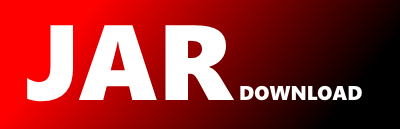
commonMain.aws.sdk.kotlin.services.datasync.DefaultDataSyncClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datasync-jvm Show documentation
Show all versions of datasync-jvm Show documentation
The AWS SDK for Kotlin client for DataSync
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync
import aws.sdk.kotlin.runtime.http.ApiMetadata
import aws.sdk.kotlin.runtime.http.AwsUserAgentMetadata
import aws.sdk.kotlin.runtime.http.interceptors.AwsSpanInterceptor
import aws.sdk.kotlin.runtime.http.interceptors.BusinessMetricsInterceptor
import aws.sdk.kotlin.runtime.http.middleware.AwsRetryHeaderMiddleware
import aws.sdk.kotlin.runtime.http.middleware.RecursionDetection
import aws.sdk.kotlin.runtime.http.middleware.UserAgent
import aws.sdk.kotlin.services.datasync.auth.DataSyncAuthSchemeProviderAdapter
import aws.sdk.kotlin.services.datasync.auth.DataSyncIdentityProviderConfigAdapter
import aws.sdk.kotlin.services.datasync.endpoints.internal.EndpointResolverAdapter
import aws.sdk.kotlin.services.datasync.model.*
import aws.sdk.kotlin.services.datasync.serde.*
import aws.smithy.kotlin.runtime.auth.AuthSchemeId
import aws.smithy.kotlin.runtime.auth.awssigning.AwsSigningAttributes
import aws.smithy.kotlin.runtime.auth.awssigning.DefaultAwsSigner
import aws.smithy.kotlin.runtime.awsprotocol.AwsAttributes
import aws.smithy.kotlin.runtime.awsprotocol.json.AwsJsonProtocol
import aws.smithy.kotlin.runtime.client.SdkClientOption
import aws.smithy.kotlin.runtime.collections.attributesOf
import aws.smithy.kotlin.runtime.collections.putIfAbsent
import aws.smithy.kotlin.runtime.collections.putIfAbsentNotNull
import aws.smithy.kotlin.runtime.http.SdkHttpClient
import aws.smithy.kotlin.runtime.http.auth.AuthScheme
import aws.smithy.kotlin.runtime.http.auth.SigV4AuthScheme
import aws.smithy.kotlin.runtime.http.operation.OperationAuthConfig
import aws.smithy.kotlin.runtime.http.operation.OperationMetrics
import aws.smithy.kotlin.runtime.http.operation.SdkHttpOperation
import aws.smithy.kotlin.runtime.http.operation.context
import aws.smithy.kotlin.runtime.http.operation.roundTrip
import aws.smithy.kotlin.runtime.http.operation.telemetry
import aws.smithy.kotlin.runtime.io.SdkManagedGroup
import aws.smithy.kotlin.runtime.io.addIfManaged
import aws.smithy.kotlin.runtime.operation.ExecutionContext
internal class DefaultDataSyncClient(override val config: DataSyncClient.Config) : DataSyncClient {
private val managedResources = SdkManagedGroup()
private val client = SdkHttpClient(config.httpClient)
private val identityProviderConfig = DataSyncIdentityProviderConfigAdapter(config)
private val configuredAuthSchemes = with(config.authSchemes.associateBy(AuthScheme::schemeId).toMutableMap()){
getOrPut(AuthSchemeId.AwsSigV4){
SigV4AuthScheme(DefaultAwsSigner, "datasync")
}
toMap()
}
private val authSchemeAdapter = DataSyncAuthSchemeProviderAdapter(config)
private val telemetryScope = "aws.sdk.kotlin.services.datasync"
private val opMetrics = OperationMetrics(telemetryScope, config.telemetryProvider)
init {
managedResources.addIfManaged(config.httpClient)
managedResources.addIfManaged(config.credentialsProvider)
}
private val awsUserAgentMetadata = AwsUserAgentMetadata.fromEnvironment(ApiMetadata(ServiceId, SdkVersion), config.applicationId)
/**
* Creates an Amazon Web Services resource for an on-premises storage system that you want DataSync Discovery to collect information about.
*/
override suspend fun addStorageSystem(input: AddStorageSystemRequest): AddStorageSystemResponse {
val op = SdkHttpOperation.build {
serializeWith = AddStorageSystemOperationSerializer()
deserializeWith = AddStorageSystemOperationDeserializer()
operationName = "AddStorageSystem"
serviceName = ServiceId
hostPrefix = "discovery-"
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Stops an DataSync task execution that's in progress. The transfer of some files are abruptly interrupted. File contents that're transferred to the destination might be incomplete or inconsistent with the source files.
*
* However, if you start a new task execution using the same task and allow it to finish, file content on the destination will be complete and consistent. This applies to other unexpected failures that interrupt a task execution. In all of these cases, DataSync successfully completes the transfer when you start the next task execution.
*/
override suspend fun cancelTaskExecution(input: CancelTaskExecutionRequest): CancelTaskExecutionResponse {
val op = SdkHttpOperation.build {
serializeWith = CancelTaskExecutionOperationSerializer()
deserializeWith = CancelTaskExecutionOperationDeserializer()
operationName = "CancelTaskExecution"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Activates an DataSync agent that you've deployed in your storage environment. The activation process associates the agent with your Amazon Web Services account.
*
* If you haven't deployed an agent yet, see the following topics to learn more:
* + [Agent requirements](https://docs.aws.amazon.com/datasync/latest/userguide/agent-requirements.html)
* + [Create an agent](https://docs.aws.amazon.com/datasync/latest/userguide/configure-agent.html)
*
* If you're transferring between Amazon Web Services storage services, you don't need a DataSync agent.
*/
override suspend fun createAgent(input: CreateAgentRequest): CreateAgentResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateAgentOperationSerializer()
deserializeWith = CreateAgentOperationDeserializer()
operationName = "CreateAgent"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a transfer *location* for a Microsoft Azure Blob Storage container. DataSync can use this location as a transfer source or destination.
*
* Before you begin, make sure you know [how DataSync accesses Azure Blob Storage](https://docs.aws.amazon.com/datasync/latest/userguide/creating-azure-blob-location.html#azure-blob-access) and works with [access tiers](https://docs.aws.amazon.com/datasync/latest/userguide/creating-azure-blob-location.html#azure-blob-access-tiers) and [blob types](https://docs.aws.amazon.com/datasync/latest/userguide/creating-azure-blob-location.html#blob-types). You also need a [DataSync agent](https://docs.aws.amazon.com/datasync/latest/userguide/creating-azure-blob-location.html#azure-blob-creating-agent) that can connect to your container.
*/
override suspend fun createLocationAzureBlob(input: CreateLocationAzureBlobRequest): CreateLocationAzureBlobResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateLocationAzureBlobOperationSerializer()
deserializeWith = CreateLocationAzureBlobOperationDeserializer()
operationName = "CreateLocationAzureBlob"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a transfer *location* for an Amazon EFS file system. DataSync can use this location as a source or destination for transferring data.
*
* Before you begin, make sure that you understand how DataSync [accesses Amazon EFS file systems](https://docs.aws.amazon.com/datasync/latest/userguide/create-efs-location.html#create-efs-location-access).
*/
override suspend fun createLocationEfs(input: CreateLocationEfsRequest): CreateLocationEfsResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateLocationEfsOperationSerializer()
deserializeWith = CreateLocationEfsOperationDeserializer()
operationName = "CreateLocationEfs"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a transfer *location* for an Amazon FSx for Lustre file system. DataSync can use this location as a source or destination for transferring data.
*
* Before you begin, make sure that you understand how DataSync [accesses FSx for Lustre file systems](https://docs.aws.amazon.com/datasync/latest/userguide/create-lustre-location.html#create-lustre-location-access).
*/
override suspend fun createLocationFsxLustre(input: CreateLocationFsxLustreRequest): CreateLocationFsxLustreResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateLocationFsxLustreOperationSerializer()
deserializeWith = CreateLocationFsxLustreOperationDeserializer()
operationName = "CreateLocationFsxLustre"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a transfer *location* for an Amazon FSx for NetApp ONTAP file system. DataSync can use this location as a source or destination for transferring data.
*
* Before you begin, make sure that you understand how DataSync [accesses FSx for ONTAP file systems](https://docs.aws.amazon.com/datasync/latest/userguide/create-ontap-location.html#create-ontap-location-access).
*/
override suspend fun createLocationFsxOntap(input: CreateLocationFsxOntapRequest): CreateLocationFsxOntapResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateLocationFsxOntapOperationSerializer()
deserializeWith = CreateLocationFsxOntapOperationDeserializer()
operationName = "CreateLocationFsxOntap"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a transfer *location* for an Amazon FSx for OpenZFS file system. DataSync can use this location as a source or destination for transferring data.
*
* Before you begin, make sure that you understand how DataSync [accesses FSx for OpenZFS file systems](https://docs.aws.amazon.com/datasync/latest/userguide/create-openzfs-location.html#create-openzfs-access).
*
* Request parameters related to `SMB` aren't supported with the `CreateLocationFsxOpenZfs` operation.
*/
override suspend fun createLocationFsxOpenZfs(input: CreateLocationFsxOpenZfsRequest): CreateLocationFsxOpenZfsResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateLocationFsxOpenZfsOperationSerializer()
deserializeWith = CreateLocationFsxOpenZfsOperationDeserializer()
operationName = "CreateLocationFsxOpenZfs"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a transfer *location* for an Amazon FSx for Windows File Server file system. DataSync can use this location as a source or destination for transferring data.
*
* Before you begin, make sure that you understand how DataSync [accesses FSx for Windows File Server file systems](https://docs.aws.amazon.com/datasync/latest/userguide/create-fsx-location.html#create-fsx-location-access).
*/
override suspend fun createLocationFsxWindows(input: CreateLocationFsxWindowsRequest): CreateLocationFsxWindowsResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateLocationFsxWindowsOperationSerializer()
deserializeWith = CreateLocationFsxWindowsOperationDeserializer()
operationName = "CreateLocationFsxWindows"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a transfer *location* for a Hadoop Distributed File System (HDFS). DataSync can use this location as a source or destination for transferring data.
*
* Before you begin, make sure that you understand how DataSync [accesses HDFS clusters](https://docs.aws.amazon.com/datasync/latest/userguide/create-hdfs-location.html#accessing-hdfs).
*/
override suspend fun createLocationHdfs(input: CreateLocationHdfsRequest): CreateLocationHdfsResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateLocationHdfsOperationSerializer()
deserializeWith = CreateLocationHdfsOperationDeserializer()
operationName = "CreateLocationHdfs"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a transfer *location* for a Network File System (NFS) file server. DataSync can use this location as a source or destination for transferring data.
*
* Before you begin, make sure that you understand how DataSync [accesses NFS file servers](https://docs.aws.amazon.com/datasync/latest/userguide/create-nfs-location.html#accessing-nfs).
*
* If you're copying data to or from an Snowcone device, you can also use `CreateLocationNfs` to create your transfer location. For more information, see [Configuring transfers with Snowcone](https://docs.aws.amazon.com/datasync/latest/userguide/nfs-on-snowcone.html).
*/
override suspend fun createLocationNfs(input: CreateLocationNfsRequest): CreateLocationNfsResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateLocationNfsOperationSerializer()
deserializeWith = CreateLocationNfsOperationDeserializer()
operationName = "CreateLocationNfs"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a transfer *location* for an object storage system. DataSync can use this location as a source or destination for transferring data.
*
* Before you begin, make sure that you understand the [prerequisites](https://docs.aws.amazon.com/datasync/latest/userguide/create-object-location.html#create-object-location-prerequisites) for DataSync to work with object storage systems.
*/
override suspend fun createLocationObjectStorage(input: CreateLocationObjectStorageRequest): CreateLocationObjectStorageResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateLocationObjectStorageOperationSerializer()
deserializeWith = CreateLocationObjectStorageOperationDeserializer()
operationName = "CreateLocationObjectStorage"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a transfer *location* for an Amazon S3 bucket. DataSync can use this location as a source or destination for transferring data.
*
* Before you begin, make sure that you read the following topics:
* + [Storage class considerations with Amazon S3 locations](https://docs.aws.amazon.com/datasync/latest/userguide/create-s3-location.html#using-storage-classes)
* + [Evaluating S3 request costs when using DataSync](https://docs.aws.amazon.com/datasync/latest/userguide/create-s3-location.html#create-s3-location-s3-requests)
* For more information, see [Configuring transfers with Amazon S3](https://docs.aws.amazon.com/datasync/latest/userguide/create-s3-location.html).
*/
override suspend fun createLocationS3(input: CreateLocationS3Request): CreateLocationS3Response {
val op = SdkHttpOperation.build {
serializeWith = CreateLocationS3OperationSerializer()
deserializeWith = CreateLocationS3OperationDeserializer()
operationName = "CreateLocationS3"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a transfer *location* for a Server Message Block (SMB) file server. DataSync can use this location as a source or destination for transferring data.
*
* Before you begin, make sure that you understand how DataSync [accesses SMB file servers](https://docs.aws.amazon.com/datasync/latest/userguide/create-smb-location.html#configuring-smb).
*/
override suspend fun createLocationSmb(input: CreateLocationSmbRequest): CreateLocationSmbResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateLocationSmbOperationSerializer()
deserializeWith = CreateLocationSmbOperationDeserializer()
operationName = "CreateLocationSmb"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Configures a *task*, which defines where and how DataSync transfers your data.
*
* A task includes a source location, destination location, and transfer options (such as bandwidth limits, scheduling, and more).
*
* If you're planning to transfer data to or from an Amazon S3 location, review [how DataSync can affect your S3 request charges](https://docs.aws.amazon.com/datasync/latest/userguide/create-s3-location.html#create-s3-location-s3-requests) and the [DataSync pricing page](http://aws.amazon.com/datasync/pricing/) before you begin.
*/
override suspend fun createTask(input: CreateTaskRequest): CreateTaskResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateTaskOperationSerializer()
deserializeWith = CreateTaskOperationDeserializer()
operationName = "CreateTask"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Removes an DataSync agent resource from your Amazon Web Services account.
*
* Keep in mind that this operation (which can't be undone) doesn't remove the agent's virtual machine (VM) or Amazon EC2 instance from your storage environment. For next steps, you can delete the VM or instance from your storage environment or reuse it to [activate a new agent](https://docs.aws.amazon.com/datasync/latest/userguide/activate-agent.html).
*/
override suspend fun deleteAgent(input: DeleteAgentRequest): DeleteAgentResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteAgentOperationSerializer()
deserializeWith = DeleteAgentOperationDeserializer()
operationName = "DeleteAgent"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes a transfer location resource from DataSync.
*/
override suspend fun deleteLocation(input: DeleteLocationRequest): DeleteLocationResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteLocationOperationSerializer()
deserializeWith = DeleteLocationOperationDeserializer()
operationName = "DeleteLocation"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes a transfer task resource from DataSync.
*/
override suspend fun deleteTask(input: DeleteTaskRequest): DeleteTaskResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteTaskOperationSerializer()
deserializeWith = DeleteTaskOperationDeserializer()
operationName = "DeleteTask"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns information about an DataSync agent, such as its name, service endpoint type, and status.
*/
override suspend fun describeAgent(input: DescribeAgentRequest): DescribeAgentResponse {
val op = SdkHttpOperation.build {
serializeWith = DescribeAgentOperationSerializer()
deserializeWith = DescribeAgentOperationDeserializer()
operationName = "DescribeAgent"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns information about a DataSync discovery job.
*/
override suspend fun describeDiscoveryJob(input: DescribeDiscoveryJobRequest): DescribeDiscoveryJobResponse {
val op = SdkHttpOperation.build {
serializeWith = DescribeDiscoveryJobOperationSerializer()
deserializeWith = DescribeDiscoveryJobOperationDeserializer()
operationName = "DescribeDiscoveryJob"
serviceName = ServiceId
hostPrefix = "discovery-"
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Provides details about how an DataSync transfer location for Microsoft Azure Blob Storage is configured.
*/
override suspend fun describeLocationAzureBlob(input: DescribeLocationAzureBlobRequest): DescribeLocationAzureBlobResponse {
val op = SdkHttpOperation.build {
serializeWith = DescribeLocationAzureBlobOperationSerializer()
deserializeWith = DescribeLocationAzureBlobOperationDeserializer()
operationName = "DescribeLocationAzureBlob"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Provides details about how an DataSync transfer location for an Amazon EFS file system is configured.
*/
override suspend fun describeLocationEfs(input: DescribeLocationEfsRequest): DescribeLocationEfsResponse {
val op = SdkHttpOperation.build {
serializeWith = DescribeLocationEfsOperationSerializer()
deserializeWith = DescribeLocationEfsOperationDeserializer()
operationName = "DescribeLocationEfs"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Provides details about how an DataSync transfer location for an Amazon FSx for Lustre file system is configured.
*/
override suspend fun describeLocationFsxLustre(input: DescribeLocationFsxLustreRequest): DescribeLocationFsxLustreResponse {
val op = SdkHttpOperation.build {
serializeWith = DescribeLocationFsxLustreOperationSerializer()
deserializeWith = DescribeLocationFsxLustreOperationDeserializer()
operationName = "DescribeLocationFsxLustre"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Provides details about how an DataSync transfer location for an Amazon FSx for NetApp ONTAP file system is configured.
*
* If your location uses SMB, the `DescribeLocationFsxOntap` operation doesn't actually return a `Password`.
*/
override suspend fun describeLocationFsxOntap(input: DescribeLocationFsxOntapRequest): DescribeLocationFsxOntapResponse {
val op = SdkHttpOperation.build {
serializeWith = DescribeLocationFsxOntapOperationSerializer()
deserializeWith = DescribeLocationFsxOntapOperationDeserializer()
operationName = "DescribeLocationFsxOntap"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Provides details about how an DataSync transfer location for an Amazon FSx for OpenZFS file system is configured.
*
* Response elements related to `SMB` aren't supported with the `DescribeLocationFsxOpenZfs` operation.
*/
override suspend fun describeLocationFsxOpenZfs(input: DescribeLocationFsxOpenZfsRequest): DescribeLocationFsxOpenZfsResponse {
val op = SdkHttpOperation.build {
serializeWith = DescribeLocationFsxOpenZfsOperationSerializer()
deserializeWith = DescribeLocationFsxOpenZfsOperationDeserializer()
operationName = "DescribeLocationFsxOpenZfs"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Provides details about how an DataSync transfer location for an Amazon FSx for Windows File Server file system is configured.
*/
override suspend fun describeLocationFsxWindows(input: DescribeLocationFsxWindowsRequest): DescribeLocationFsxWindowsResponse {
val op = SdkHttpOperation.build {
serializeWith = DescribeLocationFsxWindowsOperationSerializer()
deserializeWith = DescribeLocationFsxWindowsOperationDeserializer()
operationName = "DescribeLocationFsxWindows"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Provides details about how an DataSync transfer location for a Hadoop Distributed File System (HDFS) is configured.
*/
override suspend fun describeLocationHdfs(input: DescribeLocationHdfsRequest): DescribeLocationHdfsResponse {
val op = SdkHttpOperation.build {
serializeWith = DescribeLocationHdfsOperationSerializer()
deserializeWith = DescribeLocationHdfsOperationDeserializer()
operationName = "DescribeLocationHdfs"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Provides details about how an DataSync transfer location for a Network File System (NFS) file server is configured.
*/
override suspend fun describeLocationNfs(input: DescribeLocationNfsRequest): DescribeLocationNfsResponse {
val op = SdkHttpOperation.build {
serializeWith = DescribeLocationNfsOperationSerializer()
deserializeWith = DescribeLocationNfsOperationDeserializer()
operationName = "DescribeLocationNfs"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Provides details about how an DataSync transfer location for an object storage system is configured.
*/
override suspend fun describeLocationObjectStorage(input: DescribeLocationObjectStorageRequest): DescribeLocationObjectStorageResponse {
val op = SdkHttpOperation.build {
serializeWith = DescribeLocationObjectStorageOperationSerializer()
deserializeWith = DescribeLocationObjectStorageOperationDeserializer()
operationName = "DescribeLocationObjectStorage"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Provides details about how an DataSync transfer location for an S3 bucket is configured.
*/
override suspend fun describeLocationS3(input: DescribeLocationS3Request): DescribeLocationS3Response {
val op = SdkHttpOperation.build {
serializeWith = DescribeLocationS3OperationSerializer()
deserializeWith = DescribeLocationS3OperationDeserializer()
operationName = "DescribeLocationS3"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Provides details about how an DataSync transfer location for a Server Message Block (SMB) file server is configured.
*/
override suspend fun describeLocationSmb(input: DescribeLocationSmbRequest): DescribeLocationSmbResponse {
val op = SdkHttpOperation.build {
serializeWith = DescribeLocationSmbOperationSerializer()
deserializeWith = DescribeLocationSmbOperationDeserializer()
operationName = "DescribeLocationSmb"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns information about an on-premises storage system that you're using with DataSync Discovery.
*/
override suspend fun describeStorageSystem(input: DescribeStorageSystemRequest): DescribeStorageSystemResponse {
val op = SdkHttpOperation.build {
serializeWith = DescribeStorageSystemOperationSerializer()
deserializeWith = DescribeStorageSystemOperationDeserializer()
operationName = "DescribeStorageSystem"
serviceName = ServiceId
hostPrefix = "discovery-"
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns information, including performance data and capacity usage, which DataSync Discovery collects about a specific resource in your-premises storage system.
*/
override suspend fun describeStorageSystemResourceMetrics(input: DescribeStorageSystemResourceMetricsRequest): DescribeStorageSystemResourceMetricsResponse {
val op = SdkHttpOperation.build {
serializeWith = DescribeStorageSystemResourceMetricsOperationSerializer()
deserializeWith = DescribeStorageSystemResourceMetricsOperationDeserializer()
operationName = "DescribeStorageSystemResourceMetrics"
serviceName = ServiceId
hostPrefix = "discovery-"
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns information that DataSync Discovery collects about resources in your on-premises storage system.
*/
override suspend fun describeStorageSystemResources(input: DescribeStorageSystemResourcesRequest): DescribeStorageSystemResourcesResponse {
val op = SdkHttpOperation.build {
serializeWith = DescribeStorageSystemResourcesOperationSerializer()
deserializeWith = DescribeStorageSystemResourcesOperationDeserializer()
operationName = "DescribeStorageSystemResources"
serviceName = ServiceId
hostPrefix = "discovery-"
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Provides information about a *task*, which defines where and how DataSync transfers your data.
*/
override suspend fun describeTask(input: DescribeTaskRequest): DescribeTaskResponse {
val op = SdkHttpOperation.build {
serializeWith = DescribeTaskOperationSerializer()
deserializeWith = DescribeTaskOperationDeserializer()
operationName = "DescribeTask"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Provides information about an execution of your DataSync task. You can use this operation to help monitor the progress of an ongoing transfer or check the results of the transfer.
*/
override suspend fun describeTaskExecution(input: DescribeTaskExecutionRequest): DescribeTaskExecutionResponse {
val op = SdkHttpOperation.build {
serializeWith = DescribeTaskExecutionOperationSerializer()
deserializeWith = DescribeTaskExecutionOperationDeserializer()
operationName = "DescribeTaskExecution"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates recommendations about where to migrate your data to in Amazon Web Services. Recommendations are generated based on information that DataSync Discovery collects about your on-premises storage system's resources. For more information, see [Recommendations provided by DataSync Discovery](https://docs.aws.amazon.com/datasync/latest/userguide/discovery-understand-recommendations.html).
*
* Once generated, you can view your recommendations by using the [DescribeStorageSystemResources](https://docs.aws.amazon.com/datasync/latest/userguide/API_DescribeStorageSystemResources.html) operation.
*/
override suspend fun generateRecommendations(input: GenerateRecommendationsRequest): GenerateRecommendationsResponse {
val op = SdkHttpOperation.build {
serializeWith = GenerateRecommendationsOperationSerializer()
deserializeWith = GenerateRecommendationsOperationDeserializer()
operationName = "GenerateRecommendations"
serviceName = ServiceId
hostPrefix = "discovery-"
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of DataSync agents that belong to an Amazon Web Services account in the Amazon Web Services Region specified in the request.
*
* With pagination, you can reduce the number of agents returned in a response. If you get a truncated list of agents in a response, the response contains a marker that you can specify in your next request to fetch the next page of agents.
*
* `ListAgents` is eventually consistent. This means the result of running the operation might not reflect that you just created or deleted an agent. For example, if you create an agent with [CreateAgent](https://docs.aws.amazon.com/datasync/latest/userguide/API_CreateAgent.html) and then immediately run `ListAgents`, that agent might not show up in the list right away. In situations like this, you can always confirm whether an agent has been created (or deleted) by using [DescribeAgent](https://docs.aws.amazon.com/datasync/latest/userguide/API_DescribeAgent.html).
*/
override suspend fun listAgents(input: ListAgentsRequest): ListAgentsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListAgentsOperationSerializer()
deserializeWith = ListAgentsOperationDeserializer()
operationName = "ListAgents"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Provides a list of the existing discovery jobs in the Amazon Web Services Region and Amazon Web Services account where you're using DataSync Discovery.
*/
override suspend fun listDiscoveryJobs(input: ListDiscoveryJobsRequest): ListDiscoveryJobsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListDiscoveryJobsOperationSerializer()
deserializeWith = ListDiscoveryJobsOperationDeserializer()
operationName = "ListDiscoveryJobs"
serviceName = ServiceId
hostPrefix = "discovery-"
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of source and destination locations.
*
* If you have more locations than are returned in a response (that is, the response returns only a truncated list of your agents), the response contains a token that you can specify in your next request to fetch the next page of locations.
*/
override suspend fun listLocations(input: ListLocationsRequest): ListLocationsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListLocationsOperationSerializer()
deserializeWith = ListLocationsOperationDeserializer()
operationName = "ListLocations"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists the on-premises storage systems that you're using with DataSync Discovery.
*/
override suspend fun listStorageSystems(input: ListStorageSystemsRequest): ListStorageSystemsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListStorageSystemsOperationSerializer()
deserializeWith = ListStorageSystemsOperationDeserializer()
operationName = "ListStorageSystems"
serviceName = ServiceId
hostPrefix = "discovery-"
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns all the tags associated with an Amazon Web Services resource.
*/
override suspend fun listTagsForResource(input: ListTagsForResourceRequest): ListTagsForResourceResponse {
val op = SdkHttpOperation.build {
serializeWith = ListTagsForResourceOperationSerializer()
deserializeWith = ListTagsForResourceOperationDeserializer()
operationName = "ListTagsForResource"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of executions for an DataSync transfer task.
*/
override suspend fun listTaskExecutions(input: ListTaskExecutionsRequest): ListTaskExecutionsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListTaskExecutionsOperationSerializer()
deserializeWith = ListTaskExecutionsOperationDeserializer()
operationName = "ListTaskExecutions"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of the DataSync tasks you created.
*/
override suspend fun listTasks(input: ListTasksRequest): ListTasksResponse {
val op = SdkHttpOperation.build {
serializeWith = ListTasksOperationSerializer()
deserializeWith = ListTasksOperationDeserializer()
operationName = "ListTasks"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Permanently removes a storage system resource from DataSync Discovery, including the associated discovery jobs, collected data, and recommendations.
*/
override suspend fun removeStorageSystem(input: RemoveStorageSystemRequest): RemoveStorageSystemResponse {
val op = SdkHttpOperation.build {
serializeWith = RemoveStorageSystemOperationSerializer()
deserializeWith = RemoveStorageSystemOperationDeserializer()
operationName = "RemoveStorageSystem"
serviceName = ServiceId
hostPrefix = "discovery-"
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Runs a DataSync discovery job on your on-premises storage system. If you haven't added the storage system to DataSync Discovery yet, do this first by using the [AddStorageSystem](https://docs.aws.amazon.com/datasync/latest/userguide/API_AddStorageSystem.html) operation.
*/
override suspend fun startDiscoveryJob(input: StartDiscoveryJobRequest): StartDiscoveryJobResponse {
val op = SdkHttpOperation.build {
serializeWith = StartDiscoveryJobOperationSerializer()
deserializeWith = StartDiscoveryJobOperationDeserializer()
operationName = "StartDiscoveryJob"
serviceName = ServiceId
hostPrefix = "discovery-"
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Starts an DataSync transfer task. For each task, you can only run one task execution at a time.
*
* There are several phases to a task execution. For more information, see [Task execution statuses](https://docs.aws.amazon.com/datasync/latest/userguide/working-with-task-executions.html#understand-task-execution-statuses).
*
* If you're planning to transfer data to or from an Amazon S3 location, review [how DataSync can affect your S3 request charges](https://docs.aws.amazon.com/datasync/latest/userguide/create-s3-location.html#create-s3-location-s3-requests) and the [DataSync pricing page](http://aws.amazon.com/datasync/pricing/) before you begin.
*/
override suspend fun startTaskExecution(input: StartTaskExecutionRequest): StartTaskExecutionResponse {
val op = SdkHttpOperation.build {
serializeWith = StartTaskExecutionOperationSerializer()
deserializeWith = StartTaskExecutionOperationDeserializer()
operationName = "StartTaskExecution"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Stops a running DataSync discovery job.
*
* You can stop a discovery job anytime. A job that's stopped before it's scheduled to end likely will provide you some information about your on-premises storage system resources. To get recommendations for a stopped job, you must use the [GenerateRecommendations](https://docs.aws.amazon.com/datasync/latest/userguide/API_GenerateRecommendations.html) operation.
*/
override suspend fun stopDiscoveryJob(input: StopDiscoveryJobRequest): StopDiscoveryJobResponse {
val op = SdkHttpOperation.build {
serializeWith = StopDiscoveryJobOperationSerializer()
deserializeWith = StopDiscoveryJobOperationDeserializer()
operationName = "StopDiscoveryJob"
serviceName = ServiceId
hostPrefix = "discovery-"
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Applies a *tag* to an Amazon Web Services resource. Tags are key-value pairs that can help you manage, filter, and search for your resources.
*
* These include DataSync resources, such as locations, tasks, and task executions.
*/
override suspend fun tagResource(input: TagResourceRequest): TagResourceResponse {
val op = SdkHttpOperation.build {
serializeWith = TagResourceOperationSerializer()
deserializeWith = TagResourceOperationDeserializer()
operationName = "TagResource"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Removes tags from an Amazon Web Services resource.
*/
override suspend fun untagResource(input: UntagResourceRequest): UntagResourceResponse {
val op = SdkHttpOperation.build {
serializeWith = UntagResourceOperationSerializer()
deserializeWith = UntagResourceOperationDeserializer()
operationName = "UntagResource"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates the name of an DataSync agent.
*/
override suspend fun updateAgent(input: UpdateAgentRequest): UpdateAgentResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateAgentOperationSerializer()
deserializeWith = UpdateAgentOperationDeserializer()
operationName = "UpdateAgent"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Edits a DataSync discovery job configuration.
*/
override suspend fun updateDiscoveryJob(input: UpdateDiscoveryJobRequest): UpdateDiscoveryJobResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateDiscoveryJobOperationSerializer()
deserializeWith = UpdateDiscoveryJobOperationDeserializer()
operationName = "UpdateDiscoveryJob"
serviceName = ServiceId
hostPrefix = "discovery-"
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Modifies some configurations of the Microsoft Azure Blob Storage transfer location that you're using with DataSync.
*/
override suspend fun updateLocationAzureBlob(input: UpdateLocationAzureBlobRequest): UpdateLocationAzureBlobResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateLocationAzureBlobOperationSerializer()
deserializeWith = UpdateLocationAzureBlobOperationDeserializer()
operationName = "UpdateLocationAzureBlob"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates some parameters of a previously created location for a Hadoop Distributed File System cluster.
*/
override suspend fun updateLocationHdfs(input: UpdateLocationHdfsRequest): UpdateLocationHdfsResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateLocationHdfsOperationSerializer()
deserializeWith = UpdateLocationHdfsOperationDeserializer()
operationName = "UpdateLocationHdfs"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Modifies some configurations of the Network File System (NFS) transfer location that you're using with DataSync.
*
* For more information, see [Configuring transfers to or from an NFS file server](https://docs.aws.amazon.com/datasync/latest/userguide/create-nfs-location.html).
*/
override suspend fun updateLocationNfs(input: UpdateLocationNfsRequest): UpdateLocationNfsResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateLocationNfsOperationSerializer()
deserializeWith = UpdateLocationNfsOperationDeserializer()
operationName = "UpdateLocationNfs"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates some parameters of an existing DataSync location for an object storage system.
*/
override suspend fun updateLocationObjectStorage(input: UpdateLocationObjectStorageRequest): UpdateLocationObjectStorageResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateLocationObjectStorageOperationSerializer()
deserializeWith = UpdateLocationObjectStorageOperationDeserializer()
operationName = "UpdateLocationObjectStorage"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates some of the parameters of a Server Message Block (SMB) file server location that you can use for DataSync transfers.
*/
override suspend fun updateLocationSmb(input: UpdateLocationSmbRequest): UpdateLocationSmbResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateLocationSmbOperationSerializer()
deserializeWith = UpdateLocationSmbOperationDeserializer()
operationName = "UpdateLocationSmb"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Modifies some configurations of an on-premises storage system resource that you're using with DataSync Discovery.
*/
override suspend fun updateStorageSystem(input: UpdateStorageSystemRequest): UpdateStorageSystemResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateStorageSystemOperationSerializer()
deserializeWith = UpdateStorageSystemOperationDeserializer()
operationName = "UpdateStorageSystem"
serviceName = ServiceId
hostPrefix = "discovery-"
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates the configuration of a *task*, which defines where and how DataSync transfers your data.
*/
override suspend fun updateTask(input: UpdateTaskRequest): UpdateTaskResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateTaskOperationSerializer()
deserializeWith = UpdateTaskOperationDeserializer()
operationName = "UpdateTask"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates the configuration of a running DataSync task execution.
*
* Currently, the only `Option` that you can modify with `UpdateTaskExecution` is ` BytesPerSecond `, which throttles bandwidth for a running or queued task execution.
*/
override suspend fun updateTaskExecution(input: UpdateTaskExecutionRequest): UpdateTaskExecutionResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateTaskExecutionOperationSerializer()
deserializeWith = UpdateTaskExecutionOperationDeserializer()
operationName = "UpdateTaskExecution"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(AwsJsonProtocol("FmrsService", "1.1"))
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
override fun close() {
managedResources.unshareAll()
}
/**
* merge the defaults configured for the service into the execution context before firing off a request
*/
private fun mergeServiceDefaults(ctx: ExecutionContext) {
ctx.putIfAbsent(SdkClientOption.ClientName, config.clientName)
ctx.putIfAbsent(SdkClientOption.LogMode, config.logMode)
ctx.putIfAbsentNotNull(SdkClientOption.IdempotencyTokenProvider, config.idempotencyTokenProvider)
ctx.putIfAbsentNotNull(AwsAttributes.Region, config.region)
ctx.putIfAbsentNotNull(AwsSigningAttributes.SigningRegion, config.region)
ctx.putIfAbsent(AwsSigningAttributes.SigningService, "datasync")
ctx.putIfAbsent(AwsSigningAttributes.CredentialsProvider, config.credentialsProvider)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy