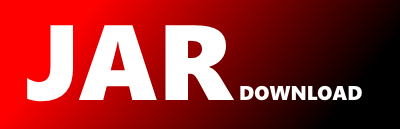
commonMain.aws.sdk.kotlin.services.datapipeline.model.SetTaskStatusRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datapipeline-jvm Show documentation
Show all versions of datapipeline-jvm Show documentation
The AWS SDK for Kotlin client for Data Pipeline
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datapipeline.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Contains the parameters for SetTaskStatus.
*/
public class SetTaskStatusRequest private constructor(builder: Builder) {
/**
* If an error occurred during the task, this value specifies the error code. This value is set on the physical attempt object. It is used to display error information to the user. It should not start with string "Service_" which is reserved by the system.
*/
public val errorId: kotlin.String? = builder.errorId
/**
* If an error occurred during the task, this value specifies a text description of the error. This value is set on the physical attempt object. It is used to display error information to the user. The web service does not parse this value.
*/
public val errorMessage: kotlin.String? = builder.errorMessage
/**
* If an error occurred during the task, this value specifies the stack trace associated with the error. This value is set on the physical attempt object. It is used to display error information to the user. The web service does not parse this value.
*/
public val errorStackTrace: kotlin.String? = builder.errorStackTrace
/**
* The ID of the task assigned to the task runner. This value is provided in the response for PollForTask.
*/
public val taskId: kotlin.String? = builder.taskId
/**
* If `FINISHED`, the task successfully completed. If `FAILED`, the task ended unsuccessfully. Preconditions use false.
*/
public val taskStatus: aws.sdk.kotlin.services.datapipeline.model.TaskStatus? = builder.taskStatus
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datapipeline.model.SetTaskStatusRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("SetTaskStatusRequest(")
append("errorId=$errorId,")
append("errorMessage=$errorMessage,")
append("errorStackTrace=$errorStackTrace,")
append("taskId=$taskId,")
append("taskStatus=$taskStatus")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = errorId?.hashCode() ?: 0
result = 31 * result + (errorMessage?.hashCode() ?: 0)
result = 31 * result + (errorStackTrace?.hashCode() ?: 0)
result = 31 * result + (taskId?.hashCode() ?: 0)
result = 31 * result + (taskStatus?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as SetTaskStatusRequest
if (errorId != other.errorId) return false
if (errorMessage != other.errorMessage) return false
if (errorStackTrace != other.errorStackTrace) return false
if (taskId != other.taskId) return false
if (taskStatus != other.taskStatus) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datapipeline.model.SetTaskStatusRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* If an error occurred during the task, this value specifies the error code. This value is set on the physical attempt object. It is used to display error information to the user. It should not start with string "Service_" which is reserved by the system.
*/
public var errorId: kotlin.String? = null
/**
* If an error occurred during the task, this value specifies a text description of the error. This value is set on the physical attempt object. It is used to display error information to the user. The web service does not parse this value.
*/
public var errorMessage: kotlin.String? = null
/**
* If an error occurred during the task, this value specifies the stack trace associated with the error. This value is set on the physical attempt object. It is used to display error information to the user. The web service does not parse this value.
*/
public var errorStackTrace: kotlin.String? = null
/**
* The ID of the task assigned to the task runner. This value is provided in the response for PollForTask.
*/
public var taskId: kotlin.String? = null
/**
* If `FINISHED`, the task successfully completed. If `FAILED`, the task ended unsuccessfully. Preconditions use false.
*/
public var taskStatus: aws.sdk.kotlin.services.datapipeline.model.TaskStatus? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datapipeline.model.SetTaskStatusRequest) : this() {
this.errorId = x.errorId
this.errorMessage = x.errorMessage
this.errorStackTrace = x.errorStackTrace
this.taskId = x.taskId
this.taskStatus = x.taskStatus
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datapipeline.model.SetTaskStatusRequest = SetTaskStatusRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy