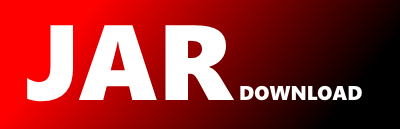
commonMain.aws.sdk.kotlin.services.datasync.model.DescribeLocationSmbResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datasync-jvm Show documentation
Show all versions of datasync-jvm Show documentation
The AWS SDK for Kotlin client for DataSync
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* DescribeLocationSmbResponse
*/
public class DescribeLocationSmbResponse private constructor(builder: Builder) {
/**
* The ARNs of the DataSync agents that can connect with your SMB file server.
*/
public val agentArns: List? = builder.agentArns
/**
* The time that the SMB location was created.
*/
public val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* The name of the Microsoft Active Directory domain that the SMB file server belongs to.
*/
public val domain: kotlin.String? = builder.domain
/**
* The ARN of the SMB location.
*/
public val locationArn: kotlin.String? = builder.locationArn
/**
* The URI of the SMB location.
*/
public val locationUri: kotlin.String? = builder.locationUri
/**
* The protocol that DataSync use to access your SMB file.
*/
public val mountOptions: aws.sdk.kotlin.services.datasync.model.SmbMountOptions? = builder.mountOptions
/**
* The user that can mount and access the files, folders, and file metadata in your SMB file server.
*/
public val user: kotlin.String? = builder.user
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.DescribeLocationSmbResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeLocationSmbResponse(")
append("agentArns=$agentArns,")
append("creationTime=$creationTime,")
append("domain=$domain,")
append("locationArn=$locationArn,")
append("locationUri=$locationUri,")
append("mountOptions=$mountOptions,")
append("user=$user")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = agentArns?.hashCode() ?: 0
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (domain?.hashCode() ?: 0)
result = 31 * result + (locationArn?.hashCode() ?: 0)
result = 31 * result + (locationUri?.hashCode() ?: 0)
result = 31 * result + (mountOptions?.hashCode() ?: 0)
result = 31 * result + (user?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeLocationSmbResponse
if (agentArns != other.agentArns) return false
if (creationTime != other.creationTime) return false
if (domain != other.domain) return false
if (locationArn != other.locationArn) return false
if (locationUri != other.locationUri) return false
if (mountOptions != other.mountOptions) return false
if (user != other.user) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.DescribeLocationSmbResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ARNs of the DataSync agents that can connect with your SMB file server.
*/
public var agentArns: List? = null
/**
* The time that the SMB location was created.
*/
public var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The name of the Microsoft Active Directory domain that the SMB file server belongs to.
*/
public var domain: kotlin.String? = null
/**
* The ARN of the SMB location.
*/
public var locationArn: kotlin.String? = null
/**
* The URI of the SMB location.
*/
public var locationUri: kotlin.String? = null
/**
* The protocol that DataSync use to access your SMB file.
*/
public var mountOptions: aws.sdk.kotlin.services.datasync.model.SmbMountOptions? = null
/**
* The user that can mount and access the files, folders, and file metadata in your SMB file server.
*/
public var user: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.DescribeLocationSmbResponse) : this() {
this.agentArns = x.agentArns
this.creationTime = x.creationTime
this.domain = x.domain
this.locationArn = x.locationArn
this.locationUri = x.locationUri
this.mountOptions = x.mountOptions
this.user = x.user
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.DescribeLocationSmbResponse = DescribeLocationSmbResponse(this)
/**
* construct an [aws.sdk.kotlin.services.datasync.model.SmbMountOptions] inside the given [block]
*/
public fun mountOptions(block: aws.sdk.kotlin.services.datasync.model.SmbMountOptions.Builder.() -> kotlin.Unit) {
this.mountOptions = aws.sdk.kotlin.services.datasync.model.SmbMountOptions.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy