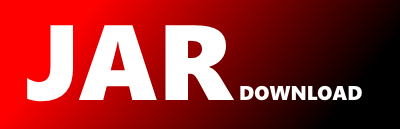
commonMain.aws.sdk.kotlin.services.datasync.model.DescribeLocationEfsResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datasync-jvm Show documentation
Show all versions of datasync-jvm Show documentation
The AWS SDK for Kotlin client for DataSync
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* DescribeLocationEfsResponse
*/
public class DescribeLocationEfsResponse private constructor(builder: Builder) {
/**
* The ARN of the access point that DataSync uses to access the Amazon EFS file system.
*/
public val accessPointArn: kotlin.String? = builder.accessPointArn
/**
* The time that the location was created.
*/
public val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* The subnet and security groups that DataSync uses to access your Amazon EFS file system.
*/
public val ec2Config: aws.sdk.kotlin.services.datasync.model.Ec2Config? = builder.ec2Config
/**
* The Identity and Access Management (IAM) role that DataSync assumes when mounting the Amazon EFS file system.
*/
public val fileSystemAccessRoleArn: kotlin.String? = builder.fileSystemAccessRoleArn
/**
* Describes whether DataSync uses Transport Layer Security (TLS) encryption when copying data to or from the Amazon EFS file system.
*/
public val inTransitEncryption: aws.sdk.kotlin.services.datasync.model.EfsInTransitEncryption? = builder.inTransitEncryption
/**
* The ARN of the Amazon EFS file system location.
*/
public val locationArn: kotlin.String? = builder.locationArn
/**
* The URL of the Amazon EFS file system location.
*/
public val locationUri: kotlin.String? = builder.locationUri
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.DescribeLocationEfsResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeLocationEfsResponse(")
append("accessPointArn=$accessPointArn,")
append("creationTime=$creationTime,")
append("ec2Config=$ec2Config,")
append("fileSystemAccessRoleArn=$fileSystemAccessRoleArn,")
append("inTransitEncryption=$inTransitEncryption,")
append("locationArn=$locationArn,")
append("locationUri=$locationUri")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = accessPointArn?.hashCode() ?: 0
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (ec2Config?.hashCode() ?: 0)
result = 31 * result + (fileSystemAccessRoleArn?.hashCode() ?: 0)
result = 31 * result + (inTransitEncryption?.hashCode() ?: 0)
result = 31 * result + (locationArn?.hashCode() ?: 0)
result = 31 * result + (locationUri?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeLocationEfsResponse
if (accessPointArn != other.accessPointArn) return false
if (creationTime != other.creationTime) return false
if (ec2Config != other.ec2Config) return false
if (fileSystemAccessRoleArn != other.fileSystemAccessRoleArn) return false
if (inTransitEncryption != other.inTransitEncryption) return false
if (locationArn != other.locationArn) return false
if (locationUri != other.locationUri) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.DescribeLocationEfsResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ARN of the access point that DataSync uses to access the Amazon EFS file system.
*/
public var accessPointArn: kotlin.String? = null
/**
* The time that the location was created.
*/
public var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The subnet and security groups that DataSync uses to access your Amazon EFS file system.
*/
public var ec2Config: aws.sdk.kotlin.services.datasync.model.Ec2Config? = null
/**
* The Identity and Access Management (IAM) role that DataSync assumes when mounting the Amazon EFS file system.
*/
public var fileSystemAccessRoleArn: kotlin.String? = null
/**
* Describes whether DataSync uses Transport Layer Security (TLS) encryption when copying data to or from the Amazon EFS file system.
*/
public var inTransitEncryption: aws.sdk.kotlin.services.datasync.model.EfsInTransitEncryption? = null
/**
* The ARN of the Amazon EFS file system location.
*/
public var locationArn: kotlin.String? = null
/**
* The URL of the Amazon EFS file system location.
*/
public var locationUri: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.DescribeLocationEfsResponse) : this() {
this.accessPointArn = x.accessPointArn
this.creationTime = x.creationTime
this.ec2Config = x.ec2Config
this.fileSystemAccessRoleArn = x.fileSystemAccessRoleArn
this.inTransitEncryption = x.inTransitEncryption
this.locationArn = x.locationArn
this.locationUri = x.locationUri
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.DescribeLocationEfsResponse = DescribeLocationEfsResponse(this)
/**
* construct an [aws.sdk.kotlin.services.datasync.model.Ec2Config] inside the given [block]
*/
public fun ec2Config(block: aws.sdk.kotlin.services.datasync.model.Ec2Config.Builder.() -> kotlin.Unit) {
this.ec2Config = aws.sdk.kotlin.services.datasync.model.Ec2Config.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy