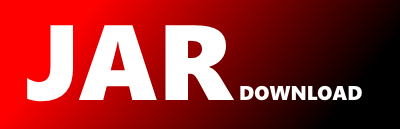
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.ModifyReplicationInstanceRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
*
*/
public class ModifyReplicationInstanceRequest private constructor(builder: Builder) {
/**
* The amount of storage (in gigabytes) to be allocated for the replication instance.
*/
public val allocatedStorage: kotlin.Int? = builder.allocatedStorage
/**
* Indicates that major version upgrades are allowed. Changing this parameter does not result in an outage, and the change is asynchronously applied as soon as possible.
*
* This parameter must be set to `true` when specifying a value for the `EngineVersion` parameter that is a different major version than the replication instance's current version.
*/
public val allowMajorVersionUpgrade: kotlin.Boolean? = builder.allowMajorVersionUpgrade
/**
* Indicates whether the changes should be applied immediately or during the next maintenance window.
*/
public val applyImmediately: kotlin.Boolean? = builder.applyImmediately
/**
* A value that indicates that minor version upgrades are applied automatically to the replication instance during the maintenance window. Changing this parameter doesn't result in an outage, except in the case described following. The change is asynchronously applied as soon as possible.
*
* An outage does result if these factors apply:
* + This parameter is set to `true` during the maintenance window.
* + A newer minor version is available.
* + DMS has enabled automatic patching for the given engine version.
*/
public val autoMinorVersionUpgrade: kotlin.Boolean? = builder.autoMinorVersionUpgrade
/**
* The engine version number of the replication instance.
*
* When modifying a major engine version of an instance, also set `AllowMajorVersionUpgrade` to `true`.
*/
public val engineVersion: kotlin.String? = builder.engineVersion
/**
* Specifies the ID of the secret that stores the key cache file required for kerberos authentication, when modifying a replication instance.
*/
public val kerberosAuthenticationSettings: aws.sdk.kotlin.services.databasemigrationservice.model.KerberosAuthenticationSettings? = builder.kerberosAuthenticationSettings
/**
* Specifies whether the replication instance is a Multi-AZ deployment. You can't set the `AvailabilityZone` parameter if the Multi-AZ parameter is set to `true`.
*/
public val multiAz: kotlin.Boolean? = builder.multiAz
/**
* The type of IP address protocol used by a replication instance, such as IPv4 only or Dual-stack that supports both IPv4 and IPv6 addressing. IPv6 only is not yet supported.
*/
public val networkType: kotlin.String? = builder.networkType
/**
* The weekly time range (in UTC) during which system maintenance can occur, which might result in an outage. Changing this parameter does not result in an outage, except in the following situation, and the change is asynchronously applied as soon as possible. If moving this window to the current time, there must be at least 30 minutes between the current time and end of the window to ensure pending changes are applied.
*
* Default: Uses existing setting
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
* Valid Days: Mon | Tue | Wed | Thu | Fri | Sat | Sun
*
* Constraints: Must be at least 30 minutes
*/
public val preferredMaintenanceWindow: kotlin.String? = builder.preferredMaintenanceWindow
/**
* The Amazon Resource Name (ARN) of the replication instance.
*/
public val replicationInstanceArn: kotlin.String? = builder.replicationInstanceArn
/**
* The compute and memory capacity of the replication instance as defined for the specified replication instance class. For example to specify the instance class dms.c4.large, set this parameter to `"dms.c4.large"`.
*
* For more information on the settings and capacities for the available replication instance classes, see [ Selecting the right DMS replication instance for your migration](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_ReplicationInstance.html#CHAP_ReplicationInstance.InDepth).
*/
public val replicationInstanceClass: kotlin.String? = builder.replicationInstanceClass
/**
* The replication instance identifier. This parameter is stored as a lowercase string.
*/
public val replicationInstanceIdentifier: kotlin.String? = builder.replicationInstanceIdentifier
/**
* Specifies the VPC security group to be used with the replication instance. The VPC security group must work with the VPC containing the replication instance.
*/
public val vpcSecurityGroupIds: List? = builder.vpcSecurityGroupIds
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.ModifyReplicationInstanceRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ModifyReplicationInstanceRequest(")
append("allocatedStorage=$allocatedStorage,")
append("allowMajorVersionUpgrade=$allowMajorVersionUpgrade,")
append("applyImmediately=$applyImmediately,")
append("autoMinorVersionUpgrade=$autoMinorVersionUpgrade,")
append("engineVersion=$engineVersion,")
append("kerberosAuthenticationSettings=$kerberosAuthenticationSettings,")
append("multiAz=$multiAz,")
append("networkType=$networkType,")
append("preferredMaintenanceWindow=$preferredMaintenanceWindow,")
append("replicationInstanceArn=$replicationInstanceArn,")
append("replicationInstanceClass=$replicationInstanceClass,")
append("replicationInstanceIdentifier=$replicationInstanceIdentifier,")
append("vpcSecurityGroupIds=$vpcSecurityGroupIds")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = allocatedStorage ?: 0
result = 31 * result + (allowMajorVersionUpgrade?.hashCode() ?: 0)
result = 31 * result + (applyImmediately?.hashCode() ?: 0)
result = 31 * result + (autoMinorVersionUpgrade?.hashCode() ?: 0)
result = 31 * result + (engineVersion?.hashCode() ?: 0)
result = 31 * result + (kerberosAuthenticationSettings?.hashCode() ?: 0)
result = 31 * result + (multiAz?.hashCode() ?: 0)
result = 31 * result + (networkType?.hashCode() ?: 0)
result = 31 * result + (preferredMaintenanceWindow?.hashCode() ?: 0)
result = 31 * result + (replicationInstanceArn?.hashCode() ?: 0)
result = 31 * result + (replicationInstanceClass?.hashCode() ?: 0)
result = 31 * result + (replicationInstanceIdentifier?.hashCode() ?: 0)
result = 31 * result + (vpcSecurityGroupIds?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ModifyReplicationInstanceRequest
if (allocatedStorage != other.allocatedStorage) return false
if (allowMajorVersionUpgrade != other.allowMajorVersionUpgrade) return false
if (applyImmediately != other.applyImmediately) return false
if (autoMinorVersionUpgrade != other.autoMinorVersionUpgrade) return false
if (engineVersion != other.engineVersion) return false
if (kerberosAuthenticationSettings != other.kerberosAuthenticationSettings) return false
if (multiAz != other.multiAz) return false
if (networkType != other.networkType) return false
if (preferredMaintenanceWindow != other.preferredMaintenanceWindow) return false
if (replicationInstanceArn != other.replicationInstanceArn) return false
if (replicationInstanceClass != other.replicationInstanceClass) return false
if (replicationInstanceIdentifier != other.replicationInstanceIdentifier) return false
if (vpcSecurityGroupIds != other.vpcSecurityGroupIds) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.ModifyReplicationInstanceRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The amount of storage (in gigabytes) to be allocated for the replication instance.
*/
public var allocatedStorage: kotlin.Int? = null
/**
* Indicates that major version upgrades are allowed. Changing this parameter does not result in an outage, and the change is asynchronously applied as soon as possible.
*
* This parameter must be set to `true` when specifying a value for the `EngineVersion` parameter that is a different major version than the replication instance's current version.
*/
public var allowMajorVersionUpgrade: kotlin.Boolean? = null
/**
* Indicates whether the changes should be applied immediately or during the next maintenance window.
*/
public var applyImmediately: kotlin.Boolean? = null
/**
* A value that indicates that minor version upgrades are applied automatically to the replication instance during the maintenance window. Changing this parameter doesn't result in an outage, except in the case described following. The change is asynchronously applied as soon as possible.
*
* An outage does result if these factors apply:
* + This parameter is set to `true` during the maintenance window.
* + A newer minor version is available.
* + DMS has enabled automatic patching for the given engine version.
*/
public var autoMinorVersionUpgrade: kotlin.Boolean? = null
/**
* The engine version number of the replication instance.
*
* When modifying a major engine version of an instance, also set `AllowMajorVersionUpgrade` to `true`.
*/
public var engineVersion: kotlin.String? = null
/**
* Specifies the ID of the secret that stores the key cache file required for kerberos authentication, when modifying a replication instance.
*/
public var kerberosAuthenticationSettings: aws.sdk.kotlin.services.databasemigrationservice.model.KerberosAuthenticationSettings? = null
/**
* Specifies whether the replication instance is a Multi-AZ deployment. You can't set the `AvailabilityZone` parameter if the Multi-AZ parameter is set to `true`.
*/
public var multiAz: kotlin.Boolean? = null
/**
* The type of IP address protocol used by a replication instance, such as IPv4 only or Dual-stack that supports both IPv4 and IPv6 addressing. IPv6 only is not yet supported.
*/
public var networkType: kotlin.String? = null
/**
* The weekly time range (in UTC) during which system maintenance can occur, which might result in an outage. Changing this parameter does not result in an outage, except in the following situation, and the change is asynchronously applied as soon as possible. If moving this window to the current time, there must be at least 30 minutes between the current time and end of the window to ensure pending changes are applied.
*
* Default: Uses existing setting
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
* Valid Days: Mon | Tue | Wed | Thu | Fri | Sat | Sun
*
* Constraints: Must be at least 30 minutes
*/
public var preferredMaintenanceWindow: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the replication instance.
*/
public var replicationInstanceArn: kotlin.String? = null
/**
* The compute and memory capacity of the replication instance as defined for the specified replication instance class. For example to specify the instance class dms.c4.large, set this parameter to `"dms.c4.large"`.
*
* For more information on the settings and capacities for the available replication instance classes, see [ Selecting the right DMS replication instance for your migration](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_ReplicationInstance.html#CHAP_ReplicationInstance.InDepth).
*/
public var replicationInstanceClass: kotlin.String? = null
/**
* The replication instance identifier. This parameter is stored as a lowercase string.
*/
public var replicationInstanceIdentifier: kotlin.String? = null
/**
* Specifies the VPC security group to be used with the replication instance. The VPC security group must work with the VPC containing the replication instance.
*/
public var vpcSecurityGroupIds: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.ModifyReplicationInstanceRequest) : this() {
this.allocatedStorage = x.allocatedStorage
this.allowMajorVersionUpgrade = x.allowMajorVersionUpgrade
this.applyImmediately = x.applyImmediately
this.autoMinorVersionUpgrade = x.autoMinorVersionUpgrade
this.engineVersion = x.engineVersion
this.kerberosAuthenticationSettings = x.kerberosAuthenticationSettings
this.multiAz = x.multiAz
this.networkType = x.networkType
this.preferredMaintenanceWindow = x.preferredMaintenanceWindow
this.replicationInstanceArn = x.replicationInstanceArn
this.replicationInstanceClass = x.replicationInstanceClass
this.replicationInstanceIdentifier = x.replicationInstanceIdentifier
this.vpcSecurityGroupIds = x.vpcSecurityGroupIds
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.ModifyReplicationInstanceRequest = ModifyReplicationInstanceRequest(this)
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.KerberosAuthenticationSettings] inside the given [block]
*/
public fun kerberosAuthenticationSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.KerberosAuthenticationSettings.Builder.() -> kotlin.Unit) {
this.kerberosAuthenticationSettings = aws.sdk.kotlin.services.databasemigrationservice.model.KerberosAuthenticationSettings.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy