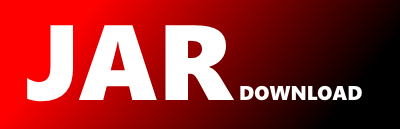
commonMain.aws.sdk.kotlin.services.datasync.model.MaxP95Performance.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datasync-jvm Show documentation
Show all versions of datasync-jvm Show documentation
The AWS SDK for Kotlin client for DataSync
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* The performance data that DataSync Discovery collects about an on-premises storage system resource.
*/
public class MaxP95Performance private constructor(builder: Builder) {
/**
* Peak IOPS unrelated to read and write operations.
*/
public val iopsOther: kotlin.Double? = builder.iopsOther
/**
* Peak IOPS related to read operations.
*/
public val iopsRead: kotlin.Double? = builder.iopsRead
/**
* Peak total IOPS on your on-premises storage system resource.
*/
public val iopsTotal: kotlin.Double? = builder.iopsTotal
/**
* Peak IOPS related to write operations.
*/
public val iopsWrite: kotlin.Double? = builder.iopsWrite
/**
* Peak latency for operations unrelated to read and write operations.
*/
public val latencyOther: kotlin.Double? = builder.latencyOther
/**
* Peak latency for read operations.
*/
public val latencyRead: kotlin.Double? = builder.latencyRead
/**
* Peak latency for write operations.
*/
public val latencyWrite: kotlin.Double? = builder.latencyWrite
/**
* Peak throughput unrelated to read and write operations.
*/
public val throughputOther: kotlin.Double? = builder.throughputOther
/**
* Peak throughput related to read operations.
*/
public val throughputRead: kotlin.Double? = builder.throughputRead
/**
* Peak total throughput on your on-premises storage system resource.
*/
public val throughputTotal: kotlin.Double? = builder.throughputTotal
/**
* Peak throughput related to write operations.
*/
public val throughputWrite: kotlin.Double? = builder.throughputWrite
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.MaxP95Performance = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("MaxP95Performance(")
append("iopsOther=$iopsOther,")
append("iopsRead=$iopsRead,")
append("iopsTotal=$iopsTotal,")
append("iopsWrite=$iopsWrite,")
append("latencyOther=$latencyOther,")
append("latencyRead=$latencyRead,")
append("latencyWrite=$latencyWrite,")
append("throughputOther=$throughputOther,")
append("throughputRead=$throughputRead,")
append("throughputTotal=$throughputTotal,")
append("throughputWrite=$throughputWrite")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = iopsOther?.hashCode() ?: 0
result = 31 * result + (iopsRead?.hashCode() ?: 0)
result = 31 * result + (iopsTotal?.hashCode() ?: 0)
result = 31 * result + (iopsWrite?.hashCode() ?: 0)
result = 31 * result + (latencyOther?.hashCode() ?: 0)
result = 31 * result + (latencyRead?.hashCode() ?: 0)
result = 31 * result + (latencyWrite?.hashCode() ?: 0)
result = 31 * result + (throughputOther?.hashCode() ?: 0)
result = 31 * result + (throughputRead?.hashCode() ?: 0)
result = 31 * result + (throughputTotal?.hashCode() ?: 0)
result = 31 * result + (throughputWrite?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as MaxP95Performance
if (!(iopsOther?.equals(other.iopsOther) ?: (other.iopsOther == null))) return false
if (!(iopsRead?.equals(other.iopsRead) ?: (other.iopsRead == null))) return false
if (!(iopsTotal?.equals(other.iopsTotal) ?: (other.iopsTotal == null))) return false
if (!(iopsWrite?.equals(other.iopsWrite) ?: (other.iopsWrite == null))) return false
if (!(latencyOther?.equals(other.latencyOther) ?: (other.latencyOther == null))) return false
if (!(latencyRead?.equals(other.latencyRead) ?: (other.latencyRead == null))) return false
if (!(latencyWrite?.equals(other.latencyWrite) ?: (other.latencyWrite == null))) return false
if (!(throughputOther?.equals(other.throughputOther) ?: (other.throughputOther == null))) return false
if (!(throughputRead?.equals(other.throughputRead) ?: (other.throughputRead == null))) return false
if (!(throughputTotal?.equals(other.throughputTotal) ?: (other.throughputTotal == null))) return false
if (!(throughputWrite?.equals(other.throughputWrite) ?: (other.throughputWrite == null))) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.MaxP95Performance = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Peak IOPS unrelated to read and write operations.
*/
public var iopsOther: kotlin.Double? = null
/**
* Peak IOPS related to read operations.
*/
public var iopsRead: kotlin.Double? = null
/**
* Peak total IOPS on your on-premises storage system resource.
*/
public var iopsTotal: kotlin.Double? = null
/**
* Peak IOPS related to write operations.
*/
public var iopsWrite: kotlin.Double? = null
/**
* Peak latency for operations unrelated to read and write operations.
*/
public var latencyOther: kotlin.Double? = null
/**
* Peak latency for read operations.
*/
public var latencyRead: kotlin.Double? = null
/**
* Peak latency for write operations.
*/
public var latencyWrite: kotlin.Double? = null
/**
* Peak throughput unrelated to read and write operations.
*/
public var throughputOther: kotlin.Double? = null
/**
* Peak throughput related to read operations.
*/
public var throughputRead: kotlin.Double? = null
/**
* Peak total throughput on your on-premises storage system resource.
*/
public var throughputTotal: kotlin.Double? = null
/**
* Peak throughput related to write operations.
*/
public var throughputWrite: kotlin.Double? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.MaxP95Performance) : this() {
this.iopsOther = x.iopsOther
this.iopsRead = x.iopsRead
this.iopsTotal = x.iopsTotal
this.iopsWrite = x.iopsWrite
this.latencyOther = x.latencyOther
this.latencyRead = x.latencyRead
this.latencyWrite = x.latencyWrite
this.throughputOther = x.throughputOther
this.throughputRead = x.throughputRead
this.throughputTotal = x.throughputTotal
this.throughputWrite = x.throughputWrite
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.MaxP95Performance = MaxP95Performance(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy