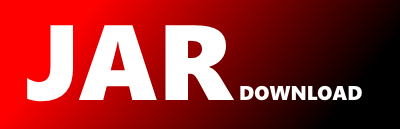
commonMain.aws.sdk.kotlin.services.databrew.model.Rule.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databrew-jvm Show documentation
Show all versions of databrew-jvm Show documentation
The AWS Kotlin client for DataBrew
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databrew.model
/**
* Represents a single data quality requirement that should be validated in the scope of this dataset.
*/
public class Rule private constructor(builder: Builder) {
/**
* The expression which includes column references, condition names followed by variable references, possibly grouped and combined with other conditions. For example, `(:col1 starts_with :prefix1 or :col1 starts_with :prefix2) and (:col1 ends_with :suffix1 or :col1 ends_with :suffix2)`. Column and value references are substitution variables that should start with the ':' symbol. Depending on the context, substitution variables' values can be either an actual value or a column name. These values are defined in the SubstitutionMap. If a CheckExpression starts with a column reference, then ColumnSelectors in the rule should be null. If ColumnSelectors has been defined, then there should be no column reference in the left side of a condition, for example, `is_between :val1 and :val2`.
*
* For more information, see [Available checks](https://docs.aws.amazon.com/databrew/latest/dg/profile.data-quality-available-checks.html)
*/
public val checkExpression: kotlin.String? = builder.checkExpression
/**
* List of column selectors. Selectors can be used to select columns using a name or regular expression from the dataset. Rule will be applied to selected columns.
*/
public val columnSelectors: List? = builder.columnSelectors
/**
* A value that specifies whether the rule is disabled. Once a rule is disabled, a profile job will not validate it during a job run. Default value is false.
*/
public val disabled: kotlin.Boolean = builder.disabled
/**
* The name of the rule.
*/
public val name: kotlin.String? = builder.name
/**
* The map of substitution variable names to their values used in a check expression. Variable names should start with a ':' (colon). Variable values can either be actual values or column names. To differentiate between the two, column names should be enclosed in backticks, for example, `":col1": "`Column A`".`
*/
public val substitutionMap: Map? = builder.substitutionMap
/**
* The threshold used with a non-aggregate check expression. Non-aggregate check expressions will be applied to each row in a specific column, and the threshold will be used to determine whether the validation succeeds.
*/
public val threshold: aws.sdk.kotlin.services.databrew.model.Threshold? = builder.threshold
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databrew.model.Rule = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Rule(")
append("checkExpression=$checkExpression,")
append("columnSelectors=$columnSelectors,")
append("disabled=$disabled,")
append("name=$name,")
append("substitutionMap=$substitutionMap,")
append("threshold=$threshold)")
}
override fun hashCode(): kotlin.Int {
var result = checkExpression?.hashCode() ?: 0
result = 31 * result + (columnSelectors?.hashCode() ?: 0)
result = 31 * result + (disabled.hashCode())
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (substitutionMap?.hashCode() ?: 0)
result = 31 * result + (threshold?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Rule
if (checkExpression != other.checkExpression) return false
if (columnSelectors != other.columnSelectors) return false
if (disabled != other.disabled) return false
if (name != other.name) return false
if (substitutionMap != other.substitutionMap) return false
if (threshold != other.threshold) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databrew.model.Rule = Builder(this).apply(block).build()
public class Builder {
/**
* The expression which includes column references, condition names followed by variable references, possibly grouped and combined with other conditions. For example, `(:col1 starts_with :prefix1 or :col1 starts_with :prefix2) and (:col1 ends_with :suffix1 or :col1 ends_with :suffix2)`. Column and value references are substitution variables that should start with the ':' symbol. Depending on the context, substitution variables' values can be either an actual value or a column name. These values are defined in the SubstitutionMap. If a CheckExpression starts with a column reference, then ColumnSelectors in the rule should be null. If ColumnSelectors has been defined, then there should be no column reference in the left side of a condition, for example, `is_between :val1 and :val2`.
*
* For more information, see [Available checks](https://docs.aws.amazon.com/databrew/latest/dg/profile.data-quality-available-checks.html)
*/
public var checkExpression: kotlin.String? = null
/**
* List of column selectors. Selectors can be used to select columns using a name or regular expression from the dataset. Rule will be applied to selected columns.
*/
public var columnSelectors: List? = null
/**
* A value that specifies whether the rule is disabled. Once a rule is disabled, a profile job will not validate it during a job run. Default value is false.
*/
public var disabled: kotlin.Boolean = false
/**
* The name of the rule.
*/
public var name: kotlin.String? = null
/**
* The map of substitution variable names to their values used in a check expression. Variable names should start with a ':' (colon). Variable values can either be actual values or column names. To differentiate between the two, column names should be enclosed in backticks, for example, `":col1": "`Column A`".`
*/
public var substitutionMap: Map? = null
/**
* The threshold used with a non-aggregate check expression. Non-aggregate check expressions will be applied to each row in a specific column, and the threshold will be used to determine whether the validation succeeds.
*/
public var threshold: aws.sdk.kotlin.services.databrew.model.Threshold? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databrew.model.Rule) : this() {
this.checkExpression = x.checkExpression
this.columnSelectors = x.columnSelectors
this.disabled = x.disabled
this.name = x.name
this.substitutionMap = x.substitutionMap
this.threshold = x.threshold
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databrew.model.Rule = Rule(this)
/**
* construct an [aws.sdk.kotlin.services.databrew.model.Threshold] inside the given [block]
*/
public fun threshold(block: aws.sdk.kotlin.services.databrew.model.Threshold.Builder.() -> kotlin.Unit) {
this.threshold = aws.sdk.kotlin.services.databrew.model.Threshold.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy