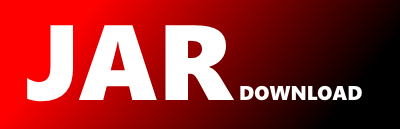
commonMain.aws.sdk.kotlin.services.databrew.model.DatabaseOutput.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databrew.model
/**
* Represents a JDBC database output object which defines the output destination for a DataBrew recipe job to write into.
*/
public class DatabaseOutput private constructor(builder: Builder) {
/**
* Represents options that specify how and where DataBrew writes the database output generated by recipe jobs.
*/
public val databaseOptions: aws.sdk.kotlin.services.databrew.model.DatabaseTableOutputOptions? = builder.databaseOptions
/**
* The output mode to write into the database. Currently supported option: NEW_TABLE.
*/
public val databaseOutputMode: aws.sdk.kotlin.services.databrew.model.DatabaseOutputMode? = builder.databaseOutputMode
/**
* The Glue connection that stores the connection information for the target database.
*/
public val glueConnectionName: kotlin.String = requireNotNull(builder.glueConnectionName) { "A non-null value must be provided for glueConnectionName" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databrew.model.DatabaseOutput = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DatabaseOutput(")
append("databaseOptions=$databaseOptions,")
append("databaseOutputMode=$databaseOutputMode,")
append("glueConnectionName=$glueConnectionName")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = databaseOptions?.hashCode() ?: 0
result = 31 * result + (databaseOutputMode?.hashCode() ?: 0)
result = 31 * result + (glueConnectionName.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DatabaseOutput
if (databaseOptions != other.databaseOptions) return false
if (databaseOutputMode != other.databaseOutputMode) return false
if (glueConnectionName != other.glueConnectionName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databrew.model.DatabaseOutput = Builder(this).apply(block).build()
public class Builder {
/**
* Represents options that specify how and where DataBrew writes the database output generated by recipe jobs.
*/
public var databaseOptions: aws.sdk.kotlin.services.databrew.model.DatabaseTableOutputOptions? = null
/**
* The output mode to write into the database. Currently supported option: NEW_TABLE.
*/
public var databaseOutputMode: aws.sdk.kotlin.services.databrew.model.DatabaseOutputMode? = null
/**
* The Glue connection that stores the connection information for the target database.
*/
public var glueConnectionName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databrew.model.DatabaseOutput) : this() {
this.databaseOptions = x.databaseOptions
this.databaseOutputMode = x.databaseOutputMode
this.glueConnectionName = x.glueConnectionName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databrew.model.DatabaseOutput = DatabaseOutput(this)
/**
* construct an [aws.sdk.kotlin.services.databrew.model.DatabaseTableOutputOptions] inside the given [block]
*/
public fun databaseOptions(block: aws.sdk.kotlin.services.databrew.model.DatabaseTableOutputOptions.Builder.() -> kotlin.Unit) {
this.databaseOptions = aws.sdk.kotlin.services.databrew.model.DatabaseTableOutputOptions.invoke(block)
}
internal fun correctErrors(): Builder {
if (glueConnectionName == null) glueConnectionName = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy