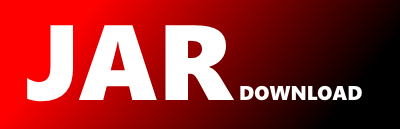
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.Recommendation.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Provides information that describes a recommendation of a target engine.
*
* A *recommendation* is a set of possible Amazon Web Services target engines that you can choose to migrate your source on-premises database. In this set, Fleet Advisor suggests a single target engine as the right sized migration destination. To determine this rightsized migration destination, Fleet Advisor uses the inventory metadata and metrics from data collector. You can use recommendations before the start of migration to save costs and reduce risks.
*
* With recommendations, you can explore different target options and compare metrics, so you can make an informed decision when you choose the migration target.
*/
public class Recommendation private constructor(builder: Builder) {
/**
* The date when Fleet Advisor created the target engine recommendation.
*/
public val createdDate: kotlin.String? = builder.createdDate
/**
* The recommendation of a target engine for the specified source database.
*/
public val data: aws.sdk.kotlin.services.databasemigrationservice.model.RecommendationData? = builder.data
/**
* The identifier of the source database for which Fleet Advisor provided this recommendation.
*/
public val databaseId: kotlin.String? = builder.databaseId
/**
* The name of the target engine. Valid values include `"rds-aurora-mysql"`, `"rds-aurora-postgresql"`, `"rds-mysql"`, `"rds-oracle"`, `"rds-sql-server"`, and `"rds-postgresql"`.
*/
public val engineName: kotlin.String? = builder.engineName
/**
* Indicates that this target is the rightsized migration destination.
*/
public val preferred: kotlin.Boolean? = builder.preferred
/**
* The settings in JSON format for the preferred target engine parameters. These parameters include capacity, resource utilization, and the usage type (production, development, or testing).
*/
public val settings: aws.sdk.kotlin.services.databasemigrationservice.model.RecommendationSettings? = builder.settings
/**
* The status of the target engine recommendation. Valid values include `"alternate"`, `"in-progress"`, `"not-viable"`, and `"recommended"`.
*/
public val status: kotlin.String? = builder.status
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.Recommendation = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Recommendation(")
append("createdDate=$createdDate,")
append("data=$data,")
append("databaseId=$databaseId,")
append("engineName=$engineName,")
append("preferred=$preferred,")
append("settings=$settings,")
append("status=$status")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = createdDate?.hashCode() ?: 0
result = 31 * result + (data?.hashCode() ?: 0)
result = 31 * result + (databaseId?.hashCode() ?: 0)
result = 31 * result + (engineName?.hashCode() ?: 0)
result = 31 * result + (preferred?.hashCode() ?: 0)
result = 31 * result + (settings?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Recommendation
if (createdDate != other.createdDate) return false
if (data != other.data) return false
if (databaseId != other.databaseId) return false
if (engineName != other.engineName) return false
if (preferred != other.preferred) return false
if (settings != other.settings) return false
if (status != other.status) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.Recommendation = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The date when Fleet Advisor created the target engine recommendation.
*/
public var createdDate: kotlin.String? = null
/**
* The recommendation of a target engine for the specified source database.
*/
public var data: aws.sdk.kotlin.services.databasemigrationservice.model.RecommendationData? = null
/**
* The identifier of the source database for which Fleet Advisor provided this recommendation.
*/
public var databaseId: kotlin.String? = null
/**
* The name of the target engine. Valid values include `"rds-aurora-mysql"`, `"rds-aurora-postgresql"`, `"rds-mysql"`, `"rds-oracle"`, `"rds-sql-server"`, and `"rds-postgresql"`.
*/
public var engineName: kotlin.String? = null
/**
* Indicates that this target is the rightsized migration destination.
*/
public var preferred: kotlin.Boolean? = null
/**
* The settings in JSON format for the preferred target engine parameters. These parameters include capacity, resource utilization, and the usage type (production, development, or testing).
*/
public var settings: aws.sdk.kotlin.services.databasemigrationservice.model.RecommendationSettings? = null
/**
* The status of the target engine recommendation. Valid values include `"alternate"`, `"in-progress"`, `"not-viable"`, and `"recommended"`.
*/
public var status: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.Recommendation) : this() {
this.createdDate = x.createdDate
this.data = x.data
this.databaseId = x.databaseId
this.engineName = x.engineName
this.preferred = x.preferred
this.settings = x.settings
this.status = x.status
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.Recommendation = Recommendation(this)
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.RecommendationData] inside the given [block]
*/
public fun data(block: aws.sdk.kotlin.services.databasemigrationservice.model.RecommendationData.Builder.() -> kotlin.Unit) {
this.data = aws.sdk.kotlin.services.databasemigrationservice.model.RecommendationData.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.RecommendationSettings] inside the given [block]
*/
public fun settings(block: aws.sdk.kotlin.services.databasemigrationservice.model.RecommendationSettings.Builder.() -> kotlin.Unit) {
this.settings = aws.sdk.kotlin.services.databasemigrationservice.model.RecommendationSettings.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy