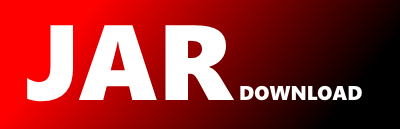
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.NeptuneSettings.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Provides information that defines an Amazon Neptune endpoint.
*/
public class NeptuneSettings private constructor(builder: Builder) {
/**
* The number of milliseconds for DMS to wait to retry a bulk-load of migrated graph data to the Neptune target database before raising an error. The default is 250.
*/
public val errorRetryDuration: kotlin.Int? = builder.errorRetryDuration
/**
* If you want Identity and Access Management (IAM) authorization enabled for this endpoint, set this parameter to `true`. Then attach the appropriate IAM policy document to your service role specified by `ServiceAccessRoleArn`. The default is `false`.
*/
public val iamAuthEnabled: kotlin.Boolean? = builder.iamAuthEnabled
/**
* The maximum size in kilobytes of migrated graph data stored in a .csv file before DMS bulk-loads the data to the Neptune target database. The default is 1,048,576 KB. If the bulk load is successful, DMS clears the bucket, ready to store the next batch of migrated graph data.
*/
public val maxFileSize: kotlin.Int? = builder.maxFileSize
/**
* The number of times for DMS to retry a bulk load of migrated graph data to the Neptune target database before raising an error. The default is 5.
*/
public val maxRetryCount: kotlin.Int? = builder.maxRetryCount
/**
* A folder path where you want DMS to store migrated graph data in the S3 bucket specified by `S3BucketName`
*/
public val s3BucketFolder: kotlin.String = requireNotNull(builder.s3BucketFolder) { "A non-null value must be provided for s3BucketFolder" }
/**
* The name of the Amazon S3 bucket where DMS can temporarily store migrated graph data in .csv files before bulk-loading it to the Neptune target database. DMS maps the SQL source data to graph data before storing it in these .csv files.
*/
public val s3BucketName: kotlin.String = requireNotNull(builder.s3BucketName) { "A non-null value must be provided for s3BucketName" }
/**
* The Amazon Resource Name (ARN) of the service role that you created for the Neptune target endpoint. The role must allow the `iam:PassRole` action. For more information, see [Creating an IAM Service Role for Accessing Amazon Neptune as a Target](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.Neptune.html#CHAP_Target.Neptune.ServiceRole) in the *Database Migration Service User Guide. *
*/
public val serviceAccessRoleArn: kotlin.String? = builder.serviceAccessRoleArn
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.NeptuneSettings = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("NeptuneSettings(")
append("errorRetryDuration=$errorRetryDuration,")
append("iamAuthEnabled=$iamAuthEnabled,")
append("maxFileSize=$maxFileSize,")
append("maxRetryCount=$maxRetryCount,")
append("s3BucketFolder=$s3BucketFolder,")
append("s3BucketName=$s3BucketName,")
append("serviceAccessRoleArn=$serviceAccessRoleArn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = errorRetryDuration ?: 0
result = 31 * result + (iamAuthEnabled?.hashCode() ?: 0)
result = 31 * result + (maxFileSize ?: 0)
result = 31 * result + (maxRetryCount ?: 0)
result = 31 * result + (s3BucketFolder.hashCode())
result = 31 * result + (s3BucketName.hashCode())
result = 31 * result + (serviceAccessRoleArn?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as NeptuneSettings
if (errorRetryDuration != other.errorRetryDuration) return false
if (iamAuthEnabled != other.iamAuthEnabled) return false
if (maxFileSize != other.maxFileSize) return false
if (maxRetryCount != other.maxRetryCount) return false
if (s3BucketFolder != other.s3BucketFolder) return false
if (s3BucketName != other.s3BucketName) return false
if (serviceAccessRoleArn != other.serviceAccessRoleArn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.NeptuneSettings = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The number of milliseconds for DMS to wait to retry a bulk-load of migrated graph data to the Neptune target database before raising an error. The default is 250.
*/
public var errorRetryDuration: kotlin.Int? = null
/**
* If you want Identity and Access Management (IAM) authorization enabled for this endpoint, set this parameter to `true`. Then attach the appropriate IAM policy document to your service role specified by `ServiceAccessRoleArn`. The default is `false`.
*/
public var iamAuthEnabled: kotlin.Boolean? = null
/**
* The maximum size in kilobytes of migrated graph data stored in a .csv file before DMS bulk-loads the data to the Neptune target database. The default is 1,048,576 KB. If the bulk load is successful, DMS clears the bucket, ready to store the next batch of migrated graph data.
*/
public var maxFileSize: kotlin.Int? = null
/**
* The number of times for DMS to retry a bulk load of migrated graph data to the Neptune target database before raising an error. The default is 5.
*/
public var maxRetryCount: kotlin.Int? = null
/**
* A folder path where you want DMS to store migrated graph data in the S3 bucket specified by `S3BucketName`
*/
public var s3BucketFolder: kotlin.String? = null
/**
* The name of the Amazon S3 bucket where DMS can temporarily store migrated graph data in .csv files before bulk-loading it to the Neptune target database. DMS maps the SQL source data to graph data before storing it in these .csv files.
*/
public var s3BucketName: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the service role that you created for the Neptune target endpoint. The role must allow the `iam:PassRole` action. For more information, see [Creating an IAM Service Role for Accessing Amazon Neptune as a Target](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.Neptune.html#CHAP_Target.Neptune.ServiceRole) in the *Database Migration Service User Guide. *
*/
public var serviceAccessRoleArn: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.NeptuneSettings) : this() {
this.errorRetryDuration = x.errorRetryDuration
this.iamAuthEnabled = x.iamAuthEnabled
this.maxFileSize = x.maxFileSize
this.maxRetryCount = x.maxRetryCount
this.s3BucketFolder = x.s3BucketFolder
this.s3BucketName = x.s3BucketName
this.serviceAccessRoleArn = x.serviceAccessRoleArn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.NeptuneSettings = NeptuneSettings(this)
internal fun correctErrors(): Builder {
if (s3BucketFolder == null) s3BucketFolder = ""
if (s3BucketName == null) s3BucketName = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy