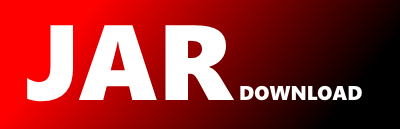
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.TimestreamSettings.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Provides information that defines an Amazon Timestream endpoint.
*/
public class TimestreamSettings private constructor(builder: Builder) {
/**
* Set this attribute to `true` to specify that DMS only applies inserts and updates, and not deletes. Amazon Timestream does not allow deleting records, so if this value is `false`, DMS nulls out the corresponding record in the Timestream database rather than deleting it.
*/
public val cdcInsertsAndUpdates: kotlin.Boolean? = builder.cdcInsertsAndUpdates
/**
* Database name for the endpoint.
*/
public val databaseName: kotlin.String = requireNotNull(builder.databaseName) { "A non-null value must be provided for databaseName" }
/**
* Set this attribute to `true` to enable memory store writes. When this value is `false`, DMS does not write records that are older in days than the value specified in `MagneticDuration`, because Amazon Timestream does not allow memory writes by default. For more information, see [Storage](https://docs.aws.amazon.com/timestream/latest/developerguide/storage.html) in the [Amazon Timestream Developer Guide](https://docs.aws.amazon.com/timestream/latest/developerguide/).
*/
public val enableMagneticStoreWrites: kotlin.Boolean? = builder.enableMagneticStoreWrites
/**
* Set this attribute to specify the default magnetic duration applied to the Amazon Timestream tables in days. This is the number of days that records remain in magnetic store before being discarded. For more information, see [Storage](https://docs.aws.amazon.com/timestream/latest/developerguide/storage.html) in the [Amazon Timestream Developer Guide](https://docs.aws.amazon.com/timestream/latest/developerguide/).
*/
public val magneticDuration: kotlin.Int = requireNotNull(builder.magneticDuration) { "A non-null value must be provided for magneticDuration" }
/**
* Set this attribute to specify the length of time to store all of the tables in memory that are migrated into Amazon Timestream from the source database. Time is measured in units of hours. When Timestream data comes in, it first resides in memory for the specified duration, which allows quick access to it.
*/
public val memoryDuration: kotlin.Int = requireNotNull(builder.memoryDuration) { "A non-null value must be provided for memoryDuration" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.TimestreamSettings = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("TimestreamSettings(")
append("cdcInsertsAndUpdates=$cdcInsertsAndUpdates,")
append("databaseName=$databaseName,")
append("enableMagneticStoreWrites=$enableMagneticStoreWrites,")
append("magneticDuration=$magneticDuration,")
append("memoryDuration=$memoryDuration")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = cdcInsertsAndUpdates?.hashCode() ?: 0
result = 31 * result + (databaseName.hashCode())
result = 31 * result + (enableMagneticStoreWrites?.hashCode() ?: 0)
result = 31 * result + (magneticDuration)
result = 31 * result + (memoryDuration)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as TimestreamSettings
if (cdcInsertsAndUpdates != other.cdcInsertsAndUpdates) return false
if (databaseName != other.databaseName) return false
if (enableMagneticStoreWrites != other.enableMagneticStoreWrites) return false
if (magneticDuration != other.magneticDuration) return false
if (memoryDuration != other.memoryDuration) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.TimestreamSettings = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Set this attribute to `true` to specify that DMS only applies inserts and updates, and not deletes. Amazon Timestream does not allow deleting records, so if this value is `false`, DMS nulls out the corresponding record in the Timestream database rather than deleting it.
*/
public var cdcInsertsAndUpdates: kotlin.Boolean? = null
/**
* Database name for the endpoint.
*/
public var databaseName: kotlin.String? = null
/**
* Set this attribute to `true` to enable memory store writes. When this value is `false`, DMS does not write records that are older in days than the value specified in `MagneticDuration`, because Amazon Timestream does not allow memory writes by default. For more information, see [Storage](https://docs.aws.amazon.com/timestream/latest/developerguide/storage.html) in the [Amazon Timestream Developer Guide](https://docs.aws.amazon.com/timestream/latest/developerguide/).
*/
public var enableMagneticStoreWrites: kotlin.Boolean? = null
/**
* Set this attribute to specify the default magnetic duration applied to the Amazon Timestream tables in days. This is the number of days that records remain in magnetic store before being discarded. For more information, see [Storage](https://docs.aws.amazon.com/timestream/latest/developerguide/storage.html) in the [Amazon Timestream Developer Guide](https://docs.aws.amazon.com/timestream/latest/developerguide/).
*/
public var magneticDuration: kotlin.Int? = null
/**
* Set this attribute to specify the length of time to store all of the tables in memory that are migrated into Amazon Timestream from the source database. Time is measured in units of hours. When Timestream data comes in, it first resides in memory for the specified duration, which allows quick access to it.
*/
public var memoryDuration: kotlin.Int? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.TimestreamSettings) : this() {
this.cdcInsertsAndUpdates = x.cdcInsertsAndUpdates
this.databaseName = x.databaseName
this.enableMagneticStoreWrites = x.enableMagneticStoreWrites
this.magneticDuration = x.magneticDuration
this.memoryDuration = x.memoryDuration
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.TimestreamSettings = TimestreamSettings(this)
internal fun correctErrors(): Builder {
if (databaseName == null) databaseName = ""
if (magneticDuration == null) magneticDuration = 0
if (memoryDuration == null) memoryDuration = 0
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy