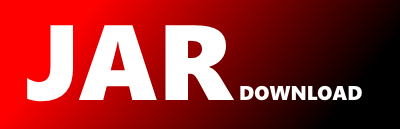
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.RedisSettings.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Provides information that defines a Redis target endpoint.
*/
public class RedisSettings private constructor(builder: Builder) {
/**
* The password provided with the `auth-role` and `auth-token` options of the `AuthType` setting for a Redis target endpoint.
*/
public val authPassword: kotlin.String? = builder.authPassword
/**
* The type of authentication to perform when connecting to a Redis target. Options include `none`, `auth-token`, and `auth-role`. The `auth-token` option requires an `AuthPassword` value to be provided. The `auth-role` option requires `AuthUserName` and `AuthPassword` values to be provided.
*/
public val authType: aws.sdk.kotlin.services.databasemigrationservice.model.RedisAuthTypeValue? = builder.authType
/**
* The user name provided with the `auth-role` option of the `AuthType` setting for a Redis target endpoint.
*/
public val authUserName: kotlin.String? = builder.authUserName
/**
* Transmission Control Protocol (TCP) port for the endpoint.
*/
public val port: kotlin.Int = builder.port
/**
* Fully qualified domain name of the endpoint.
*/
public val serverName: kotlin.String = requireNotNull(builder.serverName) { "A non-null value must be provided for serverName" }
/**
* The Amazon Resource Name (ARN) for the certificate authority (CA) that DMS uses to connect to your Redis target endpoint.
*/
public val sslCaCertificateArn: kotlin.String? = builder.sslCaCertificateArn
/**
* The connection to a Redis target endpoint using Transport Layer Security (TLS). Valid values include `plaintext` and `ssl-encryption`. The default is `ssl-encryption`. The `ssl-encryption` option makes an encrypted connection. Optionally, you can identify an Amazon Resource Name (ARN) for an SSL certificate authority (CA) using the `SslCaCertificateArn `setting. If an ARN isn't given for a CA, DMS uses the Amazon root CA.
*
* The `plaintext` option doesn't provide Transport Layer Security (TLS) encryption for traffic between endpoint and database.
*/
public val sslSecurityProtocol: aws.sdk.kotlin.services.databasemigrationservice.model.SslSecurityProtocolValue? = builder.sslSecurityProtocol
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.RedisSettings = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("RedisSettings(")
append("authPassword=*** Sensitive Data Redacted ***,")
append("authType=$authType,")
append("authUserName=$authUserName,")
append("port=$port,")
append("serverName=$serverName,")
append("sslCaCertificateArn=$sslCaCertificateArn,")
append("sslSecurityProtocol=$sslSecurityProtocol")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = authPassword?.hashCode() ?: 0
result = 31 * result + (authType?.hashCode() ?: 0)
result = 31 * result + (authUserName?.hashCode() ?: 0)
result = 31 * result + (port)
result = 31 * result + (serverName.hashCode())
result = 31 * result + (sslCaCertificateArn?.hashCode() ?: 0)
result = 31 * result + (sslSecurityProtocol?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as RedisSettings
if (authPassword != other.authPassword) return false
if (authType != other.authType) return false
if (authUserName != other.authUserName) return false
if (port != other.port) return false
if (serverName != other.serverName) return false
if (sslCaCertificateArn != other.sslCaCertificateArn) return false
if (sslSecurityProtocol != other.sslSecurityProtocol) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.RedisSettings = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The password provided with the `auth-role` and `auth-token` options of the `AuthType` setting for a Redis target endpoint.
*/
public var authPassword: kotlin.String? = null
/**
* The type of authentication to perform when connecting to a Redis target. Options include `none`, `auth-token`, and `auth-role`. The `auth-token` option requires an `AuthPassword` value to be provided. The `auth-role` option requires `AuthUserName` and `AuthPassword` values to be provided.
*/
public var authType: aws.sdk.kotlin.services.databasemigrationservice.model.RedisAuthTypeValue? = null
/**
* The user name provided with the `auth-role` option of the `AuthType` setting for a Redis target endpoint.
*/
public var authUserName: kotlin.String? = null
/**
* Transmission Control Protocol (TCP) port for the endpoint.
*/
public var port: kotlin.Int = 0
/**
* Fully qualified domain name of the endpoint.
*/
public var serverName: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) for the certificate authority (CA) that DMS uses to connect to your Redis target endpoint.
*/
public var sslCaCertificateArn: kotlin.String? = null
/**
* The connection to a Redis target endpoint using Transport Layer Security (TLS). Valid values include `plaintext` and `ssl-encryption`. The default is `ssl-encryption`. The `ssl-encryption` option makes an encrypted connection. Optionally, you can identify an Amazon Resource Name (ARN) for an SSL certificate authority (CA) using the `SslCaCertificateArn `setting. If an ARN isn't given for a CA, DMS uses the Amazon root CA.
*
* The `plaintext` option doesn't provide Transport Layer Security (TLS) encryption for traffic between endpoint and database.
*/
public var sslSecurityProtocol: aws.sdk.kotlin.services.databasemigrationservice.model.SslSecurityProtocolValue? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.RedisSettings) : this() {
this.authPassword = x.authPassword
this.authType = x.authType
this.authUserName = x.authUserName
this.port = x.port
this.serverName = x.serverName
this.sslCaCertificateArn = x.sslCaCertificateArn
this.sslSecurityProtocol = x.sslSecurityProtocol
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.RedisSettings = RedisSettings(this)
internal fun correctErrors(): Builder {
if (serverName == null) serverName = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy