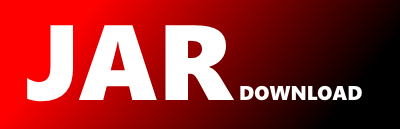
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationStats.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* This object provides a collection of statistics about a serverless replication.
*/
public class ReplicationStats private constructor(builder: Builder) {
/**
* The elapsed time of the replication, in milliseconds.
*/
public val elapsedTimeMillis: kotlin.Long = builder.elapsedTimeMillis
/**
* The date the replication was started either with a fresh start or a target reload.
*/
public val freshStartDate: aws.smithy.kotlin.runtime.time.Instant? = builder.freshStartDate
/**
* The date the replication full load was finished.
*/
public val fullLoadFinishDate: aws.smithy.kotlin.runtime.time.Instant? = builder.fullLoadFinishDate
/**
* The percent complete for the full load serverless replication.
*/
public val fullLoadProgressPercent: kotlin.Int = builder.fullLoadProgressPercent
/**
* The date the replication full load was started.
*/
public val fullLoadStartDate: aws.smithy.kotlin.runtime.time.Instant? = builder.fullLoadStartDate
/**
* The date the replication is scheduled to start.
*/
public val startDate: aws.smithy.kotlin.runtime.time.Instant? = builder.startDate
/**
* The date the replication was stopped.
*/
public val stopDate: aws.smithy.kotlin.runtime.time.Instant? = builder.stopDate
/**
* The number of errors that have occured for this replication.
*/
public val tablesErrored: kotlin.Int = builder.tablesErrored
/**
* The number of tables loaded for this replication.
*/
public val tablesLoaded: kotlin.Int = builder.tablesLoaded
/**
* The number of tables currently loading for this replication.
*/
public val tablesLoading: kotlin.Int = builder.tablesLoading
/**
* The number of tables queued for this replication.
*/
public val tablesQueued: kotlin.Int = builder.tablesQueued
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationStats = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ReplicationStats(")
append("elapsedTimeMillis=$elapsedTimeMillis,")
append("freshStartDate=$freshStartDate,")
append("fullLoadFinishDate=$fullLoadFinishDate,")
append("fullLoadProgressPercent=$fullLoadProgressPercent,")
append("fullLoadStartDate=$fullLoadStartDate,")
append("startDate=$startDate,")
append("stopDate=$stopDate,")
append("tablesErrored=$tablesErrored,")
append("tablesLoaded=$tablesLoaded,")
append("tablesLoading=$tablesLoading,")
append("tablesQueued=$tablesQueued")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = elapsedTimeMillis.hashCode()
result = 31 * result + (freshStartDate?.hashCode() ?: 0)
result = 31 * result + (fullLoadFinishDate?.hashCode() ?: 0)
result = 31 * result + (fullLoadProgressPercent)
result = 31 * result + (fullLoadStartDate?.hashCode() ?: 0)
result = 31 * result + (startDate?.hashCode() ?: 0)
result = 31 * result + (stopDate?.hashCode() ?: 0)
result = 31 * result + (tablesErrored)
result = 31 * result + (tablesLoaded)
result = 31 * result + (tablesLoading)
result = 31 * result + (tablesQueued)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ReplicationStats
if (elapsedTimeMillis != other.elapsedTimeMillis) return false
if (freshStartDate != other.freshStartDate) return false
if (fullLoadFinishDate != other.fullLoadFinishDate) return false
if (fullLoadProgressPercent != other.fullLoadProgressPercent) return false
if (fullLoadStartDate != other.fullLoadStartDate) return false
if (startDate != other.startDate) return false
if (stopDate != other.stopDate) return false
if (tablesErrored != other.tablesErrored) return false
if (tablesLoaded != other.tablesLoaded) return false
if (tablesLoading != other.tablesLoading) return false
if (tablesQueued != other.tablesQueued) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationStats = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The elapsed time of the replication, in milliseconds.
*/
public var elapsedTimeMillis: kotlin.Long = 0L
/**
* The date the replication was started either with a fresh start or a target reload.
*/
public var freshStartDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The date the replication full load was finished.
*/
public var fullLoadFinishDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The percent complete for the full load serverless replication.
*/
public var fullLoadProgressPercent: kotlin.Int = 0
/**
* The date the replication full load was started.
*/
public var fullLoadStartDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The date the replication is scheduled to start.
*/
public var startDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The date the replication was stopped.
*/
public var stopDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The number of errors that have occured for this replication.
*/
public var tablesErrored: kotlin.Int = 0
/**
* The number of tables loaded for this replication.
*/
public var tablesLoaded: kotlin.Int = 0
/**
* The number of tables currently loading for this replication.
*/
public var tablesLoading: kotlin.Int = 0
/**
* The number of tables queued for this replication.
*/
public var tablesQueued: kotlin.Int = 0
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationStats) : this() {
this.elapsedTimeMillis = x.elapsedTimeMillis
this.freshStartDate = x.freshStartDate
this.fullLoadFinishDate = x.fullLoadFinishDate
this.fullLoadProgressPercent = x.fullLoadProgressPercent
this.fullLoadStartDate = x.fullLoadStartDate
this.startDate = x.startDate
this.stopDate = x.stopDate
this.tablesErrored = x.tablesErrored
this.tablesLoaded = x.tablesLoaded
this.tablesLoading = x.tablesLoading
this.tablesQueued = x.tablesQueued
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationStats = ReplicationStats(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy