useLargeIntegerValue = x.useLargeIntegerValue
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.KinesisSettings = KinesisSettings(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy