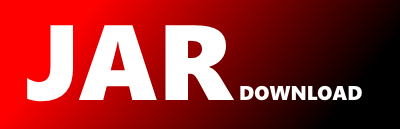
commonMain.aws.sdk.kotlin.services.datasync.model.CreateLocationEfsRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datasync-jvm Show documentation
Show all versions of datasync-jvm Show documentation
The AWS SDK for Kotlin client for DataSync
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* CreateLocationEfsRequest
*/
public class CreateLocationEfsRequest private constructor(builder: Builder) {
/**
* Specifies the Amazon Resource Name (ARN) of the access point that DataSync uses to access the Amazon EFS file system.
*/
public val accessPointArn: kotlin.String? = builder.accessPointArn
/**
* Specifies the subnet and security groups DataSync uses to access your Amazon EFS file system.
*/
public val ec2Config: aws.sdk.kotlin.services.datasync.model.Ec2Config? = builder.ec2Config
/**
* Specifies the ARN for the Amazon EFS file system.
*/
public val efsFilesystemArn: kotlin.String? = builder.efsFilesystemArn
/**
* Specifies an Identity and Access Management (IAM) role that DataSync assumes when mounting the Amazon EFS file system.
*/
public val fileSystemAccessRoleArn: kotlin.String? = builder.fileSystemAccessRoleArn
/**
* Specifies whether you want DataSync to use Transport Layer Security (TLS) 1.2 encryption when it copies data to or from the Amazon EFS file system.
*
* If you specify an access point using `AccessPointArn` or an IAM role using `FileSystemAccessRoleArn`, you must set this parameter to `TLS1_2`.
*/
public val inTransitEncryption: aws.sdk.kotlin.services.datasync.model.EfsInTransitEncryption? = builder.inTransitEncryption
/**
* Specifies a mount path for your Amazon EFS file system. This is where DataSync reads or writes data (depending on if this is a source or destination location). By default, DataSync uses the root directory, but you can also include subdirectories.
*
* You must specify a value with forward slashes (for example, `/path/to/folder`).
*/
public val subdirectory: kotlin.String? = builder.subdirectory
/**
* Specifies the key-value pair that represents a tag that you want to add to the resource. The value can be an empty string. This value helps you manage, filter, and search for your resources. We recommend that you create a name tag for your location.
*/
public val tags: List? = builder.tags
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.CreateLocationEfsRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateLocationEfsRequest(")
append("accessPointArn=$accessPointArn,")
append("ec2Config=$ec2Config,")
append("efsFilesystemArn=$efsFilesystemArn,")
append("fileSystemAccessRoleArn=$fileSystemAccessRoleArn,")
append("inTransitEncryption=$inTransitEncryption,")
append("subdirectory=$subdirectory,")
append("tags=$tags")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = accessPointArn?.hashCode() ?: 0
result = 31 * result + (ec2Config?.hashCode() ?: 0)
result = 31 * result + (efsFilesystemArn?.hashCode() ?: 0)
result = 31 * result + (fileSystemAccessRoleArn?.hashCode() ?: 0)
result = 31 * result + (inTransitEncryption?.hashCode() ?: 0)
result = 31 * result + (subdirectory?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateLocationEfsRequest
if (accessPointArn != other.accessPointArn) return false
if (ec2Config != other.ec2Config) return false
if (efsFilesystemArn != other.efsFilesystemArn) return false
if (fileSystemAccessRoleArn != other.fileSystemAccessRoleArn) return false
if (inTransitEncryption != other.inTransitEncryption) return false
if (subdirectory != other.subdirectory) return false
if (tags != other.tags) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.CreateLocationEfsRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Specifies the Amazon Resource Name (ARN) of the access point that DataSync uses to access the Amazon EFS file system.
*/
public var accessPointArn: kotlin.String? = null
/**
* Specifies the subnet and security groups DataSync uses to access your Amazon EFS file system.
*/
public var ec2Config: aws.sdk.kotlin.services.datasync.model.Ec2Config? = null
/**
* Specifies the ARN for the Amazon EFS file system.
*/
public var efsFilesystemArn: kotlin.String? = null
/**
* Specifies an Identity and Access Management (IAM) role that DataSync assumes when mounting the Amazon EFS file system.
*/
public var fileSystemAccessRoleArn: kotlin.String? = null
/**
* Specifies whether you want DataSync to use Transport Layer Security (TLS) 1.2 encryption when it copies data to or from the Amazon EFS file system.
*
* If you specify an access point using `AccessPointArn` or an IAM role using `FileSystemAccessRoleArn`, you must set this parameter to `TLS1_2`.
*/
public var inTransitEncryption: aws.sdk.kotlin.services.datasync.model.EfsInTransitEncryption? = null
/**
* Specifies a mount path for your Amazon EFS file system. This is where DataSync reads or writes data (depending on if this is a source or destination location). By default, DataSync uses the root directory, but you can also include subdirectories.
*
* You must specify a value with forward slashes (for example, `/path/to/folder`).
*/
public var subdirectory: kotlin.String? = null
/**
* Specifies the key-value pair that represents a tag that you want to add to the resource. The value can be an empty string. This value helps you manage, filter, and search for your resources. We recommend that you create a name tag for your location.
*/
public var tags: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.CreateLocationEfsRequest) : this() {
this.accessPointArn = x.accessPointArn
this.ec2Config = x.ec2Config
this.efsFilesystemArn = x.efsFilesystemArn
this.fileSystemAccessRoleArn = x.fileSystemAccessRoleArn
this.inTransitEncryption = x.inTransitEncryption
this.subdirectory = x.subdirectory
this.tags = x.tags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.CreateLocationEfsRequest = CreateLocationEfsRequest(this)
/**
* construct an [aws.sdk.kotlin.services.datasync.model.Ec2Config] inside the given [block]
*/
public fun ec2Config(block: aws.sdk.kotlin.services.datasync.model.Ec2Config.Builder.() -> kotlin.Unit) {
this.ec2Config = aws.sdk.kotlin.services.datasync.model.Ec2Config.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy