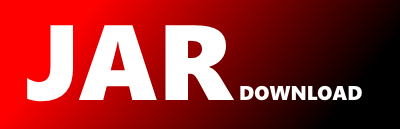
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.Limitation.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Provides information about the limitations of target Amazon Web Services engines.
*
* Your source database might include features that the target Amazon Web Services engine doesn't support. Fleet Advisor lists these features as limitations. You should consider these limitations during database migration. For each limitation, Fleet Advisor recommends an action that you can take to address or avoid this limitation.
*/
public class Limitation private constructor(builder: Builder) {
/**
* The identifier of the source database.
*/
public val databaseId: kotlin.String? = builder.databaseId
/**
* A description of the limitation. Provides additional information about the limitation, and includes recommended actions that you can take to address or avoid this limitation.
*/
public val description: kotlin.String? = builder.description
/**
* The name of the target engine that Fleet Advisor should use in the target engine recommendation. Valid values include `"rds-aurora-mysql"`, `"rds-aurora-postgresql"`, `"rds-mysql"`, `"rds-oracle"`, `"rds-sql-server"`, and `"rds-postgresql"`.
*/
public val engineName: kotlin.String? = builder.engineName
/**
* The impact of the limitation. You can use this parameter to prioritize limitations that you want to address. Valid values include `"Blocker"`, `"High"`, `"Medium"`, and `"Low"`.
*/
public val impact: kotlin.String? = builder.impact
/**
* The name of the limitation. Describes unsupported database features, migration action items, and other limitations.
*/
public val name: kotlin.String? = builder.name
/**
* The type of the limitation, such as action required, upgrade required, and limited feature.
*/
public val type: kotlin.String? = builder.type
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.Limitation = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Limitation(")
append("databaseId=$databaseId,")
append("description=$description,")
append("engineName=$engineName,")
append("impact=$impact,")
append("name=$name,")
append("type=$type")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = databaseId?.hashCode() ?: 0
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (engineName?.hashCode() ?: 0)
result = 31 * result + (impact?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (type?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Limitation
if (databaseId != other.databaseId) return false
if (description != other.description) return false
if (engineName != other.engineName) return false
if (impact != other.impact) return false
if (name != other.name) return false
if (type != other.type) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.Limitation = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The identifier of the source database.
*/
public var databaseId: kotlin.String? = null
/**
* A description of the limitation. Provides additional information about the limitation, and includes recommended actions that you can take to address or avoid this limitation.
*/
public var description: kotlin.String? = null
/**
* The name of the target engine that Fleet Advisor should use in the target engine recommendation. Valid values include `"rds-aurora-mysql"`, `"rds-aurora-postgresql"`, `"rds-mysql"`, `"rds-oracle"`, `"rds-sql-server"`, and `"rds-postgresql"`.
*/
public var engineName: kotlin.String? = null
/**
* The impact of the limitation. You can use this parameter to prioritize limitations that you want to address. Valid values include `"Blocker"`, `"High"`, `"Medium"`, and `"Low"`.
*/
public var impact: kotlin.String? = null
/**
* The name of the limitation. Describes unsupported database features, migration action items, and other limitations.
*/
public var name: kotlin.String? = null
/**
* The type of the limitation, such as action required, upgrade required, and limited feature.
*/
public var type: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.Limitation) : this() {
this.databaseId = x.databaseId
this.description = x.description
this.engineName = x.engineName
this.impact = x.impact
this.name = x.name
this.type = x.type
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.Limitation = Limitation(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy