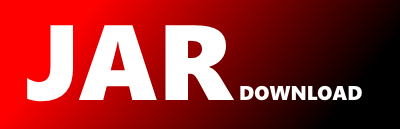
commonMain.aws.sdk.kotlin.services.databrew.DefaultDataBrewClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databrew-jvm Show documentation
Show all versions of databrew-jvm Show documentation
The AWS Kotlin client for DataBrew
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databrew
import aws.sdk.kotlin.runtime.client.AwsClientOption
import aws.sdk.kotlin.runtime.http.ApiMetadata
import aws.sdk.kotlin.runtime.http.AwsUserAgentMetadata
import aws.sdk.kotlin.runtime.http.interceptors.AwsSpanInterceptor
import aws.sdk.kotlin.runtime.http.middleware.AwsRetryHeaderMiddleware
import aws.sdk.kotlin.runtime.http.middleware.RecursionDetection
import aws.sdk.kotlin.runtime.http.middleware.UserAgent
import aws.sdk.kotlin.services.databrew.auth.DataBrewAuthSchemeProviderAdapter
import aws.sdk.kotlin.services.databrew.auth.DataBrewIdentityProviderConfigAdapter
import aws.sdk.kotlin.services.databrew.endpoints.internal.EndpointResolverAdapter
import aws.sdk.kotlin.services.databrew.model.*
import aws.sdk.kotlin.services.databrew.serde.*
import aws.smithy.kotlin.runtime.auth.AuthSchemeId
import aws.smithy.kotlin.runtime.auth.awssigning.AwsSigningAttributes
import aws.smithy.kotlin.runtime.auth.awssigning.DefaultAwsSigner
import aws.smithy.kotlin.runtime.client.SdkClientOption
import aws.smithy.kotlin.runtime.collections.attributesOf
import aws.smithy.kotlin.runtime.collections.putIfAbsent
import aws.smithy.kotlin.runtime.collections.putIfAbsentNotNull
import aws.smithy.kotlin.runtime.http.SdkHttpClient
import aws.smithy.kotlin.runtime.http.auth.AuthScheme
import aws.smithy.kotlin.runtime.http.auth.SigV4AuthScheme
import aws.smithy.kotlin.runtime.http.operation.OperationAuthConfig
import aws.smithy.kotlin.runtime.http.operation.OperationMetrics
import aws.smithy.kotlin.runtime.http.operation.SdkHttpOperation
import aws.smithy.kotlin.runtime.http.operation.context
import aws.smithy.kotlin.runtime.http.operation.roundTrip
import aws.smithy.kotlin.runtime.http.operation.telemetry
import aws.smithy.kotlin.runtime.io.SdkManagedGroup
import aws.smithy.kotlin.runtime.io.addIfManaged
import aws.smithy.kotlin.runtime.operation.ExecutionContext
public const val ServiceApiVersion: String = "2017-07-25"
internal class DefaultDataBrewClient(override val config: DataBrewClient.Config) : DataBrewClient {
private val managedResources = SdkManagedGroup()
private val client = SdkHttpClient(config.httpClient)
private val identityProviderConfig = DataBrewIdentityProviderConfigAdapter(config)
private val configuredAuthSchemes = with(config.authSchemes.associateBy(AuthScheme::schemeId).toMutableMap()){
getOrPut(AuthSchemeId.AwsSigV4){
SigV4AuthScheme(DefaultAwsSigner, "databrew")
}
toMap()
}
private val authSchemeAdapter = DataBrewAuthSchemeProviderAdapter(config)
private val telemetryScope = "aws.sdk.kotlin.services.databrew"
private val opMetrics = OperationMetrics(telemetryScope, config.telemetryProvider)
init {
managedResources.addIfManaged(config.httpClient)
managedResources.addIfManaged(config.credentialsProvider)
}
private val awsUserAgentMetadata = AwsUserAgentMetadata.fromEnvironment(ApiMetadata(ServiceId, SdkVersion), config.applicationId)
/**
* Deletes one or more versions of a recipe at a time.
*
* The entire request will be rejected if:
* + The recipe does not exist.
* + There is an invalid version identifier in the list of versions.
* + The version list is empty.
* + The version list size exceeds 50.
* + The version list contains duplicate entries.
*
* The request will complete successfully, but with partial failures, if:
* + A version does not exist.
* + A version is being used by a job.
* + You specify `LATEST_WORKING`, but it's being used by a project.
* + The version fails to be deleted.
*
* The `LATEST_WORKING` version will only be deleted if the recipe has no other versions. If you try to delete `LATEST_WORKING` while other versions exist (or if they can't be deleted), then `LATEST_WORKING` will be listed as partial failure in the response.
*/
override suspend fun batchDeleteRecipeVersion(input: BatchDeleteRecipeVersionRequest): BatchDeleteRecipeVersionResponse {
val op = SdkHttpOperation.build {
serializer = BatchDeleteRecipeVersionOperationSerializer()
deserializer = BatchDeleteRecipeVersionOperationDeserializer()
operationName = "BatchDeleteRecipeVersion"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a new DataBrew dataset.
*/
override suspend fun createDataset(input: CreateDatasetRequest): CreateDatasetResponse {
val op = SdkHttpOperation.build {
serializer = CreateDatasetOperationSerializer()
deserializer = CreateDatasetOperationDeserializer()
operationName = "CreateDataset"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a new job to analyze a dataset and create its data profile.
*/
override suspend fun createProfileJob(input: CreateProfileJobRequest): CreateProfileJobResponse {
val op = SdkHttpOperation.build {
serializer = CreateProfileJobOperationSerializer()
deserializer = CreateProfileJobOperationDeserializer()
operationName = "CreateProfileJob"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a new DataBrew project.
*/
override suspend fun createProject(input: CreateProjectRequest): CreateProjectResponse {
val op = SdkHttpOperation.build {
serializer = CreateProjectOperationSerializer()
deserializer = CreateProjectOperationDeserializer()
operationName = "CreateProject"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a new DataBrew recipe.
*/
override suspend fun createRecipe(input: CreateRecipeRequest): CreateRecipeResponse {
val op = SdkHttpOperation.build {
serializer = CreateRecipeOperationSerializer()
deserializer = CreateRecipeOperationDeserializer()
operationName = "CreateRecipe"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a new job to transform input data, using steps defined in an existing Glue DataBrew recipe
*/
override suspend fun createRecipeJob(input: CreateRecipeJobRequest): CreateRecipeJobResponse {
val op = SdkHttpOperation.build {
serializer = CreateRecipeJobOperationSerializer()
deserializer = CreateRecipeJobOperationDeserializer()
operationName = "CreateRecipeJob"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a new ruleset that can be used in a profile job to validate the data quality of a dataset.
*/
override suspend fun createRuleset(input: CreateRulesetRequest): CreateRulesetResponse {
val op = SdkHttpOperation.build {
serializer = CreateRulesetOperationSerializer()
deserializer = CreateRulesetOperationDeserializer()
operationName = "CreateRuleset"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a new schedule for one or more DataBrew jobs. Jobs can be run at a specific date and time, or at regular intervals.
*/
override suspend fun createSchedule(input: CreateScheduleRequest): CreateScheduleResponse {
val op = SdkHttpOperation.build {
serializer = CreateScheduleOperationSerializer()
deserializer = CreateScheduleOperationDeserializer()
operationName = "CreateSchedule"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes a dataset from DataBrew.
*/
override suspend fun deleteDataset(input: DeleteDatasetRequest): DeleteDatasetResponse {
val op = SdkHttpOperation.build {
serializer = DeleteDatasetOperationSerializer()
deserializer = DeleteDatasetOperationDeserializer()
operationName = "DeleteDataset"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes the specified DataBrew job.
*/
override suspend fun deleteJob(input: DeleteJobRequest): DeleteJobResponse {
val op = SdkHttpOperation.build {
serializer = DeleteJobOperationSerializer()
deserializer = DeleteJobOperationDeserializer()
operationName = "DeleteJob"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes an existing DataBrew project.
*/
override suspend fun deleteProject(input: DeleteProjectRequest): DeleteProjectResponse {
val op = SdkHttpOperation.build {
serializer = DeleteProjectOperationSerializer()
deserializer = DeleteProjectOperationDeserializer()
operationName = "DeleteProject"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes a single version of a DataBrew recipe.
*/
override suspend fun deleteRecipeVersion(input: DeleteRecipeVersionRequest): DeleteRecipeVersionResponse {
val op = SdkHttpOperation.build {
serializer = DeleteRecipeVersionOperationSerializer()
deserializer = DeleteRecipeVersionOperationDeserializer()
operationName = "DeleteRecipeVersion"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes a ruleset.
*/
override suspend fun deleteRuleset(input: DeleteRulesetRequest): DeleteRulesetResponse {
val op = SdkHttpOperation.build {
serializer = DeleteRulesetOperationSerializer()
deserializer = DeleteRulesetOperationDeserializer()
operationName = "DeleteRuleset"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes the specified DataBrew schedule.
*/
override suspend fun deleteSchedule(input: DeleteScheduleRequest): DeleteScheduleResponse {
val op = SdkHttpOperation.build {
serializer = DeleteScheduleOperationSerializer()
deserializer = DeleteScheduleOperationDeserializer()
operationName = "DeleteSchedule"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns the definition of a specific DataBrew dataset.
*/
override suspend fun describeDataset(input: DescribeDatasetRequest): DescribeDatasetResponse {
val op = SdkHttpOperation.build {
serializer = DescribeDatasetOperationSerializer()
deserializer = DescribeDatasetOperationDeserializer()
operationName = "DescribeDataset"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns the definition of a specific DataBrew job.
*/
override suspend fun describeJob(input: DescribeJobRequest): DescribeJobResponse {
val op = SdkHttpOperation.build {
serializer = DescribeJobOperationSerializer()
deserializer = DescribeJobOperationDeserializer()
operationName = "DescribeJob"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Represents one run of a DataBrew job.
*/
override suspend fun describeJobRun(input: DescribeJobRunRequest): DescribeJobRunResponse {
val op = SdkHttpOperation.build {
serializer = DescribeJobRunOperationSerializer()
deserializer = DescribeJobRunOperationDeserializer()
operationName = "DescribeJobRun"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns the definition of a specific DataBrew project.
*/
override suspend fun describeProject(input: DescribeProjectRequest): DescribeProjectResponse {
val op = SdkHttpOperation.build {
serializer = DescribeProjectOperationSerializer()
deserializer = DescribeProjectOperationDeserializer()
operationName = "DescribeProject"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns the definition of a specific DataBrew recipe corresponding to a particular version.
*/
override suspend fun describeRecipe(input: DescribeRecipeRequest): DescribeRecipeResponse {
val op = SdkHttpOperation.build {
serializer = DescribeRecipeOperationSerializer()
deserializer = DescribeRecipeOperationDeserializer()
operationName = "DescribeRecipe"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Retrieves detailed information about the ruleset.
*/
override suspend fun describeRuleset(input: DescribeRulesetRequest): DescribeRulesetResponse {
val op = SdkHttpOperation.build {
serializer = DescribeRulesetOperationSerializer()
deserializer = DescribeRulesetOperationDeserializer()
operationName = "DescribeRuleset"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns the definition of a specific DataBrew schedule.
*/
override suspend fun describeSchedule(input: DescribeScheduleRequest): DescribeScheduleResponse {
val op = SdkHttpOperation.build {
serializer = DescribeScheduleOperationSerializer()
deserializer = DescribeScheduleOperationDeserializer()
operationName = "DescribeSchedule"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists all of the DataBrew datasets.
*/
override suspend fun listDatasets(input: ListDatasetsRequest): ListDatasetsResponse {
val op = SdkHttpOperation.build {
serializer = ListDatasetsOperationSerializer()
deserializer = ListDatasetsOperationDeserializer()
operationName = "ListDatasets"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists all of the previous runs of a particular DataBrew job.
*/
override suspend fun listJobRuns(input: ListJobRunsRequest): ListJobRunsResponse {
val op = SdkHttpOperation.build {
serializer = ListJobRunsOperationSerializer()
deserializer = ListJobRunsOperationDeserializer()
operationName = "ListJobRuns"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists all of the DataBrew jobs that are defined.
*/
override suspend fun listJobs(input: ListJobsRequest): ListJobsResponse {
val op = SdkHttpOperation.build {
serializer = ListJobsOperationSerializer()
deserializer = ListJobsOperationDeserializer()
operationName = "ListJobs"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists all of the DataBrew projects that are defined.
*/
override suspend fun listProjects(input: ListProjectsRequest): ListProjectsResponse {
val op = SdkHttpOperation.build {
serializer = ListProjectsOperationSerializer()
deserializer = ListProjectsOperationDeserializer()
operationName = "ListProjects"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists the versions of a particular DataBrew recipe, except for `LATEST_WORKING`.
*/
override suspend fun listRecipeVersions(input: ListRecipeVersionsRequest): ListRecipeVersionsResponse {
val op = SdkHttpOperation.build {
serializer = ListRecipeVersionsOperationSerializer()
deserializer = ListRecipeVersionsOperationDeserializer()
operationName = "ListRecipeVersions"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists all of the DataBrew recipes that are defined.
*/
override suspend fun listRecipes(input: ListRecipesRequest): ListRecipesResponse {
val op = SdkHttpOperation.build {
serializer = ListRecipesOperationSerializer()
deserializer = ListRecipesOperationDeserializer()
operationName = "ListRecipes"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* List all rulesets available in the current account or rulesets associated with a specific resource (dataset).
*/
override suspend fun listRulesets(input: ListRulesetsRequest): ListRulesetsResponse {
val op = SdkHttpOperation.build {
serializer = ListRulesetsOperationSerializer()
deserializer = ListRulesetsOperationDeserializer()
operationName = "ListRulesets"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists the DataBrew schedules that are defined.
*/
override suspend fun listSchedules(input: ListSchedulesRequest): ListSchedulesResponse {
val op = SdkHttpOperation.build {
serializer = ListSchedulesOperationSerializer()
deserializer = ListSchedulesOperationDeserializer()
operationName = "ListSchedules"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists all the tags for a DataBrew resource.
*/
override suspend fun listTagsForResource(input: ListTagsForResourceRequest): ListTagsForResourceResponse {
val op = SdkHttpOperation.build {
serializer = ListTagsForResourceOperationSerializer()
deserializer = ListTagsForResourceOperationDeserializer()
operationName = "ListTagsForResource"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Publishes a new version of a DataBrew recipe.
*/
override suspend fun publishRecipe(input: PublishRecipeRequest): PublishRecipeResponse {
val op = SdkHttpOperation.build {
serializer = PublishRecipeOperationSerializer()
deserializer = PublishRecipeOperationDeserializer()
operationName = "PublishRecipe"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Performs a recipe step within an interactive DataBrew session that's currently open.
*/
override suspend fun sendProjectSessionAction(input: SendProjectSessionActionRequest): SendProjectSessionActionResponse {
val op = SdkHttpOperation.build {
serializer = SendProjectSessionActionOperationSerializer()
deserializer = SendProjectSessionActionOperationDeserializer()
operationName = "SendProjectSessionAction"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Runs a DataBrew job.
*/
override suspend fun startJobRun(input: StartJobRunRequest): StartJobRunResponse {
val op = SdkHttpOperation.build {
serializer = StartJobRunOperationSerializer()
deserializer = StartJobRunOperationDeserializer()
operationName = "StartJobRun"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates an interactive session, enabling you to manipulate data in a DataBrew project.
*/
override suspend fun startProjectSession(input: StartProjectSessionRequest): StartProjectSessionResponse {
val op = SdkHttpOperation.build {
serializer = StartProjectSessionOperationSerializer()
deserializer = StartProjectSessionOperationDeserializer()
operationName = "StartProjectSession"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Stops a particular run of a job.
*/
override suspend fun stopJobRun(input: StopJobRunRequest): StopJobRunResponse {
val op = SdkHttpOperation.build {
serializer = StopJobRunOperationSerializer()
deserializer = StopJobRunOperationDeserializer()
operationName = "StopJobRun"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Adds metadata tags to a DataBrew resource, such as a dataset, project, recipe, job, or schedule.
*/
override suspend fun tagResource(input: TagResourceRequest): TagResourceResponse {
val op = SdkHttpOperation.build {
serializer = TagResourceOperationSerializer()
deserializer = TagResourceOperationDeserializer()
operationName = "TagResource"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Removes metadata tags from a DataBrew resource.
*/
override suspend fun untagResource(input: UntagResourceRequest): UntagResourceResponse {
val op = SdkHttpOperation.build {
serializer = UntagResourceOperationSerializer()
deserializer = UntagResourceOperationDeserializer()
operationName = "UntagResource"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Modifies the definition of an existing DataBrew dataset.
*/
override suspend fun updateDataset(input: UpdateDatasetRequest): UpdateDatasetResponse {
val op = SdkHttpOperation.build {
serializer = UpdateDatasetOperationSerializer()
deserializer = UpdateDatasetOperationDeserializer()
operationName = "UpdateDataset"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Modifies the definition of an existing profile job.
*/
override suspend fun updateProfileJob(input: UpdateProfileJobRequest): UpdateProfileJobResponse {
val op = SdkHttpOperation.build {
serializer = UpdateProfileJobOperationSerializer()
deserializer = UpdateProfileJobOperationDeserializer()
operationName = "UpdateProfileJob"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Modifies the definition of an existing DataBrew project.
*/
override suspend fun updateProject(input: UpdateProjectRequest): UpdateProjectResponse {
val op = SdkHttpOperation.build {
serializer = UpdateProjectOperationSerializer()
deserializer = UpdateProjectOperationDeserializer()
operationName = "UpdateProject"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Modifies the definition of the `LATEST_WORKING` version of a DataBrew recipe.
*/
override suspend fun updateRecipe(input: UpdateRecipeRequest): UpdateRecipeResponse {
val op = SdkHttpOperation.build {
serializer = UpdateRecipeOperationSerializer()
deserializer = UpdateRecipeOperationDeserializer()
operationName = "UpdateRecipe"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Modifies the definition of an existing DataBrew recipe job.
*/
override suspend fun updateRecipeJob(input: UpdateRecipeJobRequest): UpdateRecipeJobResponse {
val op = SdkHttpOperation.build {
serializer = UpdateRecipeJobOperationSerializer()
deserializer = UpdateRecipeJobOperationDeserializer()
operationName = "UpdateRecipeJob"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates specified ruleset.
*/
override suspend fun updateRuleset(input: UpdateRulesetRequest): UpdateRulesetResponse {
val op = SdkHttpOperation.build {
serializer = UpdateRulesetOperationSerializer()
deserializer = UpdateRulesetOperationDeserializer()
operationName = "UpdateRuleset"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Modifies the definition of an existing DataBrew schedule.
*/
override suspend fun updateSchedule(input: UpdateScheduleRequest): UpdateScheduleResponse {
val op = SdkHttpOperation.build {
serializer = UpdateScheduleOperationSerializer()
deserializer = UpdateScheduleOperationDeserializer()
operationName = "UpdateSchedule"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
override fun close() {
managedResources.unshareAll()
}
/**
* merge the defaults configured for the service into the execution context before firing off a request
*/
private fun mergeServiceDefaults(ctx: ExecutionContext) {
ctx.putIfAbsentNotNull(AwsClientOption.Region, config.region)
ctx.putIfAbsent(SdkClientOption.ClientName, config.clientName)
ctx.putIfAbsent(SdkClientOption.LogMode, config.logMode)
ctx.putIfAbsent(AwsSigningAttributes.SigningService, "databrew")
ctx.putIfAbsentNotNull(AwsSigningAttributes.SigningRegion, config.region)
ctx.putIfAbsent(AwsSigningAttributes.CredentialsProvider, config.credentialsProvider)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy