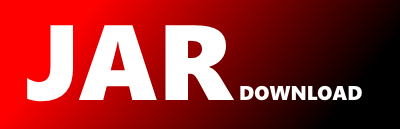
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.OrderableReplicationInstance.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* In response to the `DescribeOrderableReplicationInstances` operation, this object describes an available replication instance. This description includes the replication instance's type, engine version, and allocated storage.
*/
public class OrderableReplicationInstance private constructor(builder: Builder) {
/**
* List of Availability Zones for this replication instance.
*/
public val availabilityZones: List? = builder.availabilityZones
/**
* The default amount of storage (in gigabytes) that is allocated for the replication instance.
*/
public val defaultAllocatedStorage: kotlin.Int = builder.defaultAllocatedStorage
/**
* The version of the replication engine.
*/
public val engineVersion: kotlin.String? = builder.engineVersion
/**
* The amount of storage (in gigabytes) that is allocated for the replication instance.
*/
public val includedAllocatedStorage: kotlin.Int = builder.includedAllocatedStorage
/**
* The minimum amount of storage (in gigabytes) that can be allocated for the replication instance.
*/
public val maxAllocatedStorage: kotlin.Int = builder.maxAllocatedStorage
/**
* The minimum amount of storage (in gigabytes) that can be allocated for the replication instance.
*/
public val minAllocatedStorage: kotlin.Int = builder.minAllocatedStorage
/**
* The value returned when the specified `EngineVersion` of the replication instance is in Beta or test mode. This indicates some features might not work as expected.
*
* DMS supports the `ReleaseStatus` parameter in versions 3.1.4 and later.
*/
public val releaseStatus: aws.sdk.kotlin.services.databasemigrationservice.model.ReleaseStatusValues? = builder.releaseStatus
/**
* The compute and memory capacity of the replication instance as defined for the specified replication instance class. For example to specify the instance class dms.c4.large, set this parameter to `"dms.c4.large"`.
*
* For more information on the settings and capacities for the available replication instance classes, see [ Selecting the right DMS replication instance for your migration](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_ReplicationInstance.html#CHAP_ReplicationInstance.InDepth).
*/
public val replicationInstanceClass: kotlin.String? = builder.replicationInstanceClass
/**
* The type of storage used by the replication instance.
*/
public val storageType: kotlin.String? = builder.storageType
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.OrderableReplicationInstance = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("OrderableReplicationInstance(")
append("availabilityZones=$availabilityZones,")
append("defaultAllocatedStorage=$defaultAllocatedStorage,")
append("engineVersion=$engineVersion,")
append("includedAllocatedStorage=$includedAllocatedStorage,")
append("maxAllocatedStorage=$maxAllocatedStorage,")
append("minAllocatedStorage=$minAllocatedStorage,")
append("releaseStatus=$releaseStatus,")
append("replicationInstanceClass=$replicationInstanceClass,")
append("storageType=$storageType")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = availabilityZones?.hashCode() ?: 0
result = 31 * result + (defaultAllocatedStorage)
result = 31 * result + (engineVersion?.hashCode() ?: 0)
result = 31 * result + (includedAllocatedStorage)
result = 31 * result + (maxAllocatedStorage)
result = 31 * result + (minAllocatedStorage)
result = 31 * result + (releaseStatus?.hashCode() ?: 0)
result = 31 * result + (replicationInstanceClass?.hashCode() ?: 0)
result = 31 * result + (storageType?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as OrderableReplicationInstance
if (availabilityZones != other.availabilityZones) return false
if (defaultAllocatedStorage != other.defaultAllocatedStorage) return false
if (engineVersion != other.engineVersion) return false
if (includedAllocatedStorage != other.includedAllocatedStorage) return false
if (maxAllocatedStorage != other.maxAllocatedStorage) return false
if (minAllocatedStorage != other.minAllocatedStorage) return false
if (releaseStatus != other.releaseStatus) return false
if (replicationInstanceClass != other.replicationInstanceClass) return false
if (storageType != other.storageType) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.OrderableReplicationInstance = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* List of Availability Zones for this replication instance.
*/
public var availabilityZones: List? = null
/**
* The default amount of storage (in gigabytes) that is allocated for the replication instance.
*/
public var defaultAllocatedStorage: kotlin.Int = 0
/**
* The version of the replication engine.
*/
public var engineVersion: kotlin.String? = null
/**
* The amount of storage (in gigabytes) that is allocated for the replication instance.
*/
public var includedAllocatedStorage: kotlin.Int = 0
/**
* The minimum amount of storage (in gigabytes) that can be allocated for the replication instance.
*/
public var maxAllocatedStorage: kotlin.Int = 0
/**
* The minimum amount of storage (in gigabytes) that can be allocated for the replication instance.
*/
public var minAllocatedStorage: kotlin.Int = 0
/**
* The value returned when the specified `EngineVersion` of the replication instance is in Beta or test mode. This indicates some features might not work as expected.
*
* DMS supports the `ReleaseStatus` parameter in versions 3.1.4 and later.
*/
public var releaseStatus: aws.sdk.kotlin.services.databasemigrationservice.model.ReleaseStatusValues? = null
/**
* The compute and memory capacity of the replication instance as defined for the specified replication instance class. For example to specify the instance class dms.c4.large, set this parameter to `"dms.c4.large"`.
*
* For more information on the settings and capacities for the available replication instance classes, see [ Selecting the right DMS replication instance for your migration](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_ReplicationInstance.html#CHAP_ReplicationInstance.InDepth).
*/
public var replicationInstanceClass: kotlin.String? = null
/**
* The type of storage used by the replication instance.
*/
public var storageType: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.OrderableReplicationInstance) : this() {
this.availabilityZones = x.availabilityZones
this.defaultAllocatedStorage = x.defaultAllocatedStorage
this.engineVersion = x.engineVersion
this.includedAllocatedStorage = x.includedAllocatedStorage
this.maxAllocatedStorage = x.maxAllocatedStorage
this.minAllocatedStorage = x.minAllocatedStorage
this.releaseStatus = x.releaseStatus
this.replicationInstanceClass = x.replicationInstanceClass
this.storageType = x.storageType
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.OrderableReplicationInstance = OrderableReplicationInstance(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy