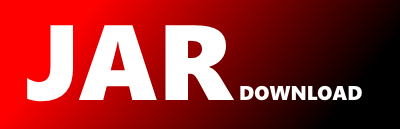
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationInstance.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Provides information that defines a replication instance.
*/
public class ReplicationInstance private constructor(builder: Builder) {
/**
* The amount of storage (in gigabytes) that is allocated for the replication instance.
*/
public val allocatedStorage: kotlin.Int = builder.allocatedStorage
/**
* Boolean value indicating if minor version upgrades will be automatically applied to the instance.
*/
public val autoMinorVersionUpgrade: kotlin.Boolean = builder.autoMinorVersionUpgrade
/**
* The Availability Zone for the instance.
*/
public val availabilityZone: kotlin.String? = builder.availabilityZone
/**
* The DNS name servers supported for the replication instance to access your on-premise source or target database.
*/
public val dnsNameServers: kotlin.String? = builder.dnsNameServers
/**
* The engine version number of the replication instance.
*
* If an engine version number is not specified when a replication instance is created, the default is the latest engine version available.
*
* When modifying a major engine version of an instance, also set `AllowMajorVersionUpgrade` to `true`.
*/
public val engineVersion: kotlin.String? = builder.engineVersion
/**
* The expiration date of the free replication instance that is part of the Free DMS program.
*/
public val freeUntil: aws.smithy.kotlin.runtime.time.Instant? = builder.freeUntil
/**
* The time the replication instance was created.
*/
public val instanceCreateTime: aws.smithy.kotlin.runtime.time.Instant? = builder.instanceCreateTime
/**
* Specifies the ID of the secret that stores the key cache file required for kerberos authentication, when replicating an instance.
*/
public val kerberosAuthenticationSettings: aws.sdk.kotlin.services.databasemigrationservice.model.KerberosAuthenticationSettings? = builder.kerberosAuthenticationSettings
/**
* An KMS key identifier that is used to encrypt the data on the replication instance.
*
* If you don't specify a value for the `KmsKeyId` parameter, then DMS uses your default encryption key.
*
* KMS creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services account has a different default encryption key for each Amazon Web Services Region.
*/
public val kmsKeyId: kotlin.String? = builder.kmsKeyId
/**
* Specifies whether the replication instance is a Multi-AZ deployment. You can't set the `AvailabilityZone` parameter if the Multi-AZ parameter is set to `true`.
*/
public val multiAz: kotlin.Boolean = builder.multiAz
/**
* The type of IP address protocol used by a replication instance, such as IPv4 only or Dual-stack that supports both IPv4 and IPv6 addressing. IPv6 only is not yet supported.
*/
public val networkType: kotlin.String? = builder.networkType
/**
* The pending modification values.
*/
public val pendingModifiedValues: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationPendingModifiedValues? = builder.pendingModifiedValues
/**
* The maintenance window times for the replication instance. Any pending upgrades to the replication instance are performed during this time.
*/
public val preferredMaintenanceWindow: kotlin.String? = builder.preferredMaintenanceWindow
/**
* Specifies the accessibility options for the replication instance. A value of `true` represents an instance with a public IP address. A value of `false` represents an instance with a private IP address. The default value is `true`.
*/
public val publiclyAccessible: kotlin.Boolean = builder.publiclyAccessible
/**
* The Amazon Resource Name (ARN) of the replication instance.
*/
public val replicationInstanceArn: kotlin.String? = builder.replicationInstanceArn
/**
* The compute and memory capacity of the replication instance as defined for the specified replication instance class. It is a required parameter, although a default value is pre-selected in the DMS console.
*
* For more information on the settings and capacities for the available replication instance classes, see [ Selecting the right DMS replication instance for your migration](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_ReplicationInstance.html#CHAP_ReplicationInstance.InDepth).
*/
public val replicationInstanceClass: kotlin.String? = builder.replicationInstanceClass
/**
* The replication instance identifier is a required parameter. This parameter is stored as a lowercase string.
*
* Constraints:
* + Must contain 1-63 alphanumeric characters or hyphens.
* + First character must be a letter.
* + Cannot end with a hyphen or contain two consecutive hyphens.
*
* Example: `myrepinstance`
*/
public val replicationInstanceIdentifier: kotlin.String? = builder.replicationInstanceIdentifier
/**
* One or more IPv6 addresses for the replication instance.
*/
public val replicationInstanceIpv6Addresses: List? = builder.replicationInstanceIpv6Addresses
/**
* The private IP address of the replication instance.
*/
@Deprecated("No longer recommended for use. See AWS API documentation for more details.")
public val replicationInstancePrivateIpAddress: kotlin.String? = builder.replicationInstancePrivateIpAddress
/**
* One or more private IP addresses for the replication instance.
*/
public val replicationInstancePrivateIpAddresses: List? = builder.replicationInstancePrivateIpAddresses
/**
* The public IP address of the replication instance.
*/
@Deprecated("No longer recommended for use. See AWS API documentation for more details.")
public val replicationInstancePublicIpAddress: kotlin.String? = builder.replicationInstancePublicIpAddress
/**
* One or more public IP addresses for the replication instance.
*/
public val replicationInstancePublicIpAddresses: List? = builder.replicationInstancePublicIpAddresses
/**
* The status of the replication instance. The possible return values include:
* + `"available"`
* + `"creating"`
* + `"deleted"`
* + `"deleting"`
* + `"failed"`
* + `"modifying"`
* + `"upgrading"`
* + `"rebooting"`
* + `"resetting-master-credentials"`
* + `"storage-full"`
* + `"incompatible-credentials"`
* + `"incompatible-network"`
* + `"maintenance"`
*/
public val replicationInstanceStatus: kotlin.String? = builder.replicationInstanceStatus
/**
* The subnet group for the replication instance.
*/
public val replicationSubnetGroup: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationSubnetGroup? = builder.replicationSubnetGroup
/**
* The Availability Zone of the standby replication instance in a Multi-AZ deployment.
*/
public val secondaryAvailabilityZone: kotlin.String? = builder.secondaryAvailabilityZone
/**
* The VPC security group for the instance.
*/
public val vpcSecurityGroups: List? = builder.vpcSecurityGroups
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationInstance = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ReplicationInstance(")
append("allocatedStorage=$allocatedStorage,")
append("autoMinorVersionUpgrade=$autoMinorVersionUpgrade,")
append("availabilityZone=$availabilityZone,")
append("dnsNameServers=$dnsNameServers,")
append("engineVersion=$engineVersion,")
append("freeUntil=$freeUntil,")
append("instanceCreateTime=$instanceCreateTime,")
append("kerberosAuthenticationSettings=$kerberosAuthenticationSettings,")
append("kmsKeyId=$kmsKeyId,")
append("multiAz=$multiAz,")
append("networkType=$networkType,")
append("pendingModifiedValues=$pendingModifiedValues,")
append("preferredMaintenanceWindow=$preferredMaintenanceWindow,")
append("publiclyAccessible=$publiclyAccessible,")
append("replicationInstanceArn=$replicationInstanceArn,")
append("replicationInstanceClass=$replicationInstanceClass,")
append("replicationInstanceIdentifier=$replicationInstanceIdentifier,")
append("replicationInstanceIpv6Addresses=$replicationInstanceIpv6Addresses,")
append("replicationInstancePrivateIpAddress=$replicationInstancePrivateIpAddress,")
append("replicationInstancePrivateIpAddresses=$replicationInstancePrivateIpAddresses,")
append("replicationInstancePublicIpAddress=$replicationInstancePublicIpAddress,")
append("replicationInstancePublicIpAddresses=$replicationInstancePublicIpAddresses,")
append("replicationInstanceStatus=$replicationInstanceStatus,")
append("replicationSubnetGroup=$replicationSubnetGroup,")
append("secondaryAvailabilityZone=$secondaryAvailabilityZone,")
append("vpcSecurityGroups=$vpcSecurityGroups")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = allocatedStorage
result = 31 * result + (autoMinorVersionUpgrade.hashCode())
result = 31 * result + (availabilityZone?.hashCode() ?: 0)
result = 31 * result + (dnsNameServers?.hashCode() ?: 0)
result = 31 * result + (engineVersion?.hashCode() ?: 0)
result = 31 * result + (freeUntil?.hashCode() ?: 0)
result = 31 * result + (instanceCreateTime?.hashCode() ?: 0)
result = 31 * result + (kerberosAuthenticationSettings?.hashCode() ?: 0)
result = 31 * result + (kmsKeyId?.hashCode() ?: 0)
result = 31 * result + (multiAz.hashCode())
result = 31 * result + (networkType?.hashCode() ?: 0)
result = 31 * result + (pendingModifiedValues?.hashCode() ?: 0)
result = 31 * result + (preferredMaintenanceWindow?.hashCode() ?: 0)
result = 31 * result + (publiclyAccessible.hashCode())
result = 31 * result + (replicationInstanceArn?.hashCode() ?: 0)
result = 31 * result + (replicationInstanceClass?.hashCode() ?: 0)
result = 31 * result + (replicationInstanceIdentifier?.hashCode() ?: 0)
result = 31 * result + (replicationInstanceIpv6Addresses?.hashCode() ?: 0)
result = 31 * result + (replicationInstancePrivateIpAddress?.hashCode() ?: 0)
result = 31 * result + (replicationInstancePrivateIpAddresses?.hashCode() ?: 0)
result = 31 * result + (replicationInstancePublicIpAddress?.hashCode() ?: 0)
result = 31 * result + (replicationInstancePublicIpAddresses?.hashCode() ?: 0)
result = 31 * result + (replicationInstanceStatus?.hashCode() ?: 0)
result = 31 * result + (replicationSubnetGroup?.hashCode() ?: 0)
result = 31 * result + (secondaryAvailabilityZone?.hashCode() ?: 0)
result = 31 * result + (vpcSecurityGroups?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ReplicationInstance
if (allocatedStorage != other.allocatedStorage) return false
if (autoMinorVersionUpgrade != other.autoMinorVersionUpgrade) return false
if (availabilityZone != other.availabilityZone) return false
if (dnsNameServers != other.dnsNameServers) return false
if (engineVersion != other.engineVersion) return false
if (freeUntil != other.freeUntil) return false
if (instanceCreateTime != other.instanceCreateTime) return false
if (kerberosAuthenticationSettings != other.kerberosAuthenticationSettings) return false
if (kmsKeyId != other.kmsKeyId) return false
if (multiAz != other.multiAz) return false
if (networkType != other.networkType) return false
if (pendingModifiedValues != other.pendingModifiedValues) return false
if (preferredMaintenanceWindow != other.preferredMaintenanceWindow) return false
if (publiclyAccessible != other.publiclyAccessible) return false
if (replicationInstanceArn != other.replicationInstanceArn) return false
if (replicationInstanceClass != other.replicationInstanceClass) return false
if (replicationInstanceIdentifier != other.replicationInstanceIdentifier) return false
if (replicationInstanceIpv6Addresses != other.replicationInstanceIpv6Addresses) return false
if (replicationInstancePrivateIpAddress != other.replicationInstancePrivateIpAddress) return false
if (replicationInstancePrivateIpAddresses != other.replicationInstancePrivateIpAddresses) return false
if (replicationInstancePublicIpAddress != other.replicationInstancePublicIpAddress) return false
if (replicationInstancePublicIpAddresses != other.replicationInstancePublicIpAddresses) return false
if (replicationInstanceStatus != other.replicationInstanceStatus) return false
if (replicationSubnetGroup != other.replicationSubnetGroup) return false
if (secondaryAvailabilityZone != other.secondaryAvailabilityZone) return false
if (vpcSecurityGroups != other.vpcSecurityGroups) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationInstance = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The amount of storage (in gigabytes) that is allocated for the replication instance.
*/
public var allocatedStorage: kotlin.Int = 0
/**
* Boolean value indicating if minor version upgrades will be automatically applied to the instance.
*/
public var autoMinorVersionUpgrade: kotlin.Boolean = false
/**
* The Availability Zone for the instance.
*/
public var availabilityZone: kotlin.String? = null
/**
* The DNS name servers supported for the replication instance to access your on-premise source or target database.
*/
public var dnsNameServers: kotlin.String? = null
/**
* The engine version number of the replication instance.
*
* If an engine version number is not specified when a replication instance is created, the default is the latest engine version available.
*
* When modifying a major engine version of an instance, also set `AllowMajorVersionUpgrade` to `true`.
*/
public var engineVersion: kotlin.String? = null
/**
* The expiration date of the free replication instance that is part of the Free DMS program.
*/
public var freeUntil: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The time the replication instance was created.
*/
public var instanceCreateTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Specifies the ID of the secret that stores the key cache file required for kerberos authentication, when replicating an instance.
*/
public var kerberosAuthenticationSettings: aws.sdk.kotlin.services.databasemigrationservice.model.KerberosAuthenticationSettings? = null
/**
* An KMS key identifier that is used to encrypt the data on the replication instance.
*
* If you don't specify a value for the `KmsKeyId` parameter, then DMS uses your default encryption key.
*
* KMS creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services account has a different default encryption key for each Amazon Web Services Region.
*/
public var kmsKeyId: kotlin.String? = null
/**
* Specifies whether the replication instance is a Multi-AZ deployment. You can't set the `AvailabilityZone` parameter if the Multi-AZ parameter is set to `true`.
*/
public var multiAz: kotlin.Boolean = false
/**
* The type of IP address protocol used by a replication instance, such as IPv4 only or Dual-stack that supports both IPv4 and IPv6 addressing. IPv6 only is not yet supported.
*/
public var networkType: kotlin.String? = null
/**
* The pending modification values.
*/
public var pendingModifiedValues: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationPendingModifiedValues? = null
/**
* The maintenance window times for the replication instance. Any pending upgrades to the replication instance are performed during this time.
*/
public var preferredMaintenanceWindow: kotlin.String? = null
/**
* Specifies the accessibility options for the replication instance. A value of `true` represents an instance with a public IP address. A value of `false` represents an instance with a private IP address. The default value is `true`.
*/
public var publiclyAccessible: kotlin.Boolean = false
/**
* The Amazon Resource Name (ARN) of the replication instance.
*/
public var replicationInstanceArn: kotlin.String? = null
/**
* The compute and memory capacity of the replication instance as defined for the specified replication instance class. It is a required parameter, although a default value is pre-selected in the DMS console.
*
* For more information on the settings and capacities for the available replication instance classes, see [ Selecting the right DMS replication instance for your migration](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_ReplicationInstance.html#CHAP_ReplicationInstance.InDepth).
*/
public var replicationInstanceClass: kotlin.String? = null
/**
* The replication instance identifier is a required parameter. This parameter is stored as a lowercase string.
*
* Constraints:
* + Must contain 1-63 alphanumeric characters or hyphens.
* + First character must be a letter.
* + Cannot end with a hyphen or contain two consecutive hyphens.
*
* Example: `myrepinstance`
*/
public var replicationInstanceIdentifier: kotlin.String? = null
/**
* One or more IPv6 addresses for the replication instance.
*/
public var replicationInstanceIpv6Addresses: List? = null
/**
* The private IP address of the replication instance.
*/
@Deprecated("No longer recommended for use. See AWS API documentation for more details.")
public var replicationInstancePrivateIpAddress: kotlin.String? = null
/**
* One or more private IP addresses for the replication instance.
*/
public var replicationInstancePrivateIpAddresses: List? = null
/**
* The public IP address of the replication instance.
*/
@Deprecated("No longer recommended for use. See AWS API documentation for more details.")
public var replicationInstancePublicIpAddress: kotlin.String? = null
/**
* One or more public IP addresses for the replication instance.
*/
public var replicationInstancePublicIpAddresses: List? = null
/**
* The status of the replication instance. The possible return values include:
* + `"available"`
* + `"creating"`
* + `"deleted"`
* + `"deleting"`
* + `"failed"`
* + `"modifying"`
* + `"upgrading"`
* + `"rebooting"`
* + `"resetting-master-credentials"`
* + `"storage-full"`
* + `"incompatible-credentials"`
* + `"incompatible-network"`
* + `"maintenance"`
*/
public var replicationInstanceStatus: kotlin.String? = null
/**
* The subnet group for the replication instance.
*/
public var replicationSubnetGroup: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationSubnetGroup? = null
/**
* The Availability Zone of the standby replication instance in a Multi-AZ deployment.
*/
public var secondaryAvailabilityZone: kotlin.String? = null
/**
* The VPC security group for the instance.
*/
public var vpcSecurityGroups: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationInstance) : this() {
this.allocatedStorage = x.allocatedStorage
this.autoMinorVersionUpgrade = x.autoMinorVersionUpgrade
this.availabilityZone = x.availabilityZone
this.dnsNameServers = x.dnsNameServers
this.engineVersion = x.engineVersion
this.freeUntil = x.freeUntil
this.instanceCreateTime = x.instanceCreateTime
this.kerberosAuthenticationSettings = x.kerberosAuthenticationSettings
this.kmsKeyId = x.kmsKeyId
this.multiAz = x.multiAz
this.networkType = x.networkType
this.pendingModifiedValues = x.pendingModifiedValues
this.preferredMaintenanceWindow = x.preferredMaintenanceWindow
this.publiclyAccessible = x.publiclyAccessible
this.replicationInstanceArn = x.replicationInstanceArn
this.replicationInstanceClass = x.replicationInstanceClass
this.replicationInstanceIdentifier = x.replicationInstanceIdentifier
this.replicationInstanceIpv6Addresses = x.replicationInstanceIpv6Addresses
this.replicationInstancePrivateIpAddress = x.replicationInstancePrivateIpAddress
this.replicationInstancePrivateIpAddresses = x.replicationInstancePrivateIpAddresses
this.replicationInstancePublicIpAddress = x.replicationInstancePublicIpAddress
this.replicationInstancePublicIpAddresses = x.replicationInstancePublicIpAddresses
this.replicationInstanceStatus = x.replicationInstanceStatus
this.replicationSubnetGroup = x.replicationSubnetGroup
this.secondaryAvailabilityZone = x.secondaryAvailabilityZone
this.vpcSecurityGroups = x.vpcSecurityGroups
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationInstance = ReplicationInstance(this)
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.KerberosAuthenticationSettings] inside the given [block]
*/
public fun kerberosAuthenticationSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.KerberosAuthenticationSettings.Builder.() -> kotlin.Unit) {
this.kerberosAuthenticationSettings = aws.sdk.kotlin.services.databasemigrationservice.model.KerberosAuthenticationSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationPendingModifiedValues] inside the given [block]
*/
public fun pendingModifiedValues(block: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationPendingModifiedValues.Builder.() -> kotlin.Unit) {
this.pendingModifiedValues = aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationPendingModifiedValues.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationSubnetGroup] inside the given [block]
*/
public fun replicationSubnetGroup(block: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationSubnetGroup.Builder.() -> kotlin.Unit) {
this.replicationSubnetGroup = aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationSubnetGroup.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy