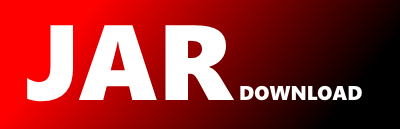
commonMain.aws.sdk.kotlin.services.datasync.model.UpdateLocationObjectStorageRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datasync-jvm Show documentation
Show all versions of datasync-jvm Show documentation
The AWS SDK for Kotlin client for DataSync
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
public class UpdateLocationObjectStorageRequest private constructor(builder: Builder) {
/**
* Specifies the access key (for example, a user name) if credentials are required to authenticate with the object storage server.
*/
public val accessKey: kotlin.String? = builder.accessKey
/**
* Specifies the Amazon Resource Names (ARNs) of the DataSync agents that can securely connect with your location.
*/
public val agentArns: List? = builder.agentArns
/**
* Specifies the ARN of the object storage system location that you're updating.
*/
public val locationArn: kotlin.String? = builder.locationArn
/**
* Specifies the secret key (for example, a password) if credentials are required to authenticate with the object storage server.
*/
public val secretKey: kotlin.String? = builder.secretKey
/**
* Specifies a certificate chain for DataSync to authenticate with your object storage system if the system uses a private or self-signed certificate authority (CA). You must specify a single `.pem` file with a full certificate chain (for example, `file:///home/user/.ssh/object_storage_certificates.pem`).
*
* The certificate chain might include:
* + The object storage system's certificate
* + All intermediate certificates (if there are any)
* + The root certificate of the signing CA
*
* You can concatenate your certificates into a `.pem` file (which can be up to 32768 bytes before base64 encoding). The following example `cat` command creates an `object_storage_certificates.pem` file that includes three certificates:
*
* `cat object_server_certificate.pem intermediate_certificate.pem ca_root_certificate.pem > object_storage_certificates.pem`
*
* To use this parameter, configure `ServerProtocol` to `HTTPS`.
*
* Updating this parameter doesn't interfere with tasks that you have in progress.
*/
public val serverCertificate: kotlin.ByteArray? = builder.serverCertificate
/**
* Specifies the port that your object storage server accepts inbound network traffic on (for example, port 443).
*/
public val serverPort: kotlin.Int? = builder.serverPort
/**
* Specifies the protocol that your object storage server uses to communicate.
*/
public val serverProtocol: aws.sdk.kotlin.services.datasync.model.ObjectStorageServerProtocol? = builder.serverProtocol
/**
* Specifies the object prefix for your object storage server. If this is a source location, DataSync only copies objects with this prefix. If this is a destination location, DataSync writes all objects with this prefix.
*/
public val subdirectory: kotlin.String? = builder.subdirectory
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.UpdateLocationObjectStorageRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateLocationObjectStorageRequest(")
append("accessKey=$accessKey,")
append("agentArns=$agentArns,")
append("locationArn=$locationArn,")
append("secretKey=*** Sensitive Data Redacted ***,")
append("serverCertificate=$serverCertificate,")
append("serverPort=$serverPort,")
append("serverProtocol=$serverProtocol,")
append("subdirectory=$subdirectory")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = accessKey?.hashCode() ?: 0
result = 31 * result + (agentArns?.hashCode() ?: 0)
result = 31 * result + (locationArn?.hashCode() ?: 0)
result = 31 * result + (secretKey?.hashCode() ?: 0)
result = 31 * result + (serverCertificate?.contentHashCode() ?: 0)
result = 31 * result + (serverPort ?: 0)
result = 31 * result + (serverProtocol?.hashCode() ?: 0)
result = 31 * result + (subdirectory?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdateLocationObjectStorageRequest
if (accessKey != other.accessKey) return false
if (agentArns != other.agentArns) return false
if (locationArn != other.locationArn) return false
if (secretKey != other.secretKey) return false
if (serverCertificate != null) {
if (other.serverCertificate == null) return false
if (!serverCertificate.contentEquals(other.serverCertificate)) return false
} else if (other.serverCertificate != null) return false
if (serverPort != other.serverPort) return false
if (serverProtocol != other.serverProtocol) return false
if (subdirectory != other.subdirectory) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.UpdateLocationObjectStorageRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Specifies the access key (for example, a user name) if credentials are required to authenticate with the object storage server.
*/
public var accessKey: kotlin.String? = null
/**
* Specifies the Amazon Resource Names (ARNs) of the DataSync agents that can securely connect with your location.
*/
public var agentArns: List? = null
/**
* Specifies the ARN of the object storage system location that you're updating.
*/
public var locationArn: kotlin.String? = null
/**
* Specifies the secret key (for example, a password) if credentials are required to authenticate with the object storage server.
*/
public var secretKey: kotlin.String? = null
/**
* Specifies a certificate chain for DataSync to authenticate with your object storage system if the system uses a private or self-signed certificate authority (CA). You must specify a single `.pem` file with a full certificate chain (for example, `file:///home/user/.ssh/object_storage_certificates.pem`).
*
* The certificate chain might include:
* + The object storage system's certificate
* + All intermediate certificates (if there are any)
* + The root certificate of the signing CA
*
* You can concatenate your certificates into a `.pem` file (which can be up to 32768 bytes before base64 encoding). The following example `cat` command creates an `object_storage_certificates.pem` file that includes three certificates:
*
* `cat object_server_certificate.pem intermediate_certificate.pem ca_root_certificate.pem > object_storage_certificates.pem`
*
* To use this parameter, configure `ServerProtocol` to `HTTPS`.
*
* Updating this parameter doesn't interfere with tasks that you have in progress.
*/
public var serverCertificate: kotlin.ByteArray? = null
/**
* Specifies the port that your object storage server accepts inbound network traffic on (for example, port 443).
*/
public var serverPort: kotlin.Int? = null
/**
* Specifies the protocol that your object storage server uses to communicate.
*/
public var serverProtocol: aws.sdk.kotlin.services.datasync.model.ObjectStorageServerProtocol? = null
/**
* Specifies the object prefix for your object storage server. If this is a source location, DataSync only copies objects with this prefix. If this is a destination location, DataSync writes all objects with this prefix.
*/
public var subdirectory: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.UpdateLocationObjectStorageRequest) : this() {
this.accessKey = x.accessKey
this.agentArns = x.agentArns
this.locationArn = x.locationArn
this.secretKey = x.secretKey
this.serverCertificate = x.serverCertificate
this.serverPort = x.serverPort
this.serverProtocol = x.serverProtocol
this.subdirectory = x.subdirectory
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.UpdateLocationObjectStorageRequest = UpdateLocationObjectStorageRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy