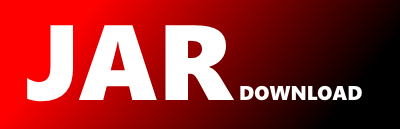
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* This object provides configuration information about a serverless replication.
*/
public class ReplicationConfig private constructor(builder: Builder) {
/**
* Configuration parameters for provisioning an DMS serverless replication.
*/
public val computeConfig: aws.sdk.kotlin.services.databasemigrationservice.model.ComputeConfig? = builder.computeConfig
/**
* The Amazon Resource Name (ARN) of this DMS Serverless replication configuration.
*/
public val replicationConfigArn: kotlin.String? = builder.replicationConfigArn
/**
* The time the serverless replication config was created.
*/
public val replicationConfigCreateTime: aws.smithy.kotlin.runtime.time.Instant? = builder.replicationConfigCreateTime
/**
* The identifier for the `ReplicationConfig` associated with the replication.
*/
public val replicationConfigIdentifier: kotlin.String? = builder.replicationConfigIdentifier
/**
* The time the serverless replication config was updated.
*/
public val replicationConfigUpdateTime: aws.smithy.kotlin.runtime.time.Instant? = builder.replicationConfigUpdateTime
/**
* Configuration parameters for an DMS serverless replication.
*/
public val replicationSettings: kotlin.String? = builder.replicationSettings
/**
* The type of the replication.
*/
public val replicationType: aws.sdk.kotlin.services.databasemigrationservice.model.MigrationTypeValue? = builder.replicationType
/**
* The Amazon Resource Name (ARN) of the source endpoint for this DMS serverless replication configuration.
*/
public val sourceEndpointArn: kotlin.String? = builder.sourceEndpointArn
/**
* Additional parameters for an DMS serverless replication.
*/
public val supplementalSettings: kotlin.String? = builder.supplementalSettings
/**
* Table mappings specified in the replication.
*/
public val tableMappings: kotlin.String? = builder.tableMappings
/**
* The Amazon Resource Name (ARN) of the target endpoint for this DMS serverless replication configuration.
*/
public val targetEndpointArn: kotlin.String? = builder.targetEndpointArn
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationConfig = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ReplicationConfig(")
append("computeConfig=$computeConfig,")
append("replicationConfigArn=$replicationConfigArn,")
append("replicationConfigCreateTime=$replicationConfigCreateTime,")
append("replicationConfigIdentifier=$replicationConfigIdentifier,")
append("replicationConfigUpdateTime=$replicationConfigUpdateTime,")
append("replicationSettings=$replicationSettings,")
append("replicationType=$replicationType,")
append("sourceEndpointArn=$sourceEndpointArn,")
append("supplementalSettings=$supplementalSettings,")
append("tableMappings=$tableMappings,")
append("targetEndpointArn=$targetEndpointArn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = computeConfig?.hashCode() ?: 0
result = 31 * result + (replicationConfigArn?.hashCode() ?: 0)
result = 31 * result + (replicationConfigCreateTime?.hashCode() ?: 0)
result = 31 * result + (replicationConfigIdentifier?.hashCode() ?: 0)
result = 31 * result + (replicationConfigUpdateTime?.hashCode() ?: 0)
result = 31 * result + (replicationSettings?.hashCode() ?: 0)
result = 31 * result + (replicationType?.hashCode() ?: 0)
result = 31 * result + (sourceEndpointArn?.hashCode() ?: 0)
result = 31 * result + (supplementalSettings?.hashCode() ?: 0)
result = 31 * result + (tableMappings?.hashCode() ?: 0)
result = 31 * result + (targetEndpointArn?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ReplicationConfig
if (computeConfig != other.computeConfig) return false
if (replicationConfigArn != other.replicationConfigArn) return false
if (replicationConfigCreateTime != other.replicationConfigCreateTime) return false
if (replicationConfigIdentifier != other.replicationConfigIdentifier) return false
if (replicationConfigUpdateTime != other.replicationConfigUpdateTime) return false
if (replicationSettings != other.replicationSettings) return false
if (replicationType != other.replicationType) return false
if (sourceEndpointArn != other.sourceEndpointArn) return false
if (supplementalSettings != other.supplementalSettings) return false
if (tableMappings != other.tableMappings) return false
if (targetEndpointArn != other.targetEndpointArn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationConfig = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Configuration parameters for provisioning an DMS serverless replication.
*/
public var computeConfig: aws.sdk.kotlin.services.databasemigrationservice.model.ComputeConfig? = null
/**
* The Amazon Resource Name (ARN) of this DMS Serverless replication configuration.
*/
public var replicationConfigArn: kotlin.String? = null
/**
* The time the serverless replication config was created.
*/
public var replicationConfigCreateTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The identifier for the `ReplicationConfig` associated with the replication.
*/
public var replicationConfigIdentifier: kotlin.String? = null
/**
* The time the serverless replication config was updated.
*/
public var replicationConfigUpdateTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Configuration parameters for an DMS serverless replication.
*/
public var replicationSettings: kotlin.String? = null
/**
* The type of the replication.
*/
public var replicationType: aws.sdk.kotlin.services.databasemigrationservice.model.MigrationTypeValue? = null
/**
* The Amazon Resource Name (ARN) of the source endpoint for this DMS serverless replication configuration.
*/
public var sourceEndpointArn: kotlin.String? = null
/**
* Additional parameters for an DMS serverless replication.
*/
public var supplementalSettings: kotlin.String? = null
/**
* Table mappings specified in the replication.
*/
public var tableMappings: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the target endpoint for this DMS serverless replication configuration.
*/
public var targetEndpointArn: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationConfig) : this() {
this.computeConfig = x.computeConfig
this.replicationConfigArn = x.replicationConfigArn
this.replicationConfigCreateTime = x.replicationConfigCreateTime
this.replicationConfigIdentifier = x.replicationConfigIdentifier
this.replicationConfigUpdateTime = x.replicationConfigUpdateTime
this.replicationSettings = x.replicationSettings
this.replicationType = x.replicationType
this.sourceEndpointArn = x.sourceEndpointArn
this.supplementalSettings = x.supplementalSettings
this.tableMappings = x.tableMappings
this.targetEndpointArn = x.targetEndpointArn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationConfig = ReplicationConfig(this)
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.ComputeConfig] inside the given [block]
*/
public fun computeConfig(block: aws.sdk.kotlin.services.databasemigrationservice.model.ComputeConfig.Builder.() -> kotlin.Unit) {
this.computeConfig = aws.sdk.kotlin.services.databasemigrationservice.model.ComputeConfig.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy