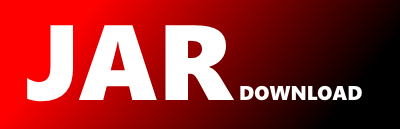
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.Replication.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Provides information that describes a serverless replication created by the `CreateReplication` operation.
*/
public class Replication private constructor(builder: Builder) {
/**
* Indicates the start time for a change data capture (CDC) operation. Use either `CdcStartTime` or `CdcStartPosition` to specify when you want a CDC operation to start. Specifying both values results in an error.
*/
public val cdcStartPosition: kotlin.String? = builder.cdcStartPosition
/**
* Indicates the start time for a change data capture (CDC) operation. Use either `CdcStartTime` or `CdcStartPosition` to specify when you want a CDC operation to start. Specifying both values results in an error.
*/
public val cdcStartTime: aws.smithy.kotlin.runtime.time.Instant? = builder.cdcStartTime
/**
* Indicates when you want a change data capture (CDC) operation to stop. The value can be either server time or commit time.
*/
public val cdcStopPosition: kotlin.String? = builder.cdcStopPosition
/**
* Error and other information about why a serverless replication failed.
*/
public val failureMessages: List? = builder.failureMessages
/**
* Information about provisioning resources for an DMS serverless replication.
*/
public val provisionData: aws.sdk.kotlin.services.databasemigrationservice.model.ProvisionData? = builder.provisionData
/**
* Indicates the last checkpoint that occurred during a change data capture (CDC) operation. You can provide this value to the `CdcStartPosition` parameter to start a CDC operation that begins at that checkpoint.
*/
public val recoveryCheckpoint: kotlin.String? = builder.recoveryCheckpoint
/**
* The Amazon Resource Name for the `ReplicationConfig` associated with the replication.
*/
public val replicationConfigArn: kotlin.String? = builder.replicationConfigArn
/**
* The identifier for the `ReplicationConfig` associated with the replication.
*/
public val replicationConfigIdentifier: kotlin.String? = builder.replicationConfigIdentifier
/**
* The time the serverless replication was created.
*/
public val replicationCreateTime: aws.smithy.kotlin.runtime.time.Instant? = builder.replicationCreateTime
/**
* The timestamp when DMS will deprovision the replication.
*/
public val replicationDeprovisionTime: aws.smithy.kotlin.runtime.time.Instant? = builder.replicationDeprovisionTime
/**
* The timestamp when replication was last stopped.
*/
public val replicationLastStopTime: aws.smithy.kotlin.runtime.time.Instant? = builder.replicationLastStopTime
/**
* This object provides a collection of statistics about a serverless replication.
*/
public val replicationStats: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationStats? = builder.replicationStats
/**
* The type of the serverless replication.
*/
public val replicationType: aws.sdk.kotlin.services.databasemigrationservice.model.MigrationTypeValue? = builder.replicationType
/**
* The time the serverless replication was updated.
*/
public val replicationUpdateTime: aws.smithy.kotlin.runtime.time.Instant? = builder.replicationUpdateTime
/**
* The Amazon Resource Name for an existing `Endpoint` the serverless replication uses for its data source.
*/
public val sourceEndpointArn: kotlin.String? = builder.sourceEndpointArn
/**
* The type of replication to start.
*/
public val startReplicationType: kotlin.String? = builder.startReplicationType
/**
* The current status of the serverless replication.
*/
public val status: kotlin.String? = builder.status
/**
* The reason the replication task was stopped. This response parameter can return one of the following values:
* + `"Stop Reason NORMAL"`
* + `"Stop Reason RECOVERABLE_ERROR"`
* + `"Stop Reason FATAL_ERROR"`
* + `"Stop Reason FULL_LOAD_ONLY_FINISHED"`
* + `"Stop Reason STOPPED_AFTER_FULL_LOAD"` – Full load completed, with cached changes not applied
* + `"Stop Reason STOPPED_AFTER_CACHED_EVENTS"` – Full load completed, with cached changes applied
* + `"Stop Reason EXPRESS_LICENSE_LIMITS_REACHED"`
* + `"Stop Reason STOPPED_AFTER_DDL_APPLY"` – User-defined stop task after DDL applied
* + `"Stop Reason STOPPED_DUE_TO_LOW_MEMORY"`
* + `"Stop Reason STOPPED_DUE_TO_LOW_DISK"`
* + `"Stop Reason STOPPED_AT_SERVER_TIME"` – User-defined server time for stopping task
* + `"Stop Reason STOPPED_AT_COMMIT_TIME"` – User-defined commit time for stopping task
* + `"Stop Reason RECONFIGURATION_RESTART"`
* + `"Stop Reason RECYCLE_TASK"`
*/
public val stopReason: kotlin.String? = builder.stopReason
/**
* The Amazon Resource Name for an existing `Endpoint` the serverless replication uses for its data target.
*/
public val targetEndpointArn: kotlin.String? = builder.targetEndpointArn
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.Replication = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Replication(")
append("cdcStartPosition=$cdcStartPosition,")
append("cdcStartTime=$cdcStartTime,")
append("cdcStopPosition=$cdcStopPosition,")
append("failureMessages=$failureMessages,")
append("provisionData=$provisionData,")
append("recoveryCheckpoint=$recoveryCheckpoint,")
append("replicationConfigArn=$replicationConfigArn,")
append("replicationConfigIdentifier=$replicationConfigIdentifier,")
append("replicationCreateTime=$replicationCreateTime,")
append("replicationDeprovisionTime=$replicationDeprovisionTime,")
append("replicationLastStopTime=$replicationLastStopTime,")
append("replicationStats=$replicationStats,")
append("replicationType=$replicationType,")
append("replicationUpdateTime=$replicationUpdateTime,")
append("sourceEndpointArn=$sourceEndpointArn,")
append("startReplicationType=$startReplicationType,")
append("status=$status,")
append("stopReason=$stopReason,")
append("targetEndpointArn=$targetEndpointArn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = cdcStartPosition?.hashCode() ?: 0
result = 31 * result + (cdcStartTime?.hashCode() ?: 0)
result = 31 * result + (cdcStopPosition?.hashCode() ?: 0)
result = 31 * result + (failureMessages?.hashCode() ?: 0)
result = 31 * result + (provisionData?.hashCode() ?: 0)
result = 31 * result + (recoveryCheckpoint?.hashCode() ?: 0)
result = 31 * result + (replicationConfigArn?.hashCode() ?: 0)
result = 31 * result + (replicationConfigIdentifier?.hashCode() ?: 0)
result = 31 * result + (replicationCreateTime?.hashCode() ?: 0)
result = 31 * result + (replicationDeprovisionTime?.hashCode() ?: 0)
result = 31 * result + (replicationLastStopTime?.hashCode() ?: 0)
result = 31 * result + (replicationStats?.hashCode() ?: 0)
result = 31 * result + (replicationType?.hashCode() ?: 0)
result = 31 * result + (replicationUpdateTime?.hashCode() ?: 0)
result = 31 * result + (sourceEndpointArn?.hashCode() ?: 0)
result = 31 * result + (startReplicationType?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (stopReason?.hashCode() ?: 0)
result = 31 * result + (targetEndpointArn?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Replication
if (cdcStartPosition != other.cdcStartPosition) return false
if (cdcStartTime != other.cdcStartTime) return false
if (cdcStopPosition != other.cdcStopPosition) return false
if (failureMessages != other.failureMessages) return false
if (provisionData != other.provisionData) return false
if (recoveryCheckpoint != other.recoveryCheckpoint) return false
if (replicationConfigArn != other.replicationConfigArn) return false
if (replicationConfigIdentifier != other.replicationConfigIdentifier) return false
if (replicationCreateTime != other.replicationCreateTime) return false
if (replicationDeprovisionTime != other.replicationDeprovisionTime) return false
if (replicationLastStopTime != other.replicationLastStopTime) return false
if (replicationStats != other.replicationStats) return false
if (replicationType != other.replicationType) return false
if (replicationUpdateTime != other.replicationUpdateTime) return false
if (sourceEndpointArn != other.sourceEndpointArn) return false
if (startReplicationType != other.startReplicationType) return false
if (status != other.status) return false
if (stopReason != other.stopReason) return false
if (targetEndpointArn != other.targetEndpointArn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.Replication = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Indicates the start time for a change data capture (CDC) operation. Use either `CdcStartTime` or `CdcStartPosition` to specify when you want a CDC operation to start. Specifying both values results in an error.
*/
public var cdcStartPosition: kotlin.String? = null
/**
* Indicates the start time for a change data capture (CDC) operation. Use either `CdcStartTime` or `CdcStartPosition` to specify when you want a CDC operation to start. Specifying both values results in an error.
*/
public var cdcStartTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Indicates when you want a change data capture (CDC) operation to stop. The value can be either server time or commit time.
*/
public var cdcStopPosition: kotlin.String? = null
/**
* Error and other information about why a serverless replication failed.
*/
public var failureMessages: List? = null
/**
* Information about provisioning resources for an DMS serverless replication.
*/
public var provisionData: aws.sdk.kotlin.services.databasemigrationservice.model.ProvisionData? = null
/**
* Indicates the last checkpoint that occurred during a change data capture (CDC) operation. You can provide this value to the `CdcStartPosition` parameter to start a CDC operation that begins at that checkpoint.
*/
public var recoveryCheckpoint: kotlin.String? = null
/**
* The Amazon Resource Name for the `ReplicationConfig` associated with the replication.
*/
public var replicationConfigArn: kotlin.String? = null
/**
* The identifier for the `ReplicationConfig` associated with the replication.
*/
public var replicationConfigIdentifier: kotlin.String? = null
/**
* The time the serverless replication was created.
*/
public var replicationCreateTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The timestamp when DMS will deprovision the replication.
*/
public var replicationDeprovisionTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The timestamp when replication was last stopped.
*/
public var replicationLastStopTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* This object provides a collection of statistics about a serverless replication.
*/
public var replicationStats: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationStats? = null
/**
* The type of the serverless replication.
*/
public var replicationType: aws.sdk.kotlin.services.databasemigrationservice.model.MigrationTypeValue? = null
/**
* The time the serverless replication was updated.
*/
public var replicationUpdateTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The Amazon Resource Name for an existing `Endpoint` the serverless replication uses for its data source.
*/
public var sourceEndpointArn: kotlin.String? = null
/**
* The type of replication to start.
*/
public var startReplicationType: kotlin.String? = null
/**
* The current status of the serverless replication.
*/
public var status: kotlin.String? = null
/**
* The reason the replication task was stopped. This response parameter can return one of the following values:
* + `"Stop Reason NORMAL"`
* + `"Stop Reason RECOVERABLE_ERROR"`
* + `"Stop Reason FATAL_ERROR"`
* + `"Stop Reason FULL_LOAD_ONLY_FINISHED"`
* + `"Stop Reason STOPPED_AFTER_FULL_LOAD"` – Full load completed, with cached changes not applied
* + `"Stop Reason STOPPED_AFTER_CACHED_EVENTS"` – Full load completed, with cached changes applied
* + `"Stop Reason EXPRESS_LICENSE_LIMITS_REACHED"`
* + `"Stop Reason STOPPED_AFTER_DDL_APPLY"` – User-defined stop task after DDL applied
* + `"Stop Reason STOPPED_DUE_TO_LOW_MEMORY"`
* + `"Stop Reason STOPPED_DUE_TO_LOW_DISK"`
* + `"Stop Reason STOPPED_AT_SERVER_TIME"` – User-defined server time for stopping task
* + `"Stop Reason STOPPED_AT_COMMIT_TIME"` – User-defined commit time for stopping task
* + `"Stop Reason RECONFIGURATION_RESTART"`
* + `"Stop Reason RECYCLE_TASK"`
*/
public var stopReason: kotlin.String? = null
/**
* The Amazon Resource Name for an existing `Endpoint` the serverless replication uses for its data target.
*/
public var targetEndpointArn: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.Replication) : this() {
this.cdcStartPosition = x.cdcStartPosition
this.cdcStartTime = x.cdcStartTime
this.cdcStopPosition = x.cdcStopPosition
this.failureMessages = x.failureMessages
this.provisionData = x.provisionData
this.recoveryCheckpoint = x.recoveryCheckpoint
this.replicationConfigArn = x.replicationConfigArn
this.replicationConfigIdentifier = x.replicationConfigIdentifier
this.replicationCreateTime = x.replicationCreateTime
this.replicationDeprovisionTime = x.replicationDeprovisionTime
this.replicationLastStopTime = x.replicationLastStopTime
this.replicationStats = x.replicationStats
this.replicationType = x.replicationType
this.replicationUpdateTime = x.replicationUpdateTime
this.sourceEndpointArn = x.sourceEndpointArn
this.startReplicationType = x.startReplicationType
this.status = x.status
this.stopReason = x.stopReason
this.targetEndpointArn = x.targetEndpointArn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.Replication = Replication(this)
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.ProvisionData] inside the given [block]
*/
public fun provisionData(block: aws.sdk.kotlin.services.databasemigrationservice.model.ProvisionData.Builder.() -> kotlin.Unit) {
this.provisionData = aws.sdk.kotlin.services.databasemigrationservice.model.ProvisionData.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationStats] inside the given [block]
*/
public fun replicationStats(block: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationStats.Builder.() -> kotlin.Unit) {
this.replicationStats = aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationStats.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy