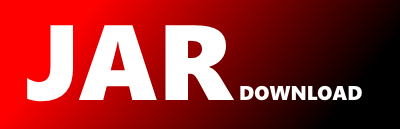
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.TableStatistics.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Provides a collection of table statistics in response to a request by the `DescribeTableStatistics` operation.
*/
public class TableStatistics private constructor(builder: Builder) {
/**
* The number of data definition language (DDL) statements used to build and modify the structure of your tables applied on the target.
*/
public val appliedDdls: kotlin.Long? = builder.appliedDdls
/**
* The number of delete actions applied on a target table.
*/
public val appliedDeletes: kotlin.Long? = builder.appliedDeletes
/**
* The number of insert actions applied on a target table.
*/
public val appliedInserts: kotlin.Long? = builder.appliedInserts
/**
* The number of update actions applied on a target table.
*/
public val appliedUpdates: kotlin.Long? = builder.appliedUpdates
/**
* The data definition language (DDL) used to build and modify the structure of your tables.
*/
public val ddls: kotlin.Long = builder.ddls
/**
* The number of delete actions performed on a table.
*/
public val deletes: kotlin.Long = builder.deletes
/**
* The number of rows that failed conditional checks during the full load operation (valid only for migrations where DynamoDB is the target).
*/
public val fullLoadCondtnlChkFailedRows: kotlin.Long = builder.fullLoadCondtnlChkFailedRows
/**
* The time when the full load operation completed.
*/
public val fullLoadEndTime: aws.smithy.kotlin.runtime.time.Instant? = builder.fullLoadEndTime
/**
* The number of rows that failed to load during the full load operation (valid only for migrations where DynamoDB is the target).
*/
public val fullLoadErrorRows: kotlin.Long = builder.fullLoadErrorRows
/**
* A value that indicates if the table was reloaded (`true`) or loaded as part of a new full load operation (`false`).
*/
public val fullLoadReloaded: kotlin.Boolean? = builder.fullLoadReloaded
/**
* The number of rows added during the full load operation.
*/
public val fullLoadRows: kotlin.Long = builder.fullLoadRows
/**
* The time when the full load operation started.
*/
public val fullLoadStartTime: aws.smithy.kotlin.runtime.time.Instant? = builder.fullLoadStartTime
/**
* The number of insert actions performed on a table.
*/
public val inserts: kotlin.Long = builder.inserts
/**
* The last time a table was updated.
*/
public val lastUpdateTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastUpdateTime
/**
* The schema name.
*/
public val schemaName: kotlin.String? = builder.schemaName
/**
* The name of the table.
*/
public val tableName: kotlin.String? = builder.tableName
/**
* The state of the tables described.
*
* Valid states: Table does not exist | Before load | Full load | Table completed | Table cancelled | Table error | Table is being reloaded
*/
public val tableState: kotlin.String? = builder.tableState
/**
* The number of update actions performed on a table.
*/
public val updates: kotlin.Long = builder.updates
/**
* The number of records that failed validation.
*/
public val validationFailedRecords: kotlin.Long = builder.validationFailedRecords
/**
* The number of records that have yet to be validated.
*/
public val validationPendingRecords: kotlin.Long = builder.validationPendingRecords
/**
* The validation state of the table.
*
* This parameter can have the following values:
* + Not enabled – Validation isn't enabled for the table in the migration task.
* + Pending records – Some records in the table are waiting for validation.
* + Mismatched records – Some records in the table don't match between the source and target.
* + Suspended records – Some records in the table couldn't be validated.
* + No primary key –The table couldn't be validated because it has no primary key.
* + Table error – The table wasn't validated because it's in an error state and some data wasn't migrated.
* + Validated – All rows in the table are validated. If the table is updated, the status can change from Validated.
* + Error – The table couldn't be validated because of an unexpected error.
* + Pending validation – The table is waiting validation.
* + Preparing table – Preparing the table enabled in the migration task for validation.
* + Pending revalidation – All rows in the table are pending validation after the table was updated.
*/
public val validationState: kotlin.String? = builder.validationState
/**
* Additional details about the state of validation.
*/
public val validationStateDetails: kotlin.String? = builder.validationStateDetails
/**
* The number of records that couldn't be validated.
*/
public val validationSuspendedRecords: kotlin.Long = builder.validationSuspendedRecords
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.TableStatistics = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("TableStatistics(")
append("appliedDdls=$appliedDdls,")
append("appliedDeletes=$appliedDeletes,")
append("appliedInserts=$appliedInserts,")
append("appliedUpdates=$appliedUpdates,")
append("ddls=$ddls,")
append("deletes=$deletes,")
append("fullLoadCondtnlChkFailedRows=$fullLoadCondtnlChkFailedRows,")
append("fullLoadEndTime=$fullLoadEndTime,")
append("fullLoadErrorRows=$fullLoadErrorRows,")
append("fullLoadReloaded=$fullLoadReloaded,")
append("fullLoadRows=$fullLoadRows,")
append("fullLoadStartTime=$fullLoadStartTime,")
append("inserts=$inserts,")
append("lastUpdateTime=$lastUpdateTime,")
append("schemaName=$schemaName,")
append("tableName=$tableName,")
append("tableState=$tableState,")
append("updates=$updates,")
append("validationFailedRecords=$validationFailedRecords,")
append("validationPendingRecords=$validationPendingRecords,")
append("validationState=$validationState,")
append("validationStateDetails=$validationStateDetails,")
append("validationSuspendedRecords=$validationSuspendedRecords")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = appliedDdls?.hashCode() ?: 0
result = 31 * result + (appliedDeletes?.hashCode() ?: 0)
result = 31 * result + (appliedInserts?.hashCode() ?: 0)
result = 31 * result + (appliedUpdates?.hashCode() ?: 0)
result = 31 * result + (ddls.hashCode())
result = 31 * result + (deletes.hashCode())
result = 31 * result + (fullLoadCondtnlChkFailedRows.hashCode())
result = 31 * result + (fullLoadEndTime?.hashCode() ?: 0)
result = 31 * result + (fullLoadErrorRows.hashCode())
result = 31 * result + (fullLoadReloaded?.hashCode() ?: 0)
result = 31 * result + (fullLoadRows.hashCode())
result = 31 * result + (fullLoadStartTime?.hashCode() ?: 0)
result = 31 * result + (inserts.hashCode())
result = 31 * result + (lastUpdateTime?.hashCode() ?: 0)
result = 31 * result + (schemaName?.hashCode() ?: 0)
result = 31 * result + (tableName?.hashCode() ?: 0)
result = 31 * result + (tableState?.hashCode() ?: 0)
result = 31 * result + (updates.hashCode())
result = 31 * result + (validationFailedRecords.hashCode())
result = 31 * result + (validationPendingRecords.hashCode())
result = 31 * result + (validationState?.hashCode() ?: 0)
result = 31 * result + (validationStateDetails?.hashCode() ?: 0)
result = 31 * result + (validationSuspendedRecords.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as TableStatistics
if (appliedDdls != other.appliedDdls) return false
if (appliedDeletes != other.appliedDeletes) return false
if (appliedInserts != other.appliedInserts) return false
if (appliedUpdates != other.appliedUpdates) return false
if (ddls != other.ddls) return false
if (deletes != other.deletes) return false
if (fullLoadCondtnlChkFailedRows != other.fullLoadCondtnlChkFailedRows) return false
if (fullLoadEndTime != other.fullLoadEndTime) return false
if (fullLoadErrorRows != other.fullLoadErrorRows) return false
if (fullLoadReloaded != other.fullLoadReloaded) return false
if (fullLoadRows != other.fullLoadRows) return false
if (fullLoadStartTime != other.fullLoadStartTime) return false
if (inserts != other.inserts) return false
if (lastUpdateTime != other.lastUpdateTime) return false
if (schemaName != other.schemaName) return false
if (tableName != other.tableName) return false
if (tableState != other.tableState) return false
if (updates != other.updates) return false
if (validationFailedRecords != other.validationFailedRecords) return false
if (validationPendingRecords != other.validationPendingRecords) return false
if (validationState != other.validationState) return false
if (validationStateDetails != other.validationStateDetails) return false
if (validationSuspendedRecords != other.validationSuspendedRecords) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.TableStatistics = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The number of data definition language (DDL) statements used to build and modify the structure of your tables applied on the target.
*/
public var appliedDdls: kotlin.Long? = null
/**
* The number of delete actions applied on a target table.
*/
public var appliedDeletes: kotlin.Long? = null
/**
* The number of insert actions applied on a target table.
*/
public var appliedInserts: kotlin.Long? = null
/**
* The number of update actions applied on a target table.
*/
public var appliedUpdates: kotlin.Long? = null
/**
* The data definition language (DDL) used to build and modify the structure of your tables.
*/
public var ddls: kotlin.Long = 0L
/**
* The number of delete actions performed on a table.
*/
public var deletes: kotlin.Long = 0L
/**
* The number of rows that failed conditional checks during the full load operation (valid only for migrations where DynamoDB is the target).
*/
public var fullLoadCondtnlChkFailedRows: kotlin.Long = 0L
/**
* The time when the full load operation completed.
*/
public var fullLoadEndTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The number of rows that failed to load during the full load operation (valid only for migrations where DynamoDB is the target).
*/
public var fullLoadErrorRows: kotlin.Long = 0L
/**
* A value that indicates if the table was reloaded (`true`) or loaded as part of a new full load operation (`false`).
*/
public var fullLoadReloaded: kotlin.Boolean? = null
/**
* The number of rows added during the full load operation.
*/
public var fullLoadRows: kotlin.Long = 0L
/**
* The time when the full load operation started.
*/
public var fullLoadStartTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The number of insert actions performed on a table.
*/
public var inserts: kotlin.Long = 0L
/**
* The last time a table was updated.
*/
public var lastUpdateTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The schema name.
*/
public var schemaName: kotlin.String? = null
/**
* The name of the table.
*/
public var tableName: kotlin.String? = null
/**
* The state of the tables described.
*
* Valid states: Table does not exist | Before load | Full load | Table completed | Table cancelled | Table error | Table is being reloaded
*/
public var tableState: kotlin.String? = null
/**
* The number of update actions performed on a table.
*/
public var updates: kotlin.Long = 0L
/**
* The number of records that failed validation.
*/
public var validationFailedRecords: kotlin.Long = 0L
/**
* The number of records that have yet to be validated.
*/
public var validationPendingRecords: kotlin.Long = 0L
/**
* The validation state of the table.
*
* This parameter can have the following values:
* + Not enabled – Validation isn't enabled for the table in the migration task.
* + Pending records – Some records in the table are waiting for validation.
* + Mismatched records – Some records in the table don't match between the source and target.
* + Suspended records – Some records in the table couldn't be validated.
* + No primary key –The table couldn't be validated because it has no primary key.
* + Table error – The table wasn't validated because it's in an error state and some data wasn't migrated.
* + Validated – All rows in the table are validated. If the table is updated, the status can change from Validated.
* + Error – The table couldn't be validated because of an unexpected error.
* + Pending validation – The table is waiting validation.
* + Preparing table – Preparing the table enabled in the migration task for validation.
* + Pending revalidation – All rows in the table are pending validation after the table was updated.
*/
public var validationState: kotlin.String? = null
/**
* Additional details about the state of validation.
*/
public var validationStateDetails: kotlin.String? = null
/**
* The number of records that couldn't be validated.
*/
public var validationSuspendedRecords: kotlin.Long = 0L
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.TableStatistics) : this() {
this.appliedDdls = x.appliedDdls
this.appliedDeletes = x.appliedDeletes
this.appliedInserts = x.appliedInserts
this.appliedUpdates = x.appliedUpdates
this.ddls = x.ddls
this.deletes = x.deletes
this.fullLoadCondtnlChkFailedRows = x.fullLoadCondtnlChkFailedRows
this.fullLoadEndTime = x.fullLoadEndTime
this.fullLoadErrorRows = x.fullLoadErrorRows
this.fullLoadReloaded = x.fullLoadReloaded
this.fullLoadRows = x.fullLoadRows
this.fullLoadStartTime = x.fullLoadStartTime
this.inserts = x.inserts
this.lastUpdateTime = x.lastUpdateTime
this.schemaName = x.schemaName
this.tableName = x.tableName
this.tableState = x.tableState
this.updates = x.updates
this.validationFailedRecords = x.validationFailedRecords
this.validationPendingRecords = x.validationPendingRecords
this.validationState = x.validationState
this.validationStateDetails = x.validationStateDetails
this.validationSuspendedRecords = x.validationSuspendedRecords
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.TableStatistics = TableStatistics(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy