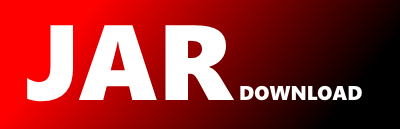
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.StartReplicationTaskAssessmentRunRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
*
*/
public class StartReplicationTaskAssessmentRunRequest private constructor(builder: Builder) {
/**
* Unique name to identify the assessment run.
*/
public val assessmentRunName: kotlin.String? = builder.assessmentRunName
/**
* Space-separated list of names for specific individual assessments that you want to exclude. These names come from the default list of individual assessments that DMS supports for the associated migration task. This task is specified by `ReplicationTaskArn`.
*
* You can't set a value for `Exclude` if you also set a value for `IncludeOnly` in the API operation.
*
* To identify the names of the default individual assessments that DMS supports for the associated migration task, run the `DescribeApplicableIndividualAssessments` operation using its own `ReplicationTaskArn` request parameter.
*/
public val exclude: List? = builder.exclude
/**
* Space-separated list of names for specific individual assessments that you want to include. These names come from the default list of individual assessments that DMS supports for the associated migration task. This task is specified by `ReplicationTaskArn`.
*
* You can't set a value for `IncludeOnly` if you also set a value for `Exclude` in the API operation.
*
* To identify the names of the default individual assessments that DMS supports for the associated migration task, run the `DescribeApplicableIndividualAssessments` operation using its own `ReplicationTaskArn` request parameter.
*/
public val includeOnly: List? = builder.includeOnly
/**
* Amazon Resource Name (ARN) of the migration task associated with the premigration assessment run that you want to start.
*/
public val replicationTaskArn: kotlin.String? = builder.replicationTaskArn
/**
* Encryption mode that you can specify to encrypt the results of this assessment run. If you don't specify this request parameter, DMS stores the assessment run results without encryption. You can specify one of the options following:
* + `"SSE_S3"` – The server-side encryption provided as a default by Amazon S3.
* + `"SSE_KMS"` – Key Management Service (KMS) encryption. This encryption can use either a custom KMS encryption key that you specify or the default KMS encryption key that DMS provides.
*/
public val resultEncryptionMode: kotlin.String? = builder.resultEncryptionMode
/**
* ARN of a custom KMS encryption key that you specify when you set `ResultEncryptionMode` to `"SSE_KMS`".
*/
public val resultKmsKeyArn: kotlin.String? = builder.resultKmsKeyArn
/**
* Amazon S3 bucket where you want DMS to store the results of this assessment run.
*/
public val resultLocationBucket: kotlin.String? = builder.resultLocationBucket
/**
* Folder within an Amazon S3 bucket where you want DMS to store the results of this assessment run.
*/
public val resultLocationFolder: kotlin.String? = builder.resultLocationFolder
/**
* ARN of the service role needed to start the assessment run. The role must allow the `iam:PassRole` action.
*/
public val serviceAccessRoleArn: kotlin.String? = builder.serviceAccessRoleArn
/**
* One or more tags to be assigned to the premigration assessment run that you want to start.
*/
public val tags: List? = builder.tags
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.StartReplicationTaskAssessmentRunRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("StartReplicationTaskAssessmentRunRequest(")
append("assessmentRunName=$assessmentRunName,")
append("exclude=$exclude,")
append("includeOnly=$includeOnly,")
append("replicationTaskArn=$replicationTaskArn,")
append("resultEncryptionMode=$resultEncryptionMode,")
append("resultKmsKeyArn=$resultKmsKeyArn,")
append("resultLocationBucket=$resultLocationBucket,")
append("resultLocationFolder=$resultLocationFolder,")
append("serviceAccessRoleArn=$serviceAccessRoleArn,")
append("tags=$tags")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = assessmentRunName?.hashCode() ?: 0
result = 31 * result + (exclude?.hashCode() ?: 0)
result = 31 * result + (includeOnly?.hashCode() ?: 0)
result = 31 * result + (replicationTaskArn?.hashCode() ?: 0)
result = 31 * result + (resultEncryptionMode?.hashCode() ?: 0)
result = 31 * result + (resultKmsKeyArn?.hashCode() ?: 0)
result = 31 * result + (resultLocationBucket?.hashCode() ?: 0)
result = 31 * result + (resultLocationFolder?.hashCode() ?: 0)
result = 31 * result + (serviceAccessRoleArn?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as StartReplicationTaskAssessmentRunRequest
if (assessmentRunName != other.assessmentRunName) return false
if (exclude != other.exclude) return false
if (includeOnly != other.includeOnly) return false
if (replicationTaskArn != other.replicationTaskArn) return false
if (resultEncryptionMode != other.resultEncryptionMode) return false
if (resultKmsKeyArn != other.resultKmsKeyArn) return false
if (resultLocationBucket != other.resultLocationBucket) return false
if (resultLocationFolder != other.resultLocationFolder) return false
if (serviceAccessRoleArn != other.serviceAccessRoleArn) return false
if (tags != other.tags) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.StartReplicationTaskAssessmentRunRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Unique name to identify the assessment run.
*/
public var assessmentRunName: kotlin.String? = null
/**
* Space-separated list of names for specific individual assessments that you want to exclude. These names come from the default list of individual assessments that DMS supports for the associated migration task. This task is specified by `ReplicationTaskArn`.
*
* You can't set a value for `Exclude` if you also set a value for `IncludeOnly` in the API operation.
*
* To identify the names of the default individual assessments that DMS supports for the associated migration task, run the `DescribeApplicableIndividualAssessments` operation using its own `ReplicationTaskArn` request parameter.
*/
public var exclude: List? = null
/**
* Space-separated list of names for specific individual assessments that you want to include. These names come from the default list of individual assessments that DMS supports for the associated migration task. This task is specified by `ReplicationTaskArn`.
*
* You can't set a value for `IncludeOnly` if you also set a value for `Exclude` in the API operation.
*
* To identify the names of the default individual assessments that DMS supports for the associated migration task, run the `DescribeApplicableIndividualAssessments` operation using its own `ReplicationTaskArn` request parameter.
*/
public var includeOnly: List? = null
/**
* Amazon Resource Name (ARN) of the migration task associated with the premigration assessment run that you want to start.
*/
public var replicationTaskArn: kotlin.String? = null
/**
* Encryption mode that you can specify to encrypt the results of this assessment run. If you don't specify this request parameter, DMS stores the assessment run results without encryption. You can specify one of the options following:
* + `"SSE_S3"` – The server-side encryption provided as a default by Amazon S3.
* + `"SSE_KMS"` – Key Management Service (KMS) encryption. This encryption can use either a custom KMS encryption key that you specify or the default KMS encryption key that DMS provides.
*/
public var resultEncryptionMode: kotlin.String? = null
/**
* ARN of a custom KMS encryption key that you specify when you set `ResultEncryptionMode` to `"SSE_KMS`".
*/
public var resultKmsKeyArn: kotlin.String? = null
/**
* Amazon S3 bucket where you want DMS to store the results of this assessment run.
*/
public var resultLocationBucket: kotlin.String? = null
/**
* Folder within an Amazon S3 bucket where you want DMS to store the results of this assessment run.
*/
public var resultLocationFolder: kotlin.String? = null
/**
* ARN of the service role needed to start the assessment run. The role must allow the `iam:PassRole` action.
*/
public var serviceAccessRoleArn: kotlin.String? = null
/**
* One or more tags to be assigned to the premigration assessment run that you want to start.
*/
public var tags: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.StartReplicationTaskAssessmentRunRequest) : this() {
this.assessmentRunName = x.assessmentRunName
this.exclude = x.exclude
this.includeOnly = x.includeOnly
this.replicationTaskArn = x.replicationTaskArn
this.resultEncryptionMode = x.resultEncryptionMode
this.resultKmsKeyArn = x.resultKmsKeyArn
this.resultLocationBucket = x.resultLocationBucket
this.resultLocationFolder = x.resultLocationFolder
this.serviceAccessRoleArn = x.serviceAccessRoleArn
this.tags = x.tags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.StartReplicationTaskAssessmentRunRequest = StartReplicationTaskAssessmentRunRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy