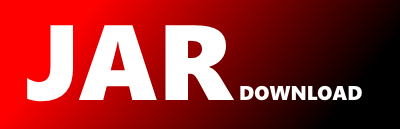
commonMain.aws.sdk.kotlin.services.datasync.model.DescribeTaskResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datasync-jvm Show documentation
Show all versions of datasync-jvm Show documentation
The AWS SDK for Kotlin client for DataSync
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* DescribeTaskResponse
*/
public class DescribeTaskResponse private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) of an Amazon CloudWatch log group for monitoring your task.
*
* For more information, see [Monitoring DataSync with Amazon CloudWatch](https://docs.aws.amazon.com/datasync/latest/userguide/monitor-datasync.html).
*/
public val cloudWatchLogGroupArn: kotlin.String? = builder.cloudWatchLogGroupArn
/**
* The time that the task was created.
*/
public val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* The ARN of the most recent task execution.
*/
public val currentTaskExecutionArn: kotlin.String? = builder.currentTaskExecutionArn
/**
* The ARN of your transfer's destination location.
*/
public val destinationLocationArn: kotlin.String? = builder.destinationLocationArn
/**
* The ARNs of the [network interfaces](https://docs.aws.amazon.com/datasync/latest/userguide/datasync-network.html#required-network-interfaces) that DataSync created for your destination location.
*/
public val destinationNetworkInterfaceArns: List? = builder.destinationNetworkInterfaceArns
/**
* If there's an issue with your task, you can use the error code to help you troubleshoot the problem. For more information, see [Troubleshooting issues with DataSync transfers](https://docs.aws.amazon.com/datasync/latest/userguide/troubleshooting-datasync-locations-tasks.html).
*/
public val errorCode: kotlin.String? = builder.errorCode
/**
* If there's an issue with your task, you can use the error details to help you troubleshoot the problem. For more information, see [Troubleshooting issues with DataSync transfers](https://docs.aws.amazon.com/datasync/latest/userguide/troubleshooting-datasync-locations-tasks.html).
*/
public val errorDetail: kotlin.String? = builder.errorDetail
/**
* The exclude filters that define the files, objects, and folders in your source location that you don't want DataSync to transfer. For more information and examples, see [Specifying what DataSync transfers by using filters](https://docs.aws.amazon.com/datasync/latest/userguide/filtering.html).
*/
public val excludes: List? = builder.excludes
/**
* The include filters that define the files, objects, and folders in your source location that you want DataSync to transfer. For more information and examples, see [Specifying what DataSync transfers by using filters](https://docs.aws.amazon.com/datasync/latest/userguide/filtering.html).
*/
public val includes: List? = builder.includes
/**
* The configuration of the manifest that lists the files or objects that you want DataSync to transfer. For more information, see [Specifying what DataSync transfers by using a manifest](https://docs.aws.amazon.com/datasync/latest/userguide/transferring-with-manifest.html).
*/
public val manifestConfig: aws.sdk.kotlin.services.datasync.model.ManifestConfig? = builder.manifestConfig
/**
* The name of your task.
*/
public val name: kotlin.String? = builder.name
/**
* The task's settings. For example, what file metadata gets preserved, how data integrity gets verified at the end of your transfer, bandwidth limits, among other options.
*/
public val options: aws.sdk.kotlin.services.datasync.model.Options? = builder.options
/**
* The schedule for when you want your task to run. For more information, see [Scheduling your task](https://docs.aws.amazon.com/datasync/latest/userguide/task-scheduling.html).
*/
public val schedule: aws.sdk.kotlin.services.datasync.model.TaskSchedule? = builder.schedule
/**
* The details about your [task schedule](https://docs.aws.amazon.com/datasync/latest/userguide/task-scheduling.html).
*/
public val scheduleDetails: aws.sdk.kotlin.services.datasync.model.TaskScheduleDetails? = builder.scheduleDetails
/**
* The ARN of your transfer's source location.
*/
public val sourceLocationArn: kotlin.String? = builder.sourceLocationArn
/**
* The ARNs of the [network interfaces](https://docs.aws.amazon.com/datasync/latest/userguide/datasync-network.html#required-network-interfaces) that DataSync created for your source location.
*/
public val sourceNetworkInterfaceArns: List? = builder.sourceNetworkInterfaceArns
/**
* The status of your task. For information about what each status means, see [Task statuses](https://docs.aws.amazon.com/datasync/latest/userguide/understand-task-statuses.html#understand-task-creation-statuses).
*/
public val status: aws.sdk.kotlin.services.datasync.model.TaskStatus? = builder.status
/**
* The ARN of your task.
*/
public val taskArn: kotlin.String? = builder.taskArn
/**
* The configuration of your task report, which provides detailed information about your DataSync transfer. For more information, see [Monitoring your DataSync transfers with task reports](https://docs.aws.amazon.com/datasync/latest/userguide/task-reports.html).
*/
public val taskReportConfig: aws.sdk.kotlin.services.datasync.model.TaskReportConfig? = builder.taskReportConfig
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.DescribeTaskResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeTaskResponse(")
append("cloudWatchLogGroupArn=$cloudWatchLogGroupArn,")
append("creationTime=$creationTime,")
append("currentTaskExecutionArn=$currentTaskExecutionArn,")
append("destinationLocationArn=$destinationLocationArn,")
append("destinationNetworkInterfaceArns=$destinationNetworkInterfaceArns,")
append("errorCode=$errorCode,")
append("errorDetail=$errorDetail,")
append("excludes=$excludes,")
append("includes=$includes,")
append("manifestConfig=$manifestConfig,")
append("name=$name,")
append("options=$options,")
append("schedule=$schedule,")
append("scheduleDetails=$scheduleDetails,")
append("sourceLocationArn=$sourceLocationArn,")
append("sourceNetworkInterfaceArns=$sourceNetworkInterfaceArns,")
append("status=$status,")
append("taskArn=$taskArn,")
append("taskReportConfig=$taskReportConfig")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = cloudWatchLogGroupArn?.hashCode() ?: 0
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (currentTaskExecutionArn?.hashCode() ?: 0)
result = 31 * result + (destinationLocationArn?.hashCode() ?: 0)
result = 31 * result + (destinationNetworkInterfaceArns?.hashCode() ?: 0)
result = 31 * result + (errorCode?.hashCode() ?: 0)
result = 31 * result + (errorDetail?.hashCode() ?: 0)
result = 31 * result + (excludes?.hashCode() ?: 0)
result = 31 * result + (includes?.hashCode() ?: 0)
result = 31 * result + (manifestConfig?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (options?.hashCode() ?: 0)
result = 31 * result + (schedule?.hashCode() ?: 0)
result = 31 * result + (scheduleDetails?.hashCode() ?: 0)
result = 31 * result + (sourceLocationArn?.hashCode() ?: 0)
result = 31 * result + (sourceNetworkInterfaceArns?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (taskArn?.hashCode() ?: 0)
result = 31 * result + (taskReportConfig?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeTaskResponse
if (cloudWatchLogGroupArn != other.cloudWatchLogGroupArn) return false
if (creationTime != other.creationTime) return false
if (currentTaskExecutionArn != other.currentTaskExecutionArn) return false
if (destinationLocationArn != other.destinationLocationArn) return false
if (destinationNetworkInterfaceArns != other.destinationNetworkInterfaceArns) return false
if (errorCode != other.errorCode) return false
if (errorDetail != other.errorDetail) return false
if (excludes != other.excludes) return false
if (includes != other.includes) return false
if (manifestConfig != other.manifestConfig) return false
if (name != other.name) return false
if (options != other.options) return false
if (schedule != other.schedule) return false
if (scheduleDetails != other.scheduleDetails) return false
if (sourceLocationArn != other.sourceLocationArn) return false
if (sourceNetworkInterfaceArns != other.sourceNetworkInterfaceArns) return false
if (status != other.status) return false
if (taskArn != other.taskArn) return false
if (taskReportConfig != other.taskReportConfig) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.DescribeTaskResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The Amazon Resource Name (ARN) of an Amazon CloudWatch log group for monitoring your task.
*
* For more information, see [Monitoring DataSync with Amazon CloudWatch](https://docs.aws.amazon.com/datasync/latest/userguide/monitor-datasync.html).
*/
public var cloudWatchLogGroupArn: kotlin.String? = null
/**
* The time that the task was created.
*/
public var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ARN of the most recent task execution.
*/
public var currentTaskExecutionArn: kotlin.String? = null
/**
* The ARN of your transfer's destination location.
*/
public var destinationLocationArn: kotlin.String? = null
/**
* The ARNs of the [network interfaces](https://docs.aws.amazon.com/datasync/latest/userguide/datasync-network.html#required-network-interfaces) that DataSync created for your destination location.
*/
public var destinationNetworkInterfaceArns: List? = null
/**
* If there's an issue with your task, you can use the error code to help you troubleshoot the problem. For more information, see [Troubleshooting issues with DataSync transfers](https://docs.aws.amazon.com/datasync/latest/userguide/troubleshooting-datasync-locations-tasks.html).
*/
public var errorCode: kotlin.String? = null
/**
* If there's an issue with your task, you can use the error details to help you troubleshoot the problem. For more information, see [Troubleshooting issues with DataSync transfers](https://docs.aws.amazon.com/datasync/latest/userguide/troubleshooting-datasync-locations-tasks.html).
*/
public var errorDetail: kotlin.String? = null
/**
* The exclude filters that define the files, objects, and folders in your source location that you don't want DataSync to transfer. For more information and examples, see [Specifying what DataSync transfers by using filters](https://docs.aws.amazon.com/datasync/latest/userguide/filtering.html).
*/
public var excludes: List? = null
/**
* The include filters that define the files, objects, and folders in your source location that you want DataSync to transfer. For more information and examples, see [Specifying what DataSync transfers by using filters](https://docs.aws.amazon.com/datasync/latest/userguide/filtering.html).
*/
public var includes: List? = null
/**
* The configuration of the manifest that lists the files or objects that you want DataSync to transfer. For more information, see [Specifying what DataSync transfers by using a manifest](https://docs.aws.amazon.com/datasync/latest/userguide/transferring-with-manifest.html).
*/
public var manifestConfig: aws.sdk.kotlin.services.datasync.model.ManifestConfig? = null
/**
* The name of your task.
*/
public var name: kotlin.String? = null
/**
* The task's settings. For example, what file metadata gets preserved, how data integrity gets verified at the end of your transfer, bandwidth limits, among other options.
*/
public var options: aws.sdk.kotlin.services.datasync.model.Options? = null
/**
* The schedule for when you want your task to run. For more information, see [Scheduling your task](https://docs.aws.amazon.com/datasync/latest/userguide/task-scheduling.html).
*/
public var schedule: aws.sdk.kotlin.services.datasync.model.TaskSchedule? = null
/**
* The details about your [task schedule](https://docs.aws.amazon.com/datasync/latest/userguide/task-scheduling.html).
*/
public var scheduleDetails: aws.sdk.kotlin.services.datasync.model.TaskScheduleDetails? = null
/**
* The ARN of your transfer's source location.
*/
public var sourceLocationArn: kotlin.String? = null
/**
* The ARNs of the [network interfaces](https://docs.aws.amazon.com/datasync/latest/userguide/datasync-network.html#required-network-interfaces) that DataSync created for your source location.
*/
public var sourceNetworkInterfaceArns: List? = null
/**
* The status of your task. For information about what each status means, see [Task statuses](https://docs.aws.amazon.com/datasync/latest/userguide/understand-task-statuses.html#understand-task-creation-statuses).
*/
public var status: aws.sdk.kotlin.services.datasync.model.TaskStatus? = null
/**
* The ARN of your task.
*/
public var taskArn: kotlin.String? = null
/**
* The configuration of your task report, which provides detailed information about your DataSync transfer. For more information, see [Monitoring your DataSync transfers with task reports](https://docs.aws.amazon.com/datasync/latest/userguide/task-reports.html).
*/
public var taskReportConfig: aws.sdk.kotlin.services.datasync.model.TaskReportConfig? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.DescribeTaskResponse) : this() {
this.cloudWatchLogGroupArn = x.cloudWatchLogGroupArn
this.creationTime = x.creationTime
this.currentTaskExecutionArn = x.currentTaskExecutionArn
this.destinationLocationArn = x.destinationLocationArn
this.destinationNetworkInterfaceArns = x.destinationNetworkInterfaceArns
this.errorCode = x.errorCode
this.errorDetail = x.errorDetail
this.excludes = x.excludes
this.includes = x.includes
this.manifestConfig = x.manifestConfig
this.name = x.name
this.options = x.options
this.schedule = x.schedule
this.scheduleDetails = x.scheduleDetails
this.sourceLocationArn = x.sourceLocationArn
this.sourceNetworkInterfaceArns = x.sourceNetworkInterfaceArns
this.status = x.status
this.taskArn = x.taskArn
this.taskReportConfig = x.taskReportConfig
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.DescribeTaskResponse = DescribeTaskResponse(this)
/**
* construct an [aws.sdk.kotlin.services.datasync.model.ManifestConfig] inside the given [block]
*/
public fun manifestConfig(block: aws.sdk.kotlin.services.datasync.model.ManifestConfig.Builder.() -> kotlin.Unit) {
this.manifestConfig = aws.sdk.kotlin.services.datasync.model.ManifestConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.datasync.model.Options] inside the given [block]
*/
public fun options(block: aws.sdk.kotlin.services.datasync.model.Options.Builder.() -> kotlin.Unit) {
this.options = aws.sdk.kotlin.services.datasync.model.Options.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.datasync.model.TaskSchedule] inside the given [block]
*/
public fun schedule(block: aws.sdk.kotlin.services.datasync.model.TaskSchedule.Builder.() -> kotlin.Unit) {
this.schedule = aws.sdk.kotlin.services.datasync.model.TaskSchedule.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.datasync.model.TaskScheduleDetails] inside the given [block]
*/
public fun scheduleDetails(block: aws.sdk.kotlin.services.datasync.model.TaskScheduleDetails.Builder.() -> kotlin.Unit) {
this.scheduleDetails = aws.sdk.kotlin.services.datasync.model.TaskScheduleDetails.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.datasync.model.TaskReportConfig] inside the given [block]
*/
public fun taskReportConfig(block: aws.sdk.kotlin.services.datasync.model.TaskReportConfig.Builder.() -> kotlin.Unit) {
this.taskReportConfig = aws.sdk.kotlin.services.datasync.model.TaskReportConfig.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy