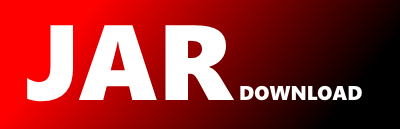
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.PostgreSqlSettings.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Provides information that defines a PostgreSQL endpoint.
*/
public class PostgreSqlSettings private constructor(builder: Builder) {
/**
* For use with change data capture (CDC) only, this attribute has DMS bypass foreign keys and user triggers to reduce the time it takes to bulk load data.
*
* Example: `afterConnectScript=SET session_replication_role='replica'`
*/
public val afterConnectScript: kotlin.String? = builder.afterConnectScript
/**
* The Babelfish for Aurora PostgreSQL database name for the endpoint.
*/
public val babelfishDatabaseName: kotlin.String? = builder.babelfishDatabaseName
/**
* To capture DDL events, DMS creates various artifacts in the PostgreSQL database when the task starts. You can later remove these artifacts.
*
* The default value is `true`.
*
* If this value is set to `N`, you don't have to create tables or triggers on the source database.
*/
public val captureDdls: kotlin.Boolean? = builder.captureDdls
/**
* Specifies the default behavior of the replication's handling of PostgreSQL- compatible endpoints that require some additional configuration, such as Babelfish endpoints.
*/
public val databaseMode: aws.sdk.kotlin.services.databasemigrationservice.model.DatabaseMode? = builder.databaseMode
/**
* Database name for the endpoint.
*/
public val databaseName: kotlin.String? = builder.databaseName
/**
* The schema in which the operational DDL database artifacts are created.
*
* The default value is `public`.
*
* Example: `ddlArtifactsSchema=xyzddlschema;`
*/
public val ddlArtifactsSchema: kotlin.String? = builder.ddlArtifactsSchema
/**
* Disables the Unicode source filter with PostgreSQL, for values passed into the Selection rule filter on Source Endpoint column values. By default DMS performs source filter comparisons using a Unicode string which can cause look ups to ignore the indexes in the text columns and slow down migrations.
*
* Unicode support should only be disabled when using a selection rule filter is on a text column in the Source database that is indexed.
*/
public val disableUnicodeSourceFilter: kotlin.Boolean? = builder.disableUnicodeSourceFilter
/**
* Sets the client statement timeout for the PostgreSQL instance, in seconds. The default value is 60 seconds.
*
* Example: `executeTimeout=100;`
*/
public val executeTimeout: kotlin.Int? = builder.executeTimeout
/**
* When set to `true`, this value causes a task to fail if the actual size of a LOB column is greater than the specified `LobMaxSize`.
*
* The default value is `false`.
*
* If task is set to Limited LOB mode and this option is set to true, the task fails instead of truncating the LOB data.
*/
public val failTasksOnLobTruncation: kotlin.Boolean? = builder.failTasksOnLobTruncation
/**
* The write-ahead log (WAL) heartbeat feature mimics a dummy transaction. By doing this, it prevents idle logical replication slots from holding onto old WAL logs, which can result in storage full situations on the source. This heartbeat keeps `restart_lsn` moving and prevents storage full scenarios.
*
* The default value is `false`.
*/
public val heartbeatEnable: kotlin.Boolean? = builder.heartbeatEnable
/**
* Sets the WAL heartbeat frequency (in minutes).
*
* The default value is 5 minutes.
*/
public val heartbeatFrequency: kotlin.Int? = builder.heartbeatFrequency
/**
* Sets the schema in which the heartbeat artifacts are created.
*
* The default value is `public`.
*/
public val heartbeatSchema: kotlin.String? = builder.heartbeatSchema
/**
* When true, lets PostgreSQL migrate the boolean type as boolean. By default, PostgreSQL migrates booleans as `varchar(5)`. You must set this setting on both the source and target endpoints for it to take effect.
*
* The default value is `false`.
*/
public val mapBooleanAsBoolean: kotlin.Boolean? = builder.mapBooleanAsBoolean
/**
* When true, DMS migrates JSONB values as CLOB.
*
* The default value is `false`.
*/
public val mapJsonbAsClob: kotlin.Boolean? = builder.mapJsonbAsClob
/**
* Sets what datatype to map LONG values as.
*
* The default value is `wstring`.
*/
public val mapLongVarcharAs: aws.sdk.kotlin.services.databasemigrationservice.model.LongVarcharMappingType? = builder.mapLongVarcharAs
/**
* Specifies the maximum size (in KB) of any .csv file used to transfer data to PostgreSQL.
*
* The default value is 32,768 KB (32 MB).
*
* Example: `maxFileSize=512`
*/
public val maxFileSize: kotlin.Int? = builder.maxFileSize
/**
* Endpoint connection password.
*/
public val password: kotlin.String? = builder.password
/**
* Specifies the plugin to use to create a replication slot.
*
* The default value is `pglogical`.
*/
public val pluginName: aws.sdk.kotlin.services.databasemigrationservice.model.PluginNameValue? = builder.pluginName
/**
* Endpoint TCP port. The default is 5432.
*/
public val port: kotlin.Int? = builder.port
/**
* The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants the required permissions to access the value in `SecretsManagerSecret`. The role must allow the `iam:PassRole` action. `SecretsManagerSecret` has the value of the Amazon Web Services Secrets Manager secret that allows access to the PostgreSQL endpoint.
*
* You can specify one of two sets of values for these permissions. You can specify the values for this setting and `SecretsManagerSecretId`. Or you can specify clear-text values for `UserName`, `Password`, `ServerName`, and `Port`. You can't specify both. For more information on creating this `SecretsManagerSecret` and the `SecretsManagerAccessRoleArn` and `SecretsManagerSecretId` required to access it, see [Using secrets to access Database Migration Service resources](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Security.html#security-iam-secretsmanager) in the *Database Migration Service User Guide*.
*/
public val secretsManagerAccessRoleArn: kotlin.String? = builder.secretsManagerAccessRoleArn
/**
* The full ARN, partial ARN, or friendly name of the `SecretsManagerSecret` that contains the PostgreSQL endpoint connection details.
*/
public val secretsManagerSecretId: kotlin.String? = builder.secretsManagerSecretId
/**
* The host name of the endpoint database.
*
* For an Amazon RDS PostgreSQL instance, this is the output of [DescribeDBInstances](https://docs.aws.amazon.com/AmazonRDS/latest/APIReference/API_DescribeDBInstances.html), in the ` Endpoint.Address` field.
*
* For an Aurora PostgreSQL instance, this is the output of [DescribeDBClusters](https://docs.aws.amazon.com/AmazonRDS/latest/APIReference/API_DescribeDBClusters.html), in the `Endpoint` field.
*/
public val serverName: kotlin.String? = builder.serverName
/**
* Sets the name of a previously created logical replication slot for a change data capture (CDC) load of the PostgreSQL source instance.
*
* When used with the `CdcStartPosition` request parameter for the DMS API , this attribute also makes it possible to use native CDC start points. DMS verifies that the specified logical replication slot exists before starting the CDC load task. It also verifies that the task was created with a valid setting of `CdcStartPosition`. If the specified slot doesn't exist or the task doesn't have a valid `CdcStartPosition` setting, DMS raises an error.
*
* For more information about setting the `CdcStartPosition` request parameter, see [Determining a CDC native start point](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Task.CDC.html#CHAP_Task.CDC.StartPoint.Native) in the *Database Migration Service User Guide*. For more information about using `CdcStartPosition`, see [CreateReplicationTask](https://docs.aws.amazon.com/dms/latest/APIReference/API_CreateReplicationTask.html), [StartReplicationTask](https://docs.aws.amazon.com/dms/latest/APIReference/API_StartReplicationTask.html), and [ModifyReplicationTask](https://docs.aws.amazon.com/dms/latest/APIReference/API_ModifyReplicationTask.html).
*/
public val slotName: kotlin.String? = builder.slotName
/**
* Use the `TrimSpaceInChar` source endpoint setting to trim data on CHAR and NCHAR data types during migration. The default value is `true`.
*/
public val trimSpaceInChar: kotlin.Boolean? = builder.trimSpaceInChar
/**
* Endpoint connection user name.
*/
public val username: kotlin.String? = builder.username
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.PostgreSqlSettings = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("PostgreSqlSettings(")
append("afterConnectScript=$afterConnectScript,")
append("babelfishDatabaseName=$babelfishDatabaseName,")
append("captureDdls=$captureDdls,")
append("databaseMode=$databaseMode,")
append("databaseName=$databaseName,")
append("ddlArtifactsSchema=$ddlArtifactsSchema,")
append("disableUnicodeSourceFilter=$disableUnicodeSourceFilter,")
append("executeTimeout=$executeTimeout,")
append("failTasksOnLobTruncation=$failTasksOnLobTruncation,")
append("heartbeatEnable=$heartbeatEnable,")
append("heartbeatFrequency=$heartbeatFrequency,")
append("heartbeatSchema=$heartbeatSchema,")
append("mapBooleanAsBoolean=$mapBooleanAsBoolean,")
append("mapJsonbAsClob=$mapJsonbAsClob,")
append("mapLongVarcharAs=$mapLongVarcharAs,")
append("maxFileSize=$maxFileSize,")
append("password=*** Sensitive Data Redacted ***,")
append("pluginName=$pluginName,")
append("port=$port,")
append("secretsManagerAccessRoleArn=$secretsManagerAccessRoleArn,")
append("secretsManagerSecretId=$secretsManagerSecretId,")
append("serverName=$serverName,")
append("slotName=$slotName,")
append("trimSpaceInChar=$trimSpaceInChar,")
append("username=$username")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = afterConnectScript?.hashCode() ?: 0
result = 31 * result + (babelfishDatabaseName?.hashCode() ?: 0)
result = 31 * result + (captureDdls?.hashCode() ?: 0)
result = 31 * result + (databaseMode?.hashCode() ?: 0)
result = 31 * result + (databaseName?.hashCode() ?: 0)
result = 31 * result + (ddlArtifactsSchema?.hashCode() ?: 0)
result = 31 * result + (disableUnicodeSourceFilter?.hashCode() ?: 0)
result = 31 * result + (executeTimeout ?: 0)
result = 31 * result + (failTasksOnLobTruncation?.hashCode() ?: 0)
result = 31 * result + (heartbeatEnable?.hashCode() ?: 0)
result = 31 * result + (heartbeatFrequency ?: 0)
result = 31 * result + (heartbeatSchema?.hashCode() ?: 0)
result = 31 * result + (mapBooleanAsBoolean?.hashCode() ?: 0)
result = 31 * result + (mapJsonbAsClob?.hashCode() ?: 0)
result = 31 * result + (mapLongVarcharAs?.hashCode() ?: 0)
result = 31 * result + (maxFileSize ?: 0)
result = 31 * result + (password?.hashCode() ?: 0)
result = 31 * result + (pluginName?.hashCode() ?: 0)
result = 31 * result + (port ?: 0)
result = 31 * result + (secretsManagerAccessRoleArn?.hashCode() ?: 0)
result = 31 * result + (secretsManagerSecretId?.hashCode() ?: 0)
result = 31 * result + (serverName?.hashCode() ?: 0)
result = 31 * result + (slotName?.hashCode() ?: 0)
result = 31 * result + (trimSpaceInChar?.hashCode() ?: 0)
result = 31 * result + (username?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as PostgreSqlSettings
if (afterConnectScript != other.afterConnectScript) return false
if (babelfishDatabaseName != other.babelfishDatabaseName) return false
if (captureDdls != other.captureDdls) return false
if (databaseMode != other.databaseMode) return false
if (databaseName != other.databaseName) return false
if (ddlArtifactsSchema != other.ddlArtifactsSchema) return false
if (disableUnicodeSourceFilter != other.disableUnicodeSourceFilter) return false
if (executeTimeout != other.executeTimeout) return false
if (failTasksOnLobTruncation != other.failTasksOnLobTruncation) return false
if (heartbeatEnable != other.heartbeatEnable) return false
if (heartbeatFrequency != other.heartbeatFrequency) return false
if (heartbeatSchema != other.heartbeatSchema) return false
if (mapBooleanAsBoolean != other.mapBooleanAsBoolean) return false
if (mapJsonbAsClob != other.mapJsonbAsClob) return false
if (mapLongVarcharAs != other.mapLongVarcharAs) return false
if (maxFileSize != other.maxFileSize) return false
if (password != other.password) return false
if (pluginName != other.pluginName) return false
if (port != other.port) return false
if (secretsManagerAccessRoleArn != other.secretsManagerAccessRoleArn) return false
if (secretsManagerSecretId != other.secretsManagerSecretId) return false
if (serverName != other.serverName) return false
if (slotName != other.slotName) return false
if (trimSpaceInChar != other.trimSpaceInChar) return false
if (username != other.username) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.PostgreSqlSettings = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* For use with change data capture (CDC) only, this attribute has DMS bypass foreign keys and user triggers to reduce the time it takes to bulk load data.
*
* Example: `afterConnectScript=SET session_replication_role='replica'`
*/
public var afterConnectScript: kotlin.String? = null
/**
* The Babelfish for Aurora PostgreSQL database name for the endpoint.
*/
public var babelfishDatabaseName: kotlin.String? = null
/**
* To capture DDL events, DMS creates various artifacts in the PostgreSQL database when the task starts. You can later remove these artifacts.
*
* The default value is `true`.
*
* If this value is set to `N`, you don't have to create tables or triggers on the source database.
*/
public var captureDdls: kotlin.Boolean? = null
/**
* Specifies the default behavior of the replication's handling of PostgreSQL- compatible endpoints that require some additional configuration, such as Babelfish endpoints.
*/
public var databaseMode: aws.sdk.kotlin.services.databasemigrationservice.model.DatabaseMode? = null
/**
* Database name for the endpoint.
*/
public var databaseName: kotlin.String? = null
/**
* The schema in which the operational DDL database artifacts are created.
*
* The default value is `public`.
*
* Example: `ddlArtifactsSchema=xyzddlschema;`
*/
public var ddlArtifactsSchema: kotlin.String? = null
/**
* Disables the Unicode source filter with PostgreSQL, for values passed into the Selection rule filter on Source Endpoint column values. By default DMS performs source filter comparisons using a Unicode string which can cause look ups to ignore the indexes in the text columns and slow down migrations.
*
* Unicode support should only be disabled when using a selection rule filter is on a text column in the Source database that is indexed.
*/
public var disableUnicodeSourceFilter: kotlin.Boolean? = null
/**
* Sets the client statement timeout for the PostgreSQL instance, in seconds. The default value is 60 seconds.
*
* Example: `executeTimeout=100;`
*/
public var executeTimeout: kotlin.Int? = null
/**
* When set to `true`, this value causes a task to fail if the actual size of a LOB column is greater than the specified `LobMaxSize`.
*
* The default value is `false`.
*
* If task is set to Limited LOB mode and this option is set to true, the task fails instead of truncating the LOB data.
*/
public var failTasksOnLobTruncation: kotlin.Boolean? = null
/**
* The write-ahead log (WAL) heartbeat feature mimics a dummy transaction. By doing this, it prevents idle logical replication slots from holding onto old WAL logs, which can result in storage full situations on the source. This heartbeat keeps `restart_lsn` moving and prevents storage full scenarios.
*
* The default value is `false`.
*/
public var heartbeatEnable: kotlin.Boolean? = null
/**
* Sets the WAL heartbeat frequency (in minutes).
*
* The default value is 5 minutes.
*/
public var heartbeatFrequency: kotlin.Int? = null
/**
* Sets the schema in which the heartbeat artifacts are created.
*
* The default value is `public`.
*/
public var heartbeatSchema: kotlin.String? = null
/**
* When true, lets PostgreSQL migrate the boolean type as boolean. By default, PostgreSQL migrates booleans as `varchar(5)`. You must set this setting on both the source and target endpoints for it to take effect.
*
* The default value is `false`.
*/
public var mapBooleanAsBoolean: kotlin.Boolean? = null
/**
* When true, DMS migrates JSONB values as CLOB.
*
* The default value is `false`.
*/
public var mapJsonbAsClob: kotlin.Boolean? = null
/**
* Sets what datatype to map LONG values as.
*
* The default value is `wstring`.
*/
public var mapLongVarcharAs: aws.sdk.kotlin.services.databasemigrationservice.model.LongVarcharMappingType? = null
/**
* Specifies the maximum size (in KB) of any .csv file used to transfer data to PostgreSQL.
*
* The default value is 32,768 KB (32 MB).
*
* Example: `maxFileSize=512`
*/
public var maxFileSize: kotlin.Int? = null
/**
* Endpoint connection password.
*/
public var password: kotlin.String? = null
/**
* Specifies the plugin to use to create a replication slot.
*
* The default value is `pglogical`.
*/
public var pluginName: aws.sdk.kotlin.services.databasemigrationservice.model.PluginNameValue? = null
/**
* Endpoint TCP port. The default is 5432.
*/
public var port: kotlin.Int? = null
/**
* The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants the required permissions to access the value in `SecretsManagerSecret`. The role must allow the `iam:PassRole` action. `SecretsManagerSecret` has the value of the Amazon Web Services Secrets Manager secret that allows access to the PostgreSQL endpoint.
*
* You can specify one of two sets of values for these permissions. You can specify the values for this setting and `SecretsManagerSecretId`. Or you can specify clear-text values for `UserName`, `Password`, `ServerName`, and `Port`. You can't specify both. For more information on creating this `SecretsManagerSecret` and the `SecretsManagerAccessRoleArn` and `SecretsManagerSecretId` required to access it, see [Using secrets to access Database Migration Service resources](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Security.html#security-iam-secretsmanager) in the *Database Migration Service User Guide*.
*/
public var secretsManagerAccessRoleArn: kotlin.String? = null
/**
* The full ARN, partial ARN, or friendly name of the `SecretsManagerSecret` that contains the PostgreSQL endpoint connection details.
*/
public var secretsManagerSecretId: kotlin.String? = null
/**
* The host name of the endpoint database.
*
* For an Amazon RDS PostgreSQL instance, this is the output of [DescribeDBInstances](https://docs.aws.amazon.com/AmazonRDS/latest/APIReference/API_DescribeDBInstances.html), in the ` Endpoint.Address` field.
*
* For an Aurora PostgreSQL instance, this is the output of [DescribeDBClusters](https://docs.aws.amazon.com/AmazonRDS/latest/APIReference/API_DescribeDBClusters.html), in the `Endpoint` field.
*/
public var serverName: kotlin.String? = null
/**
* Sets the name of a previously created logical replication slot for a change data capture (CDC) load of the PostgreSQL source instance.
*
* When used with the `CdcStartPosition` request parameter for the DMS API , this attribute also makes it possible to use native CDC start points. DMS verifies that the specified logical replication slot exists before starting the CDC load task. It also verifies that the task was created with a valid setting of `CdcStartPosition`. If the specified slot doesn't exist or the task doesn't have a valid `CdcStartPosition` setting, DMS raises an error.
*
* For more information about setting the `CdcStartPosition` request parameter, see [Determining a CDC native start point](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Task.CDC.html#CHAP_Task.CDC.StartPoint.Native) in the *Database Migration Service User Guide*. For more information about using `CdcStartPosition`, see [CreateReplicationTask](https://docs.aws.amazon.com/dms/latest/APIReference/API_CreateReplicationTask.html), [StartReplicationTask](https://docs.aws.amazon.com/dms/latest/APIReference/API_StartReplicationTask.html), and [ModifyReplicationTask](https://docs.aws.amazon.com/dms/latest/APIReference/API_ModifyReplicationTask.html).
*/
public var slotName: kotlin.String? = null
/**
* Use the `TrimSpaceInChar` source endpoint setting to trim data on CHAR and NCHAR data types during migration. The default value is `true`.
*/
public var trimSpaceInChar: kotlin.Boolean? = null
/**
* Endpoint connection user name.
*/
public var username: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.PostgreSqlSettings) : this() {
this.afterConnectScript = x.afterConnectScript
this.babelfishDatabaseName = x.babelfishDatabaseName
this.captureDdls = x.captureDdls
this.databaseMode = x.databaseMode
this.databaseName = x.databaseName
this.ddlArtifactsSchema = x.ddlArtifactsSchema
this.disableUnicodeSourceFilter = x.disableUnicodeSourceFilter
this.executeTimeout = x.executeTimeout
this.failTasksOnLobTruncation = x.failTasksOnLobTruncation
this.heartbeatEnable = x.heartbeatEnable
this.heartbeatFrequency = x.heartbeatFrequency
this.heartbeatSchema = x.heartbeatSchema
this.mapBooleanAsBoolean = x.mapBooleanAsBoolean
this.mapJsonbAsClob = x.mapJsonbAsClob
this.mapLongVarcharAs = x.mapLongVarcharAs
this.maxFileSize = x.maxFileSize
this.password = x.password
this.pluginName = x.pluginName
this.port = x.port
this.secretsManagerAccessRoleArn = x.secretsManagerAccessRoleArn
this.secretsManagerSecretId = x.secretsManagerSecretId
this.serverName = x.serverName
this.slotName = x.slotName
this.trimSpaceInChar = x.trimSpaceInChar
this.username = x.username
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.PostgreSqlSettings = PostgreSqlSettings(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy