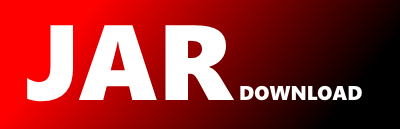
commonMain.aws.sdk.kotlin.services.datasync.model.NetAppOntapVolume.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datasync-jvm Show documentation
Show all versions of datasync-jvm Show documentation
The AWS SDK for Kotlin client for DataSync
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* The information that DataSync Discovery collects about a volume in your on-premises storage system.
*/
public class NetAppOntapVolume private constructor(builder: Builder) {
/**
* The total storage space that's available in the volume.
*/
public val capacityProvisioned: kotlin.Long? = builder.capacityProvisioned
/**
* The storage space that's being used in the volume.
*/
public val capacityUsed: kotlin.Long? = builder.capacityUsed
/**
* The number of CIFS shares in the volume.
*/
public val cifsShareCount: kotlin.Long? = builder.cifsShareCount
/**
* The storage space that's being used in the volume without accounting for compression or deduplication.
*/
public val logicalCapacityUsed: kotlin.Long? = builder.logicalCapacityUsed
/**
* The number of LUNs (logical unit numbers) in the volume.
*/
public val lunCount: kotlin.Long? = builder.lunCount
/**
* The performance data that DataSync Discovery collects about the volume.
*/
public val maxP95Performance: aws.sdk.kotlin.services.datasync.model.MaxP95Performance? = builder.maxP95Performance
/**
* The number of NFS volumes in the volume.
*/
public val nfsExported: kotlin.Boolean = builder.nfsExported
/**
* Indicates whether DataSync Discovery recommendations for the volume are ready to view, incomplete, or can't be determined.
*
* For more information, see [Recommendation statuses](https://docs.aws.amazon.com/datasync/latest/userguide/discovery-job-statuses.html#recommendation-statuses-table).
*/
public val recommendationStatus: aws.sdk.kotlin.services.datasync.model.RecommendationStatus? = builder.recommendationStatus
/**
* The Amazon Web Services storage services that DataSync Discovery recommends for the volume. For more information, see [Recommendations provided by DataSync Discovery](https://docs.aws.amazon.com/datasync/latest/userguide/discovery-understand-recommendations.html).
*/
public val recommendations: List? = builder.recommendations
/**
* The universally unique identifier (UUID) of the volume.
*/
public val resourceId: kotlin.String? = builder.resourceId
/**
* The volume's security style (such as Unix or NTFS).
*/
public val securityStyle: kotlin.String? = builder.securityStyle
/**
* The amount of storage in the volume that's being used for snapshots.
*/
public val snapshotCapacityUsed: kotlin.Long? = builder.snapshotCapacityUsed
/**
* The name of the SVM associated with the volume.
*/
public val svmName: kotlin.String? = builder.svmName
/**
* The UUID of the storage virtual machine (SVM) associated with the volume.
*/
public val svmUuid: kotlin.String? = builder.svmUuid
/**
* The name of the volume.
*/
public val volumeName: kotlin.String? = builder.volumeName
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.NetAppOntapVolume = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("NetAppOntapVolume(")
append("capacityProvisioned=$capacityProvisioned,")
append("capacityUsed=$capacityUsed,")
append("cifsShareCount=$cifsShareCount,")
append("logicalCapacityUsed=$logicalCapacityUsed,")
append("lunCount=$lunCount,")
append("maxP95Performance=$maxP95Performance,")
append("nfsExported=$nfsExported,")
append("recommendationStatus=$recommendationStatus,")
append("recommendations=$recommendations,")
append("resourceId=$resourceId,")
append("securityStyle=$securityStyle,")
append("snapshotCapacityUsed=$snapshotCapacityUsed,")
append("svmName=$svmName,")
append("svmUuid=$svmUuid,")
append("volumeName=$volumeName")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = capacityProvisioned?.hashCode() ?: 0
result = 31 * result + (capacityUsed?.hashCode() ?: 0)
result = 31 * result + (cifsShareCount?.hashCode() ?: 0)
result = 31 * result + (logicalCapacityUsed?.hashCode() ?: 0)
result = 31 * result + (lunCount?.hashCode() ?: 0)
result = 31 * result + (maxP95Performance?.hashCode() ?: 0)
result = 31 * result + (nfsExported.hashCode())
result = 31 * result + (recommendationStatus?.hashCode() ?: 0)
result = 31 * result + (recommendations?.hashCode() ?: 0)
result = 31 * result + (resourceId?.hashCode() ?: 0)
result = 31 * result + (securityStyle?.hashCode() ?: 0)
result = 31 * result + (snapshotCapacityUsed?.hashCode() ?: 0)
result = 31 * result + (svmName?.hashCode() ?: 0)
result = 31 * result + (svmUuid?.hashCode() ?: 0)
result = 31 * result + (volumeName?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as NetAppOntapVolume
if (capacityProvisioned != other.capacityProvisioned) return false
if (capacityUsed != other.capacityUsed) return false
if (cifsShareCount != other.cifsShareCount) return false
if (logicalCapacityUsed != other.logicalCapacityUsed) return false
if (lunCount != other.lunCount) return false
if (maxP95Performance != other.maxP95Performance) return false
if (nfsExported != other.nfsExported) return false
if (recommendationStatus != other.recommendationStatus) return false
if (recommendations != other.recommendations) return false
if (resourceId != other.resourceId) return false
if (securityStyle != other.securityStyle) return false
if (snapshotCapacityUsed != other.snapshotCapacityUsed) return false
if (svmName != other.svmName) return false
if (svmUuid != other.svmUuid) return false
if (volumeName != other.volumeName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.NetAppOntapVolume = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The total storage space that's available in the volume.
*/
public var capacityProvisioned: kotlin.Long? = null
/**
* The storage space that's being used in the volume.
*/
public var capacityUsed: kotlin.Long? = null
/**
* The number of CIFS shares in the volume.
*/
public var cifsShareCount: kotlin.Long? = null
/**
* The storage space that's being used in the volume without accounting for compression or deduplication.
*/
public var logicalCapacityUsed: kotlin.Long? = null
/**
* The number of LUNs (logical unit numbers) in the volume.
*/
public var lunCount: kotlin.Long? = null
/**
* The performance data that DataSync Discovery collects about the volume.
*/
public var maxP95Performance: aws.sdk.kotlin.services.datasync.model.MaxP95Performance? = null
/**
* The number of NFS volumes in the volume.
*/
public var nfsExported: kotlin.Boolean = false
/**
* Indicates whether DataSync Discovery recommendations for the volume are ready to view, incomplete, or can't be determined.
*
* For more information, see [Recommendation statuses](https://docs.aws.amazon.com/datasync/latest/userguide/discovery-job-statuses.html#recommendation-statuses-table).
*/
public var recommendationStatus: aws.sdk.kotlin.services.datasync.model.RecommendationStatus? = null
/**
* The Amazon Web Services storage services that DataSync Discovery recommends for the volume. For more information, see [Recommendations provided by DataSync Discovery](https://docs.aws.amazon.com/datasync/latest/userguide/discovery-understand-recommendations.html).
*/
public var recommendations: List? = null
/**
* The universally unique identifier (UUID) of the volume.
*/
public var resourceId: kotlin.String? = null
/**
* The volume's security style (such as Unix or NTFS).
*/
public var securityStyle: kotlin.String? = null
/**
* The amount of storage in the volume that's being used for snapshots.
*/
public var snapshotCapacityUsed: kotlin.Long? = null
/**
* The name of the SVM associated with the volume.
*/
public var svmName: kotlin.String? = null
/**
* The UUID of the storage virtual machine (SVM) associated with the volume.
*/
public var svmUuid: kotlin.String? = null
/**
* The name of the volume.
*/
public var volumeName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.NetAppOntapVolume) : this() {
this.capacityProvisioned = x.capacityProvisioned
this.capacityUsed = x.capacityUsed
this.cifsShareCount = x.cifsShareCount
this.logicalCapacityUsed = x.logicalCapacityUsed
this.lunCount = x.lunCount
this.maxP95Performance = x.maxP95Performance
this.nfsExported = x.nfsExported
this.recommendationStatus = x.recommendationStatus
this.recommendations = x.recommendations
this.resourceId = x.resourceId
this.securityStyle = x.securityStyle
this.snapshotCapacityUsed = x.snapshotCapacityUsed
this.svmName = x.svmName
this.svmUuid = x.svmUuid
this.volumeName = x.volumeName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.NetAppOntapVolume = NetAppOntapVolume(this)
/**
* construct an [aws.sdk.kotlin.services.datasync.model.MaxP95Performance] inside the given [block]
*/
public fun maxP95Performance(block: aws.sdk.kotlin.services.datasync.model.MaxP95Performance.Builder.() -> kotlin.Unit) {
this.maxP95Performance = aws.sdk.kotlin.services.datasync.model.MaxP95Performance.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy