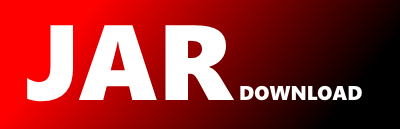
commonMain.aws.sdk.kotlin.services.datasync.model.TaskScheduleDetails.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datasync-jvm Show documentation
Show all versions of datasync-jvm Show documentation
The AWS SDK for Kotlin client for DataSync
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Provides information about your DataSync [task schedule](https://docs.aws.amazon.com/datasync/latest/userguide/task-scheduling.html).
*/
public class TaskScheduleDetails private constructor(builder: Builder) {
/**
* Indicates how your task schedule was disabled.
* + `USER` - Your schedule was manually disabled by using the [UpdateTask](https://docs.aws.amazon.com/datasync/latest/userguide/API_UpdateTask.html) operation or DataSync console.
* + `SERVICE` - Your schedule was automatically disabled by DataSync because the task failed repeatedly with the same error.
*/
public val disabledBy: aws.sdk.kotlin.services.datasync.model.ScheduleDisabledBy? = builder.disabledBy
/**
* Provides a reason if the task schedule is disabled.
*
* If your schedule is disabled by `USER`, you see a `Manually disabled by user.` message.
*
* If your schedule is disabled by `SERVICE`, you see an error message to help you understand why the task keeps failing. For information on resolving DataSync errors, see [Troubleshooting issues with DataSync transfers](https://docs.aws.amazon.com/datasync/latest/userguide/troubleshooting-datasync-locations-tasks.html).
*/
public val disabledReason: kotlin.String? = builder.disabledReason
/**
* Indicates the last time the status of your task schedule changed. For example, if DataSync automatically disables your schedule because of a repeated error, you can see when the schedule was disabled.
*/
public val statusUpdateTime: aws.smithy.kotlin.runtime.time.Instant? = builder.statusUpdateTime
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.TaskScheduleDetails = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("TaskScheduleDetails(")
append("disabledBy=$disabledBy,")
append("disabledReason=$disabledReason,")
append("statusUpdateTime=$statusUpdateTime")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = disabledBy?.hashCode() ?: 0
result = 31 * result + (disabledReason?.hashCode() ?: 0)
result = 31 * result + (statusUpdateTime?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as TaskScheduleDetails
if (disabledBy != other.disabledBy) return false
if (disabledReason != other.disabledReason) return false
if (statusUpdateTime != other.statusUpdateTime) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.TaskScheduleDetails = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Indicates how your task schedule was disabled.
* + `USER` - Your schedule was manually disabled by using the [UpdateTask](https://docs.aws.amazon.com/datasync/latest/userguide/API_UpdateTask.html) operation or DataSync console.
* + `SERVICE` - Your schedule was automatically disabled by DataSync because the task failed repeatedly with the same error.
*/
public var disabledBy: aws.sdk.kotlin.services.datasync.model.ScheduleDisabledBy? = null
/**
* Provides a reason if the task schedule is disabled.
*
* If your schedule is disabled by `USER`, you see a `Manually disabled by user.` message.
*
* If your schedule is disabled by `SERVICE`, you see an error message to help you understand why the task keeps failing. For information on resolving DataSync errors, see [Troubleshooting issues with DataSync transfers](https://docs.aws.amazon.com/datasync/latest/userguide/troubleshooting-datasync-locations-tasks.html).
*/
public var disabledReason: kotlin.String? = null
/**
* Indicates the last time the status of your task schedule changed. For example, if DataSync automatically disables your schedule because of a repeated error, you can see when the schedule was disabled.
*/
public var statusUpdateTime: aws.smithy.kotlin.runtime.time.Instant? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.TaskScheduleDetails) : this() {
this.disabledBy = x.disabledBy
this.disabledReason = x.disabledReason
this.statusUpdateTime = x.statusUpdateTime
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.TaskScheduleDetails = TaskScheduleDetails(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy