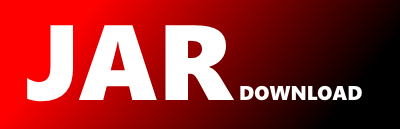
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.OracleDataProviderSettings.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Provides information that defines an Oracle data provider.
*/
public class OracleDataProviderSettings private constructor(builder: Builder) {
/**
* The address of your Oracle Automatic Storage Management (ASM) server. You can set this value from the `asm_server` value. You set `asm_server` as part of the extra connection attribute string to access an Oracle server with Binary Reader that uses ASM. For more information, see [Configuration for change data capture (CDC) on an Oracle source database](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Source.Oracle.html#dms/latest/userguide/CHAP_Source.Oracle.html#CHAP_Source.Oracle.CDC.Configuration).
*/
public val asmServer: kotlin.String? = builder.asmServer
/**
* The Amazon Resource Name (ARN) of the certificate used for SSL connection.
*/
public val certificateArn: kotlin.String? = builder.certificateArn
/**
* The database name on the Oracle data provider.
*/
public val databaseName: kotlin.String? = builder.databaseName
/**
* The port value for the Oracle data provider.
*/
public val port: kotlin.Int? = builder.port
/**
* The ARN of the IAM role that provides access to the secret in Secrets Manager that contains the Oracle ASM connection details.
*/
public val secretsManagerOracleAsmAccessRoleArn: kotlin.String? = builder.secretsManagerOracleAsmAccessRoleArn
/**
* The identifier of the secret in Secrets Manager that contains the Oracle ASM connection details.
*
* Required only if your data provider uses the Oracle ASM server.
*/
public val secretsManagerOracleAsmSecretId: kotlin.String? = builder.secretsManagerOracleAsmSecretId
/**
* The ARN of the IAM role that provides access to the secret in Secrets Manager that contains the TDE password.
*/
public val secretsManagerSecurityDbEncryptionAccessRoleArn: kotlin.String? = builder.secretsManagerSecurityDbEncryptionAccessRoleArn
/**
* The identifier of the secret in Secrets Manager that contains the transparent data encryption (TDE) password. DMS requires this password to access Oracle redo logs encrypted by TDE using Binary Reader.
*/
public val secretsManagerSecurityDbEncryptionSecretId: kotlin.String? = builder.secretsManagerSecurityDbEncryptionSecretId
/**
* The name of the Oracle server.
*/
public val serverName: kotlin.String? = builder.serverName
/**
* The SSL mode used to connect to the Oracle data provider. The default value is `none`.
*/
public val sslMode: aws.sdk.kotlin.services.databasemigrationservice.model.DmsSslModeValue? = builder.sslMode
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.OracleDataProviderSettings = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("OracleDataProviderSettings(")
append("asmServer=$asmServer,")
append("certificateArn=$certificateArn,")
append("databaseName=$databaseName,")
append("port=$port,")
append("secretsManagerOracleAsmAccessRoleArn=$secretsManagerOracleAsmAccessRoleArn,")
append("secretsManagerOracleAsmSecretId=$secretsManagerOracleAsmSecretId,")
append("secretsManagerSecurityDbEncryptionAccessRoleArn=$secretsManagerSecurityDbEncryptionAccessRoleArn,")
append("secretsManagerSecurityDbEncryptionSecretId=$secretsManagerSecurityDbEncryptionSecretId,")
append("serverName=$serverName,")
append("sslMode=$sslMode")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = asmServer?.hashCode() ?: 0
result = 31 * result + (certificateArn?.hashCode() ?: 0)
result = 31 * result + (databaseName?.hashCode() ?: 0)
result = 31 * result + (port ?: 0)
result = 31 * result + (secretsManagerOracleAsmAccessRoleArn?.hashCode() ?: 0)
result = 31 * result + (secretsManagerOracleAsmSecretId?.hashCode() ?: 0)
result = 31 * result + (secretsManagerSecurityDbEncryptionAccessRoleArn?.hashCode() ?: 0)
result = 31 * result + (secretsManagerSecurityDbEncryptionSecretId?.hashCode() ?: 0)
result = 31 * result + (serverName?.hashCode() ?: 0)
result = 31 * result + (sslMode?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as OracleDataProviderSettings
if (asmServer != other.asmServer) return false
if (certificateArn != other.certificateArn) return false
if (databaseName != other.databaseName) return false
if (port != other.port) return false
if (secretsManagerOracleAsmAccessRoleArn != other.secretsManagerOracleAsmAccessRoleArn) return false
if (secretsManagerOracleAsmSecretId != other.secretsManagerOracleAsmSecretId) return false
if (secretsManagerSecurityDbEncryptionAccessRoleArn != other.secretsManagerSecurityDbEncryptionAccessRoleArn) return false
if (secretsManagerSecurityDbEncryptionSecretId != other.secretsManagerSecurityDbEncryptionSecretId) return false
if (serverName != other.serverName) return false
if (sslMode != other.sslMode) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.OracleDataProviderSettings = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The address of your Oracle Automatic Storage Management (ASM) server. You can set this value from the `asm_server` value. You set `asm_server` as part of the extra connection attribute string to access an Oracle server with Binary Reader that uses ASM. For more information, see [Configuration for change data capture (CDC) on an Oracle source database](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Source.Oracle.html#dms/latest/userguide/CHAP_Source.Oracle.html#CHAP_Source.Oracle.CDC.Configuration).
*/
public var asmServer: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the certificate used for SSL connection.
*/
public var certificateArn: kotlin.String? = null
/**
* The database name on the Oracle data provider.
*/
public var databaseName: kotlin.String? = null
/**
* The port value for the Oracle data provider.
*/
public var port: kotlin.Int? = null
/**
* The ARN of the IAM role that provides access to the secret in Secrets Manager that contains the Oracle ASM connection details.
*/
public var secretsManagerOracleAsmAccessRoleArn: kotlin.String? = null
/**
* The identifier of the secret in Secrets Manager that contains the Oracle ASM connection details.
*
* Required only if your data provider uses the Oracle ASM server.
*/
public var secretsManagerOracleAsmSecretId: kotlin.String? = null
/**
* The ARN of the IAM role that provides access to the secret in Secrets Manager that contains the TDE password.
*/
public var secretsManagerSecurityDbEncryptionAccessRoleArn: kotlin.String? = null
/**
* The identifier of the secret in Secrets Manager that contains the transparent data encryption (TDE) password. DMS requires this password to access Oracle redo logs encrypted by TDE using Binary Reader.
*/
public var secretsManagerSecurityDbEncryptionSecretId: kotlin.String? = null
/**
* The name of the Oracle server.
*/
public var serverName: kotlin.String? = null
/**
* The SSL mode used to connect to the Oracle data provider. The default value is `none`.
*/
public var sslMode: aws.sdk.kotlin.services.databasemigrationservice.model.DmsSslModeValue? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.OracleDataProviderSettings) : this() {
this.asmServer = x.asmServer
this.certificateArn = x.certificateArn
this.databaseName = x.databaseName
this.port = x.port
this.secretsManagerOracleAsmAccessRoleArn = x.secretsManagerOracleAsmAccessRoleArn
this.secretsManagerOracleAsmSecretId = x.secretsManagerOracleAsmSecretId
this.secretsManagerSecurityDbEncryptionAccessRoleArn = x.secretsManagerSecurityDbEncryptionAccessRoleArn
this.secretsManagerSecurityDbEncryptionSecretId = x.secretsManagerSecurityDbEncryptionSecretId
this.serverName = x.serverName
this.sslMode = x.sslMode
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.OracleDataProviderSettings = OracleDataProviderSettings(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy