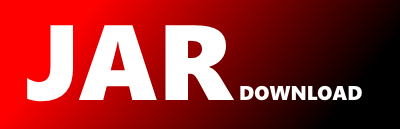
commonMain.aws.sdk.kotlin.services.datasync.model.ResourceMetrics.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datasync-jvm Show documentation
Show all versions of datasync-jvm Show documentation
The AWS SDK for Kotlin client for DataSync
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Information, including performance data and capacity usage, provided by DataSync Discovery about a resource in your on-premises storage system.
*/
public class ResourceMetrics private constructor(builder: Builder) {
/**
* The storage capacity of the on-premises storage system resource.
*/
public val capacity: aws.sdk.kotlin.services.datasync.model.Capacity? = builder.capacity
/**
* The types of performance data that DataSync Discovery collects about the on-premises storage system resource.
*/
public val p95Metrics: aws.sdk.kotlin.services.datasync.model.P95Metrics? = builder.p95Metrics
/**
* The universally unique identifier (UUID) of the on-premises storage system resource.
*/
public val resourceId: kotlin.String? = builder.resourceId
/**
* The type of on-premises storage system resource.
*/
public val resourceType: aws.sdk.kotlin.services.datasync.model.DiscoveryResourceType? = builder.resourceType
/**
* The time when DataSync Discovery collected this information from the resource.
*/
public val timestamp: aws.smithy.kotlin.runtime.time.Instant? = builder.timestamp
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.ResourceMetrics = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ResourceMetrics(")
append("capacity=$capacity,")
append("p95Metrics=$p95Metrics,")
append("resourceId=$resourceId,")
append("resourceType=$resourceType,")
append("timestamp=$timestamp")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = capacity?.hashCode() ?: 0
result = 31 * result + (p95Metrics?.hashCode() ?: 0)
result = 31 * result + (resourceId?.hashCode() ?: 0)
result = 31 * result + (resourceType?.hashCode() ?: 0)
result = 31 * result + (timestamp?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ResourceMetrics
if (capacity != other.capacity) return false
if (p95Metrics != other.p95Metrics) return false
if (resourceId != other.resourceId) return false
if (resourceType != other.resourceType) return false
if (timestamp != other.timestamp) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.ResourceMetrics = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The storage capacity of the on-premises storage system resource.
*/
public var capacity: aws.sdk.kotlin.services.datasync.model.Capacity? = null
/**
* The types of performance data that DataSync Discovery collects about the on-premises storage system resource.
*/
public var p95Metrics: aws.sdk.kotlin.services.datasync.model.P95Metrics? = null
/**
* The universally unique identifier (UUID) of the on-premises storage system resource.
*/
public var resourceId: kotlin.String? = null
/**
* The type of on-premises storage system resource.
*/
public var resourceType: aws.sdk.kotlin.services.datasync.model.DiscoveryResourceType? = null
/**
* The time when DataSync Discovery collected this information from the resource.
*/
public var timestamp: aws.smithy.kotlin.runtime.time.Instant? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.ResourceMetrics) : this() {
this.capacity = x.capacity
this.p95Metrics = x.p95Metrics
this.resourceId = x.resourceId
this.resourceType = x.resourceType
this.timestamp = x.timestamp
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.ResourceMetrics = ResourceMetrics(this)
/**
* construct an [aws.sdk.kotlin.services.datasync.model.Capacity] inside the given [block]
*/
public fun capacity(block: aws.sdk.kotlin.services.datasync.model.Capacity.Builder.() -> kotlin.Unit) {
this.capacity = aws.sdk.kotlin.services.datasync.model.Capacity.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.datasync.model.P95Metrics] inside the given [block]
*/
public fun p95Metrics(block: aws.sdk.kotlin.services.datasync.model.P95Metrics.Builder.() -> kotlin.Unit) {
this.p95Metrics = aws.sdk.kotlin.services.datasync.model.P95Metrics.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy