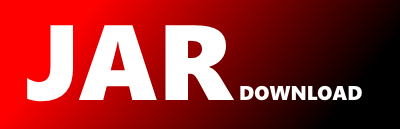
commonMain.aws.sdk.kotlin.services.databrew.model.ProfileConfiguration.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databrew-jvm Show documentation
Show all versions of databrew-jvm Show documentation
The AWS Kotlin client for DataBrew
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databrew.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Configuration for profile jobs. Configuration can be used to select columns, do evaluations, and override default parameters of evaluations. When configuration is undefined, the profile job will apply default settings to all supported columns.
*/
public class ProfileConfiguration private constructor(builder: Builder) {
/**
* List of configurations for column evaluations. ColumnStatisticsConfigurations are used to select evaluations and override parameters of evaluations for particular columns. When ColumnStatisticsConfigurations is undefined, the profile job will profile all supported columns and run all supported evaluations.
*/
public val columnStatisticsConfigurations: List? = builder.columnStatisticsConfigurations
/**
* Configuration for inter-column evaluations. Configuration can be used to select evaluations and override parameters of evaluations. When configuration is undefined, the profile job will run all supported inter-column evaluations.
*/
public val datasetStatisticsConfiguration: aws.sdk.kotlin.services.databrew.model.StatisticsConfiguration? = builder.datasetStatisticsConfiguration
/**
* Configuration of entity detection for a profile job. When undefined, entity detection is disabled.
*/
public val entityDetectorConfiguration: aws.sdk.kotlin.services.databrew.model.EntityDetectorConfiguration? = builder.entityDetectorConfiguration
/**
* List of column selectors. ProfileColumns can be used to select columns from the dataset. When ProfileColumns is undefined, the profile job will profile all supported columns.
*/
public val profileColumns: List? = builder.profileColumns
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databrew.model.ProfileConfiguration = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ProfileConfiguration(")
append("columnStatisticsConfigurations=$columnStatisticsConfigurations,")
append("datasetStatisticsConfiguration=$datasetStatisticsConfiguration,")
append("entityDetectorConfiguration=$entityDetectorConfiguration,")
append("profileColumns=$profileColumns")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = columnStatisticsConfigurations?.hashCode() ?: 0
result = 31 * result + (datasetStatisticsConfiguration?.hashCode() ?: 0)
result = 31 * result + (entityDetectorConfiguration?.hashCode() ?: 0)
result = 31 * result + (profileColumns?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ProfileConfiguration
if (columnStatisticsConfigurations != other.columnStatisticsConfigurations) return false
if (datasetStatisticsConfiguration != other.datasetStatisticsConfiguration) return false
if (entityDetectorConfiguration != other.entityDetectorConfiguration) return false
if (profileColumns != other.profileColumns) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databrew.model.ProfileConfiguration = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* List of configurations for column evaluations. ColumnStatisticsConfigurations are used to select evaluations and override parameters of evaluations for particular columns. When ColumnStatisticsConfigurations is undefined, the profile job will profile all supported columns and run all supported evaluations.
*/
public var columnStatisticsConfigurations: List? = null
/**
* Configuration for inter-column evaluations. Configuration can be used to select evaluations and override parameters of evaluations. When configuration is undefined, the profile job will run all supported inter-column evaluations.
*/
public var datasetStatisticsConfiguration: aws.sdk.kotlin.services.databrew.model.StatisticsConfiguration? = null
/**
* Configuration of entity detection for a profile job. When undefined, entity detection is disabled.
*/
public var entityDetectorConfiguration: aws.sdk.kotlin.services.databrew.model.EntityDetectorConfiguration? = null
/**
* List of column selectors. ProfileColumns can be used to select columns from the dataset. When ProfileColumns is undefined, the profile job will profile all supported columns.
*/
public var profileColumns: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databrew.model.ProfileConfiguration) : this() {
this.columnStatisticsConfigurations = x.columnStatisticsConfigurations
this.datasetStatisticsConfiguration = x.datasetStatisticsConfiguration
this.entityDetectorConfiguration = x.entityDetectorConfiguration
this.profileColumns = x.profileColumns
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databrew.model.ProfileConfiguration = ProfileConfiguration(this)
/**
* construct an [aws.sdk.kotlin.services.databrew.model.StatisticsConfiguration] inside the given [block]
*/
public fun datasetStatisticsConfiguration(block: aws.sdk.kotlin.services.databrew.model.StatisticsConfiguration.Builder.() -> kotlin.Unit) {
this.datasetStatisticsConfiguration = aws.sdk.kotlin.services.databrew.model.StatisticsConfiguration.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databrew.model.EntityDetectorConfiguration] inside the given [block]
*/
public fun entityDetectorConfiguration(block: aws.sdk.kotlin.services.databrew.model.EntityDetectorConfiguration.Builder.() -> kotlin.Unit) {
this.entityDetectorConfiguration = aws.sdk.kotlin.services.databrew.model.EntityDetectorConfiguration.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy