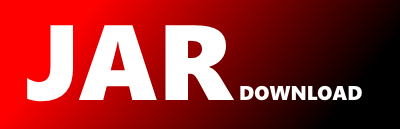
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.ModifyInstanceProfileRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
public class ModifyInstanceProfileRequest private constructor(builder: Builder) {
/**
* The Availability Zone where the instance profile runs.
*/
public val availabilityZone: kotlin.String? = builder.availabilityZone
/**
* A user-friendly description for the instance profile.
*/
public val description: kotlin.String? = builder.description
/**
* The identifier of the instance profile. Identifiers must begin with a letter and must contain only ASCII letters, digits, and hyphens. They can't end with a hyphen, or contain two consecutive hyphens.
*/
public val instanceProfileIdentifier: kotlin.String? = builder.instanceProfileIdentifier
/**
* A user-friendly name for the instance profile.
*/
public val instanceProfileName: kotlin.String? = builder.instanceProfileName
/**
* The Amazon Resource Name (ARN) of the KMS key that is used to encrypt the connection parameters for the instance profile.
*
* If you don't specify a value for the `KmsKeyArn` parameter, then DMS uses your default encryption key.
*
* KMS creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services account has a different default encryption key for each Amazon Web Services Region.
*/
public val kmsKeyArn: kotlin.String? = builder.kmsKeyArn
/**
* Specifies the network type for the instance profile. A value of `IPV4` represents an instance profile with IPv4 network type and only supports IPv4 addressing. A value of `IPV6` represents an instance profile with IPv6 network type and only supports IPv6 addressing. A value of `DUAL` represents an instance profile with dual network type that supports IPv4 and IPv6 addressing.
*/
public val networkType: kotlin.String? = builder.networkType
/**
* Specifies the accessibility options for the instance profile. A value of `true` represents an instance profile with a public IP address. A value of `false` represents an instance profile with a private IP address. The default value is `true`.
*/
public val publiclyAccessible: kotlin.Boolean? = builder.publiclyAccessible
/**
* A subnet group to associate with the instance profile.
*/
public val subnetGroupIdentifier: kotlin.String? = builder.subnetGroupIdentifier
/**
* Specifies the VPC security groups to be used with the instance profile. The VPC security group must work with the VPC containing the instance profile.
*/
public val vpcSecurityGroups: List? = builder.vpcSecurityGroups
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.ModifyInstanceProfileRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ModifyInstanceProfileRequest(")
append("availabilityZone=$availabilityZone,")
append("description=$description,")
append("instanceProfileIdentifier=$instanceProfileIdentifier,")
append("instanceProfileName=$instanceProfileName,")
append("kmsKeyArn=$kmsKeyArn,")
append("networkType=$networkType,")
append("publiclyAccessible=$publiclyAccessible,")
append("subnetGroupIdentifier=$subnetGroupIdentifier,")
append("vpcSecurityGroups=$vpcSecurityGroups")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = availabilityZone?.hashCode() ?: 0
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (instanceProfileIdentifier?.hashCode() ?: 0)
result = 31 * result + (instanceProfileName?.hashCode() ?: 0)
result = 31 * result + (kmsKeyArn?.hashCode() ?: 0)
result = 31 * result + (networkType?.hashCode() ?: 0)
result = 31 * result + (publiclyAccessible?.hashCode() ?: 0)
result = 31 * result + (subnetGroupIdentifier?.hashCode() ?: 0)
result = 31 * result + (vpcSecurityGroups?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ModifyInstanceProfileRequest
if (availabilityZone != other.availabilityZone) return false
if (description != other.description) return false
if (instanceProfileIdentifier != other.instanceProfileIdentifier) return false
if (instanceProfileName != other.instanceProfileName) return false
if (kmsKeyArn != other.kmsKeyArn) return false
if (networkType != other.networkType) return false
if (publiclyAccessible != other.publiclyAccessible) return false
if (subnetGroupIdentifier != other.subnetGroupIdentifier) return false
if (vpcSecurityGroups != other.vpcSecurityGroups) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.ModifyInstanceProfileRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The Availability Zone where the instance profile runs.
*/
public var availabilityZone: kotlin.String? = null
/**
* A user-friendly description for the instance profile.
*/
public var description: kotlin.String? = null
/**
* The identifier of the instance profile. Identifiers must begin with a letter and must contain only ASCII letters, digits, and hyphens. They can't end with a hyphen, or contain two consecutive hyphens.
*/
public var instanceProfileIdentifier: kotlin.String? = null
/**
* A user-friendly name for the instance profile.
*/
public var instanceProfileName: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the KMS key that is used to encrypt the connection parameters for the instance profile.
*
* If you don't specify a value for the `KmsKeyArn` parameter, then DMS uses your default encryption key.
*
* KMS creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services account has a different default encryption key for each Amazon Web Services Region.
*/
public var kmsKeyArn: kotlin.String? = null
/**
* Specifies the network type for the instance profile. A value of `IPV4` represents an instance profile with IPv4 network type and only supports IPv4 addressing. A value of `IPV6` represents an instance profile with IPv6 network type and only supports IPv6 addressing. A value of `DUAL` represents an instance profile with dual network type that supports IPv4 and IPv6 addressing.
*/
public var networkType: kotlin.String? = null
/**
* Specifies the accessibility options for the instance profile. A value of `true` represents an instance profile with a public IP address. A value of `false` represents an instance profile with a private IP address. The default value is `true`.
*/
public var publiclyAccessible: kotlin.Boolean? = null
/**
* A subnet group to associate with the instance profile.
*/
public var subnetGroupIdentifier: kotlin.String? = null
/**
* Specifies the VPC security groups to be used with the instance profile. The VPC security group must work with the VPC containing the instance profile.
*/
public var vpcSecurityGroups: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.ModifyInstanceProfileRequest) : this() {
this.availabilityZone = x.availabilityZone
this.description = x.description
this.instanceProfileIdentifier = x.instanceProfileIdentifier
this.instanceProfileName = x.instanceProfileName
this.kmsKeyArn = x.kmsKeyArn
this.networkType = x.networkType
this.publiclyAccessible = x.publiclyAccessible
this.subnetGroupIdentifier = x.subnetGroupIdentifier
this.vpcSecurityGroups = x.vpcSecurityGroups
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.ModifyInstanceProfileRequest = ModifyInstanceProfileRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy