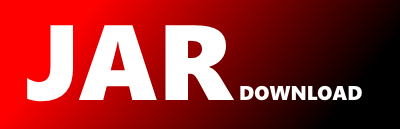
commonMain.aws.sdk.kotlin.services.datapipeline.model.CreatePipelineRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datapipeline-jvm Show documentation
Show all versions of datapipeline-jvm Show documentation
The AWS SDK for Kotlin client for Data Pipeline
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datapipeline.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Contains the parameters for CreatePipeline.
*/
public class CreatePipelineRequest private constructor(builder: Builder) {
/**
* The description for the pipeline.
*/
public val description: kotlin.String? = builder.description
/**
* The name for the pipeline. You can use the same name for multiple pipelines associated with your AWS account, because AWS Data Pipeline assigns each pipeline a unique pipeline identifier.
*/
public val name: kotlin.String? = builder.name
/**
* A list of tags to associate with the pipeline at creation. Tags let you control access to pipelines. For more information, see [Controlling User Access to Pipelines](http://docs.aws.amazon.com/datapipeline/latest/DeveloperGuide/dp-control-access.html) in the *AWS Data Pipeline Developer Guide*.
*/
public val tags: List? = builder.tags
/**
* A unique identifier. This identifier is not the same as the pipeline identifier assigned by AWS Data Pipeline. You are responsible for defining the format and ensuring the uniqueness of this identifier. You use this parameter to ensure idempotency during repeated calls to `CreatePipeline`. For example, if the first call to `CreatePipeline` does not succeed, you can pass in the same unique identifier and pipeline name combination on a subsequent call to `CreatePipeline`. `CreatePipeline` ensures that if a pipeline already exists with the same name and unique identifier, a new pipeline is not created. Instead, you'll receive the pipeline identifier from the previous attempt. The uniqueness of the name and unique identifier combination is scoped to the AWS account or IAM user credentials.
*/
public val uniqueId: kotlin.String? = builder.uniqueId
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datapipeline.model.CreatePipelineRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreatePipelineRequest(")
append("description=$description,")
append("name=$name,")
append("tags=$tags,")
append("uniqueId=$uniqueId")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = description?.hashCode() ?: 0
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (uniqueId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreatePipelineRequest
if (description != other.description) return false
if (name != other.name) return false
if (tags != other.tags) return false
if (uniqueId != other.uniqueId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datapipeline.model.CreatePipelineRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The description for the pipeline.
*/
public var description: kotlin.String? = null
/**
* The name for the pipeline. You can use the same name for multiple pipelines associated with your AWS account, because AWS Data Pipeline assigns each pipeline a unique pipeline identifier.
*/
public var name: kotlin.String? = null
/**
* A list of tags to associate with the pipeline at creation. Tags let you control access to pipelines. For more information, see [Controlling User Access to Pipelines](http://docs.aws.amazon.com/datapipeline/latest/DeveloperGuide/dp-control-access.html) in the *AWS Data Pipeline Developer Guide*.
*/
public var tags: List? = null
/**
* A unique identifier. This identifier is not the same as the pipeline identifier assigned by AWS Data Pipeline. You are responsible for defining the format and ensuring the uniqueness of this identifier. You use this parameter to ensure idempotency during repeated calls to `CreatePipeline`. For example, if the first call to `CreatePipeline` does not succeed, you can pass in the same unique identifier and pipeline name combination on a subsequent call to `CreatePipeline`. `CreatePipeline` ensures that if a pipeline already exists with the same name and unique identifier, a new pipeline is not created. Instead, you'll receive the pipeline identifier from the previous attempt. The uniqueness of the name and unique identifier combination is scoped to the AWS account or IAM user credentials.
*/
public var uniqueId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datapipeline.model.CreatePipelineRequest) : this() {
this.description = x.description
this.name = x.name
this.tags = x.tags
this.uniqueId = x.uniqueId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datapipeline.model.CreatePipelineRequest = CreatePipelineRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy