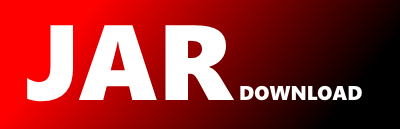
commonMain.aws.sdk.kotlin.services.datasync.model.DescribeLocationHdfsResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datasync-jvm Show documentation
Show all versions of datasync-jvm Show documentation
The AWS SDK for Kotlin client for DataSync
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
public class DescribeLocationHdfsResponse private constructor(builder: Builder) {
/**
* The ARNs of the DataSync agents that can connect with your HDFS cluster.
*/
public val agentArns: List? = builder.agentArns
/**
* The type of authentication used to determine the identity of the user.
*/
public val authenticationType: aws.sdk.kotlin.services.datasync.model.HdfsAuthenticationType? = builder.authenticationType
/**
* The size of the data blocks to write into the HDFS cluster.
*/
public val blockSize: kotlin.Int? = builder.blockSize
/**
* The time that the HDFS location was created.
*/
public val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* The Kerberos principal with access to the files and folders on the HDFS cluster. This parameter is used if the `AuthenticationType` is defined as `KERBEROS`.
*/
public val kerberosPrincipal: kotlin.String? = builder.kerberosPrincipal
/**
* The URI of the HDFS cluster's Key Management Server (KMS).
*/
public val kmsKeyProviderUri: kotlin.String? = builder.kmsKeyProviderUri
/**
* The ARN of the HDFS location.
*/
public val locationArn: kotlin.String? = builder.locationArn
/**
* The URI of the HDFS location.
*/
public val locationUri: kotlin.String? = builder.locationUri
/**
* The NameNode that manages the HDFS namespace.
*/
public val nameNodes: List? = builder.nameNodes
/**
* The Quality of Protection (QOP) configuration, which specifies the Remote Procedure Call (RPC) and data transfer protection settings configured on the HDFS cluster.
*/
public val qopConfiguration: aws.sdk.kotlin.services.datasync.model.QopConfiguration? = builder.qopConfiguration
/**
* The number of DataNodes to replicate the data to when writing to the HDFS cluster.
*/
public val replicationFactor: kotlin.Int? = builder.replicationFactor
/**
* The user name to identify the client on the host operating system. This parameter is used if the `AuthenticationType` is defined as `SIMPLE`.
*/
public val simpleUser: kotlin.String? = builder.simpleUser
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.DescribeLocationHdfsResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeLocationHdfsResponse(")
append("agentArns=$agentArns,")
append("authenticationType=$authenticationType,")
append("blockSize=$blockSize,")
append("creationTime=$creationTime,")
append("kerberosPrincipal=$kerberosPrincipal,")
append("kmsKeyProviderUri=$kmsKeyProviderUri,")
append("locationArn=$locationArn,")
append("locationUri=$locationUri,")
append("nameNodes=$nameNodes,")
append("qopConfiguration=$qopConfiguration,")
append("replicationFactor=$replicationFactor,")
append("simpleUser=$simpleUser")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = agentArns?.hashCode() ?: 0
result = 31 * result + (authenticationType?.hashCode() ?: 0)
result = 31 * result + (blockSize ?: 0)
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (kerberosPrincipal?.hashCode() ?: 0)
result = 31 * result + (kmsKeyProviderUri?.hashCode() ?: 0)
result = 31 * result + (locationArn?.hashCode() ?: 0)
result = 31 * result + (locationUri?.hashCode() ?: 0)
result = 31 * result + (nameNodes?.hashCode() ?: 0)
result = 31 * result + (qopConfiguration?.hashCode() ?: 0)
result = 31 * result + (replicationFactor ?: 0)
result = 31 * result + (simpleUser?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeLocationHdfsResponse
if (agentArns != other.agentArns) return false
if (authenticationType != other.authenticationType) return false
if (blockSize != other.blockSize) return false
if (creationTime != other.creationTime) return false
if (kerberosPrincipal != other.kerberosPrincipal) return false
if (kmsKeyProviderUri != other.kmsKeyProviderUri) return false
if (locationArn != other.locationArn) return false
if (locationUri != other.locationUri) return false
if (nameNodes != other.nameNodes) return false
if (qopConfiguration != other.qopConfiguration) return false
if (replicationFactor != other.replicationFactor) return false
if (simpleUser != other.simpleUser) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.DescribeLocationHdfsResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ARNs of the DataSync agents that can connect with your HDFS cluster.
*/
public var agentArns: List? = null
/**
* The type of authentication used to determine the identity of the user.
*/
public var authenticationType: aws.sdk.kotlin.services.datasync.model.HdfsAuthenticationType? = null
/**
* The size of the data blocks to write into the HDFS cluster.
*/
public var blockSize: kotlin.Int? = null
/**
* The time that the HDFS location was created.
*/
public var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The Kerberos principal with access to the files and folders on the HDFS cluster. This parameter is used if the `AuthenticationType` is defined as `KERBEROS`.
*/
public var kerberosPrincipal: kotlin.String? = null
/**
* The URI of the HDFS cluster's Key Management Server (KMS).
*/
public var kmsKeyProviderUri: kotlin.String? = null
/**
* The ARN of the HDFS location.
*/
public var locationArn: kotlin.String? = null
/**
* The URI of the HDFS location.
*/
public var locationUri: kotlin.String? = null
/**
* The NameNode that manages the HDFS namespace.
*/
public var nameNodes: List? = null
/**
* The Quality of Protection (QOP) configuration, which specifies the Remote Procedure Call (RPC) and data transfer protection settings configured on the HDFS cluster.
*/
public var qopConfiguration: aws.sdk.kotlin.services.datasync.model.QopConfiguration? = null
/**
* The number of DataNodes to replicate the data to when writing to the HDFS cluster.
*/
public var replicationFactor: kotlin.Int? = null
/**
* The user name to identify the client on the host operating system. This parameter is used if the `AuthenticationType` is defined as `SIMPLE`.
*/
public var simpleUser: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.DescribeLocationHdfsResponse) : this() {
this.agentArns = x.agentArns
this.authenticationType = x.authenticationType
this.blockSize = x.blockSize
this.creationTime = x.creationTime
this.kerberosPrincipal = x.kerberosPrincipal
this.kmsKeyProviderUri = x.kmsKeyProviderUri
this.locationArn = x.locationArn
this.locationUri = x.locationUri
this.nameNodes = x.nameNodes
this.qopConfiguration = x.qopConfiguration
this.replicationFactor = x.replicationFactor
this.simpleUser = x.simpleUser
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.DescribeLocationHdfsResponse = DescribeLocationHdfsResponse(this)
/**
* construct an [aws.sdk.kotlin.services.datasync.model.QopConfiguration] inside the given [block]
*/
public fun qopConfiguration(block: aws.sdk.kotlin.services.datasync.model.QopConfiguration.Builder.() -> kotlin.Unit) {
this.qopConfiguration = aws.sdk.kotlin.services.datasync.model.QopConfiguration.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy