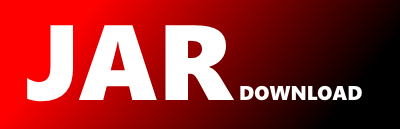
commonMain.aws.sdk.kotlin.services.datasync.model.CreateLocationSmbRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datasync-jvm Show documentation
Show all versions of datasync-jvm Show documentation
The AWS SDK for Kotlin client for DataSync
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* CreateLocationSmbRequest
*/
public class CreateLocationSmbRequest private constructor(builder: Builder) {
/**
* Specifies the DataSync agent (or agents) which you want to connect to your SMB file server. You specify an agent by using its Amazon Resource Name (ARN).
*/
public val agentArns: List? = builder.agentArns
/**
* Specifies the name of the Active Directory domain that your SMB file server belongs to.
*
* If you have multiple Active Directory domains in your environment, configuring this parameter makes sure that DataSync connects to the right file server.
*/
public val domain: kotlin.String? = builder.domain
/**
* Specifies the version of the SMB protocol that DataSync uses to access your SMB file server.
*/
public val mountOptions: aws.sdk.kotlin.services.datasync.model.SmbMountOptions? = builder.mountOptions
/**
* Specifies the password of the user who can mount your SMB file server and has permission to access the files and folders involved in your transfer.
*
* For more information, see [required permissions](https://docs.aws.amazon.com/datasync/latest/userguide/create-smb-location.html#configuring-smb-permissions) for SMB locations.
*/
public val password: kotlin.String? = builder.password
/**
* Specifies the Domain Name Service (DNS) name or IP address of the SMB file server that your DataSync agent will mount.
*
* You can't specify an IP version 6 (IPv6) address.
*/
public val serverHostname: kotlin.String? = builder.serverHostname
/**
* Specifies the name of the share exported by your SMB file server where DataSync will read or write data. You can include a subdirectory in the share path (for example, `/path/to/subdirectory`). Make sure that other SMB clients in your network can also mount this path.
*
* To copy all data in the subdirectory, DataSync must be able to mount the SMB share and access all of its data. For more information, see [required permissions](https://docs.aws.amazon.com/datasync/latest/userguide/create-smb-location.html#configuring-smb-permissions) for SMB locations.
*/
public val subdirectory: kotlin.String? = builder.subdirectory
/**
* Specifies labels that help you categorize, filter, and search for your Amazon Web Services resources. We recommend creating at least a name tag for your location.
*/
public val tags: List? = builder.tags
/**
* Specifies the user that can mount and access the files, folders, and file metadata in your SMB file server.
*
* For information about choosing a user with the right level of access for your transfer, see [required permissions](https://docs.aws.amazon.com/datasync/latest/userguide/create-smb-location.html#configuring-smb-permissions) for SMB locations.
*/
public val user: kotlin.String? = builder.user
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.CreateLocationSmbRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateLocationSmbRequest(")
append("agentArns=$agentArns,")
append("domain=$domain,")
append("mountOptions=$mountOptions,")
append("password=*** Sensitive Data Redacted ***,")
append("serverHostname=$serverHostname,")
append("subdirectory=$subdirectory,")
append("tags=$tags,")
append("user=$user")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = agentArns?.hashCode() ?: 0
result = 31 * result + (domain?.hashCode() ?: 0)
result = 31 * result + (mountOptions?.hashCode() ?: 0)
result = 31 * result + (password?.hashCode() ?: 0)
result = 31 * result + (serverHostname?.hashCode() ?: 0)
result = 31 * result + (subdirectory?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (user?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateLocationSmbRequest
if (agentArns != other.agentArns) return false
if (domain != other.domain) return false
if (mountOptions != other.mountOptions) return false
if (password != other.password) return false
if (serverHostname != other.serverHostname) return false
if (subdirectory != other.subdirectory) return false
if (tags != other.tags) return false
if (user != other.user) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.CreateLocationSmbRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Specifies the DataSync agent (or agents) which you want to connect to your SMB file server. You specify an agent by using its Amazon Resource Name (ARN).
*/
public var agentArns: List? = null
/**
* Specifies the name of the Active Directory domain that your SMB file server belongs to.
*
* If you have multiple Active Directory domains in your environment, configuring this parameter makes sure that DataSync connects to the right file server.
*/
public var domain: kotlin.String? = null
/**
* Specifies the version of the SMB protocol that DataSync uses to access your SMB file server.
*/
public var mountOptions: aws.sdk.kotlin.services.datasync.model.SmbMountOptions? = null
/**
* Specifies the password of the user who can mount your SMB file server and has permission to access the files and folders involved in your transfer.
*
* For more information, see [required permissions](https://docs.aws.amazon.com/datasync/latest/userguide/create-smb-location.html#configuring-smb-permissions) for SMB locations.
*/
public var password: kotlin.String? = null
/**
* Specifies the Domain Name Service (DNS) name or IP address of the SMB file server that your DataSync agent will mount.
*
* You can't specify an IP version 6 (IPv6) address.
*/
public var serverHostname: kotlin.String? = null
/**
* Specifies the name of the share exported by your SMB file server where DataSync will read or write data. You can include a subdirectory in the share path (for example, `/path/to/subdirectory`). Make sure that other SMB clients in your network can also mount this path.
*
* To copy all data in the subdirectory, DataSync must be able to mount the SMB share and access all of its data. For more information, see [required permissions](https://docs.aws.amazon.com/datasync/latest/userguide/create-smb-location.html#configuring-smb-permissions) for SMB locations.
*/
public var subdirectory: kotlin.String? = null
/**
* Specifies labels that help you categorize, filter, and search for your Amazon Web Services resources. We recommend creating at least a name tag for your location.
*/
public var tags: List? = null
/**
* Specifies the user that can mount and access the files, folders, and file metadata in your SMB file server.
*
* For information about choosing a user with the right level of access for your transfer, see [required permissions](https://docs.aws.amazon.com/datasync/latest/userguide/create-smb-location.html#configuring-smb-permissions) for SMB locations.
*/
public var user: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.CreateLocationSmbRequest) : this() {
this.agentArns = x.agentArns
this.domain = x.domain
this.mountOptions = x.mountOptions
this.password = x.password
this.serverHostname = x.serverHostname
this.subdirectory = x.subdirectory
this.tags = x.tags
this.user = x.user
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.CreateLocationSmbRequest = CreateLocationSmbRequest(this)
/**
* construct an [aws.sdk.kotlin.services.datasync.model.SmbMountOptions] inside the given [block]
*/
public fun mountOptions(block: aws.sdk.kotlin.services.datasync.model.SmbMountOptions.Builder.() -> kotlin.Unit) {
this.mountOptions = aws.sdk.kotlin.services.datasync.model.SmbMountOptions.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy