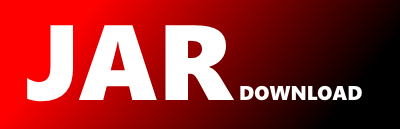
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationTaskAssessmentRun.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Provides information that describes a premigration assessment run that you have started using the `StartReplicationTaskAssessmentRun` operation.
*
* Some of the information appears based on other operations that can return the `ReplicationTaskAssessmentRun` object.
*/
public class ReplicationTaskAssessmentRun private constructor(builder: Builder) {
/**
* Indication of the completion progress for the individual assessments specified to run.
*/
public val assessmentProgress: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationTaskAssessmentRunProgress? = builder.assessmentProgress
/**
* Unique name of the assessment run.
*/
public val assessmentRunName: kotlin.String? = builder.assessmentRunName
/**
* Indicates that the following PreflightAssessmentRun is the latest for the ReplicationTask. The status is either true or false.
*/
public val isLatestTaskAssessmentRun: kotlin.Boolean = builder.isLatestTaskAssessmentRun
/**
* Last message generated by an individual assessment failure.
*/
public val lastFailureMessage: kotlin.String? = builder.lastFailureMessage
/**
* ARN of the migration task associated with this premigration assessment run.
*/
public val replicationTaskArn: kotlin.String? = builder.replicationTaskArn
/**
* Amazon Resource Name (ARN) of this assessment run.
*/
public val replicationTaskAssessmentRunArn: kotlin.String? = builder.replicationTaskAssessmentRunArn
/**
* Date on which the assessment run was created using the `StartReplicationTaskAssessmentRun` operation.
*/
public val replicationTaskAssessmentRunCreationDate: aws.smithy.kotlin.runtime.time.Instant? = builder.replicationTaskAssessmentRunCreationDate
/**
* Encryption mode used to encrypt the assessment run results.
*/
public val resultEncryptionMode: kotlin.String? = builder.resultEncryptionMode
/**
* ARN of the KMS encryption key used to encrypt the assessment run results.
*/
public val resultKmsKeyArn: kotlin.String? = builder.resultKmsKeyArn
/**
* Amazon S3 bucket where DMS stores the results of this assessment run.
*/
public val resultLocationBucket: kotlin.String? = builder.resultLocationBucket
/**
* Folder in an Amazon S3 bucket where DMS stores the results of this assessment run.
*/
public val resultLocationFolder: kotlin.String? = builder.resultLocationFolder
/**
* Result statistics for a completed assessment run, showing aggregated statistics of IndividualAssessments for how many assessments were passed, failed, or encountered issues such as errors or warnings.
*/
public val resultStatistic: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationTaskAssessmentRunResultStatistic? = builder.resultStatistic
/**
* ARN of the service role used to start the assessment run using the `StartReplicationTaskAssessmentRun` operation. The role must allow the `iam:PassRole` action.
*/
public val serviceAccessRoleArn: kotlin.String? = builder.serviceAccessRoleArn
/**
* Assessment run status.
*
* This status can have one of the following values:
* + `"cancelling"` – The assessment run was canceled by the `CancelReplicationTaskAssessmentRun` operation.
* + `"deleting"` – The assessment run was deleted by the `DeleteReplicationTaskAssessmentRun` operation.
* + `"failed"` – At least one individual assessment completed with a `failed` status.
* + `"error-provisioning"` – An internal error occurred while resources were provisioned (during `provisioning` status).
* + `"error-executing"` – An internal error occurred while individual assessments ran (during `running` status).
* + `"invalid state"` – The assessment run is in an unknown state.
* + `"passed"` – All individual assessments have completed, and none has a `failed` status.
* + `"provisioning"` – Resources required to run individual assessments are being provisioned.
* + `"running"` – Individual assessments are being run.
* + `"starting"` – The assessment run is starting, but resources are not yet being provisioned for individual assessments.
* + `"warning"` – At least one individual assessment completed with a `warning` status.
*/
public val status: kotlin.String? = builder.status
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationTaskAssessmentRun = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ReplicationTaskAssessmentRun(")
append("assessmentProgress=$assessmentProgress,")
append("assessmentRunName=$assessmentRunName,")
append("isLatestTaskAssessmentRun=$isLatestTaskAssessmentRun,")
append("lastFailureMessage=$lastFailureMessage,")
append("replicationTaskArn=$replicationTaskArn,")
append("replicationTaskAssessmentRunArn=$replicationTaskAssessmentRunArn,")
append("replicationTaskAssessmentRunCreationDate=$replicationTaskAssessmentRunCreationDate,")
append("resultEncryptionMode=$resultEncryptionMode,")
append("resultKmsKeyArn=$resultKmsKeyArn,")
append("resultLocationBucket=$resultLocationBucket,")
append("resultLocationFolder=$resultLocationFolder,")
append("resultStatistic=$resultStatistic,")
append("serviceAccessRoleArn=$serviceAccessRoleArn,")
append("status=$status")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = assessmentProgress?.hashCode() ?: 0
result = 31 * result + (assessmentRunName?.hashCode() ?: 0)
result = 31 * result + (isLatestTaskAssessmentRun.hashCode())
result = 31 * result + (lastFailureMessage?.hashCode() ?: 0)
result = 31 * result + (replicationTaskArn?.hashCode() ?: 0)
result = 31 * result + (replicationTaskAssessmentRunArn?.hashCode() ?: 0)
result = 31 * result + (replicationTaskAssessmentRunCreationDate?.hashCode() ?: 0)
result = 31 * result + (resultEncryptionMode?.hashCode() ?: 0)
result = 31 * result + (resultKmsKeyArn?.hashCode() ?: 0)
result = 31 * result + (resultLocationBucket?.hashCode() ?: 0)
result = 31 * result + (resultLocationFolder?.hashCode() ?: 0)
result = 31 * result + (resultStatistic?.hashCode() ?: 0)
result = 31 * result + (serviceAccessRoleArn?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ReplicationTaskAssessmentRun
if (assessmentProgress != other.assessmentProgress) return false
if (assessmentRunName != other.assessmentRunName) return false
if (isLatestTaskAssessmentRun != other.isLatestTaskAssessmentRun) return false
if (lastFailureMessage != other.lastFailureMessage) return false
if (replicationTaskArn != other.replicationTaskArn) return false
if (replicationTaskAssessmentRunArn != other.replicationTaskAssessmentRunArn) return false
if (replicationTaskAssessmentRunCreationDate != other.replicationTaskAssessmentRunCreationDate) return false
if (resultEncryptionMode != other.resultEncryptionMode) return false
if (resultKmsKeyArn != other.resultKmsKeyArn) return false
if (resultLocationBucket != other.resultLocationBucket) return false
if (resultLocationFolder != other.resultLocationFolder) return false
if (resultStatistic != other.resultStatistic) return false
if (serviceAccessRoleArn != other.serviceAccessRoleArn) return false
if (status != other.status) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationTaskAssessmentRun = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Indication of the completion progress for the individual assessments specified to run.
*/
public var assessmentProgress: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationTaskAssessmentRunProgress? = null
/**
* Unique name of the assessment run.
*/
public var assessmentRunName: kotlin.String? = null
/**
* Indicates that the following PreflightAssessmentRun is the latest for the ReplicationTask. The status is either true or false.
*/
public var isLatestTaskAssessmentRun: kotlin.Boolean = false
/**
* Last message generated by an individual assessment failure.
*/
public var lastFailureMessage: kotlin.String? = null
/**
* ARN of the migration task associated with this premigration assessment run.
*/
public var replicationTaskArn: kotlin.String? = null
/**
* Amazon Resource Name (ARN) of this assessment run.
*/
public var replicationTaskAssessmentRunArn: kotlin.String? = null
/**
* Date on which the assessment run was created using the `StartReplicationTaskAssessmentRun` operation.
*/
public var replicationTaskAssessmentRunCreationDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Encryption mode used to encrypt the assessment run results.
*/
public var resultEncryptionMode: kotlin.String? = null
/**
* ARN of the KMS encryption key used to encrypt the assessment run results.
*/
public var resultKmsKeyArn: kotlin.String? = null
/**
* Amazon S3 bucket where DMS stores the results of this assessment run.
*/
public var resultLocationBucket: kotlin.String? = null
/**
* Folder in an Amazon S3 bucket where DMS stores the results of this assessment run.
*/
public var resultLocationFolder: kotlin.String? = null
/**
* Result statistics for a completed assessment run, showing aggregated statistics of IndividualAssessments for how many assessments were passed, failed, or encountered issues such as errors or warnings.
*/
public var resultStatistic: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationTaskAssessmentRunResultStatistic? = null
/**
* ARN of the service role used to start the assessment run using the `StartReplicationTaskAssessmentRun` operation. The role must allow the `iam:PassRole` action.
*/
public var serviceAccessRoleArn: kotlin.String? = null
/**
* Assessment run status.
*
* This status can have one of the following values:
* + `"cancelling"` – The assessment run was canceled by the `CancelReplicationTaskAssessmentRun` operation.
* + `"deleting"` – The assessment run was deleted by the `DeleteReplicationTaskAssessmentRun` operation.
* + `"failed"` – At least one individual assessment completed with a `failed` status.
* + `"error-provisioning"` – An internal error occurred while resources were provisioned (during `provisioning` status).
* + `"error-executing"` – An internal error occurred while individual assessments ran (during `running` status).
* + `"invalid state"` – The assessment run is in an unknown state.
* + `"passed"` – All individual assessments have completed, and none has a `failed` status.
* + `"provisioning"` – Resources required to run individual assessments are being provisioned.
* + `"running"` – Individual assessments are being run.
* + `"starting"` – The assessment run is starting, but resources are not yet being provisioned for individual assessments.
* + `"warning"` – At least one individual assessment completed with a `warning` status.
*/
public var status: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationTaskAssessmentRun) : this() {
this.assessmentProgress = x.assessmentProgress
this.assessmentRunName = x.assessmentRunName
this.isLatestTaskAssessmentRun = x.isLatestTaskAssessmentRun
this.lastFailureMessage = x.lastFailureMessage
this.replicationTaskArn = x.replicationTaskArn
this.replicationTaskAssessmentRunArn = x.replicationTaskAssessmentRunArn
this.replicationTaskAssessmentRunCreationDate = x.replicationTaskAssessmentRunCreationDate
this.resultEncryptionMode = x.resultEncryptionMode
this.resultKmsKeyArn = x.resultKmsKeyArn
this.resultLocationBucket = x.resultLocationBucket
this.resultLocationFolder = x.resultLocationFolder
this.resultStatistic = x.resultStatistic
this.serviceAccessRoleArn = x.serviceAccessRoleArn
this.status = x.status
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationTaskAssessmentRun = ReplicationTaskAssessmentRun(this)
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationTaskAssessmentRunProgress] inside the given [block]
*/
public fun assessmentProgress(block: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationTaskAssessmentRunProgress.Builder.() -> kotlin.Unit) {
this.assessmentProgress = aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationTaskAssessmentRunProgress.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationTaskAssessmentRunResultStatistic] inside the given [block]
*/
public fun resultStatistic(block: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationTaskAssessmentRunResultStatistic.Builder.() -> kotlin.Unit) {
this.resultStatistic = aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationTaskAssessmentRunResultStatistic.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy