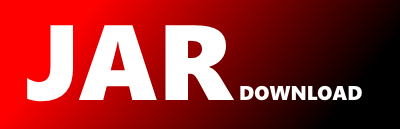
commonMain.aws.sdk.kotlin.services.datasync.model.AddStorageSystemRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
public class AddStorageSystemRequest private constructor(builder: Builder) {
/**
* Specifies the Amazon Resource Name (ARN) of the DataSync agent that connects to and reads from your on-premises storage system's management interface. You can only specify one ARN.
*/
public val agentArns: List? = builder.agentArns
/**
* Specifies a client token to make sure requests with this API operation are idempotent. If you don't specify a client token, DataSync generates one for you automatically.
*/
public val clientToken: kotlin.String? = builder.clientToken
/**
* Specifies the ARN of the Amazon CloudWatch log group for monitoring and logging discovery job events.
*/
public val cloudWatchLogGroupArn: kotlin.String? = builder.cloudWatchLogGroupArn
/**
* Specifies the user name and password for accessing your on-premises storage system's management interface.
*/
public val credentials: aws.sdk.kotlin.services.datasync.model.Credentials? = builder.credentials
/**
* Specifies a familiar name for your on-premises storage system.
*/
public val name: kotlin.String? = builder.name
/**
* Specifies the server name and network port required to connect with the management interface of your on-premises storage system.
*/
public val serverConfiguration: aws.sdk.kotlin.services.datasync.model.DiscoveryServerConfiguration? = builder.serverConfiguration
/**
* Specifies the type of on-premises storage system that you want DataSync Discovery to collect information about.
*
* DataSync Discovery currently supports NetApp Fabric-Attached Storage (FAS) and All Flash FAS (AFF) systems running ONTAP 9.7 or later.
*/
public val systemType: aws.sdk.kotlin.services.datasync.model.DiscoverySystemType? = builder.systemType
/**
* Specifies labels that help you categorize, filter, and search for your Amazon Web Services resources. We recommend creating at least a name tag for your on-premises storage system.
*/
public val tags: List? = builder.tags
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.AddStorageSystemRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("AddStorageSystemRequest(")
append("agentArns=$agentArns,")
append("clientToken=$clientToken,")
append("cloudWatchLogGroupArn=$cloudWatchLogGroupArn,")
append("credentials=$credentials,")
append("name=$name,")
append("serverConfiguration=$serverConfiguration,")
append("systemType=$systemType,")
append("tags=$tags")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = agentArns?.hashCode() ?: 0
result = 31 * result + (clientToken?.hashCode() ?: 0)
result = 31 * result + (cloudWatchLogGroupArn?.hashCode() ?: 0)
result = 31 * result + (credentials?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (serverConfiguration?.hashCode() ?: 0)
result = 31 * result + (systemType?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AddStorageSystemRequest
if (agentArns != other.agentArns) return false
if (clientToken != other.clientToken) return false
if (cloudWatchLogGroupArn != other.cloudWatchLogGroupArn) return false
if (credentials != other.credentials) return false
if (name != other.name) return false
if (serverConfiguration != other.serverConfiguration) return false
if (systemType != other.systemType) return false
if (tags != other.tags) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.AddStorageSystemRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Specifies the Amazon Resource Name (ARN) of the DataSync agent that connects to and reads from your on-premises storage system's management interface. You can only specify one ARN.
*/
public var agentArns: List? = null
/**
* Specifies a client token to make sure requests with this API operation are idempotent. If you don't specify a client token, DataSync generates one for you automatically.
*/
public var clientToken: kotlin.String? = null
/**
* Specifies the ARN of the Amazon CloudWatch log group for monitoring and logging discovery job events.
*/
public var cloudWatchLogGroupArn: kotlin.String? = null
/**
* Specifies the user name and password for accessing your on-premises storage system's management interface.
*/
public var credentials: aws.sdk.kotlin.services.datasync.model.Credentials? = null
/**
* Specifies a familiar name for your on-premises storage system.
*/
public var name: kotlin.String? = null
/**
* Specifies the server name and network port required to connect with the management interface of your on-premises storage system.
*/
public var serverConfiguration: aws.sdk.kotlin.services.datasync.model.DiscoveryServerConfiguration? = null
/**
* Specifies the type of on-premises storage system that you want DataSync Discovery to collect information about.
*
* DataSync Discovery currently supports NetApp Fabric-Attached Storage (FAS) and All Flash FAS (AFF) systems running ONTAP 9.7 or later.
*/
public var systemType: aws.sdk.kotlin.services.datasync.model.DiscoverySystemType? = null
/**
* Specifies labels that help you categorize, filter, and search for your Amazon Web Services resources. We recommend creating at least a name tag for your on-premises storage system.
*/
public var tags: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.AddStorageSystemRequest) : this() {
this.agentArns = x.agentArns
this.clientToken = x.clientToken
this.cloudWatchLogGroupArn = x.cloudWatchLogGroupArn
this.credentials = x.credentials
this.name = x.name
this.serverConfiguration = x.serverConfiguration
this.systemType = x.systemType
this.tags = x.tags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.AddStorageSystemRequest = AddStorageSystemRequest(this)
/**
* construct an [aws.sdk.kotlin.services.datasync.model.Credentials] inside the given [block]
*/
public fun credentials(block: aws.sdk.kotlin.services.datasync.model.Credentials.Builder.() -> kotlin.Unit) {
this.credentials = aws.sdk.kotlin.services.datasync.model.Credentials.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.datasync.model.DiscoveryServerConfiguration] inside the given [block]
*/
public fun serverConfiguration(block: aws.sdk.kotlin.services.datasync.model.DiscoveryServerConfiguration.Builder.() -> kotlin.Unit) {
this.serverConfiguration = aws.sdk.kotlin.services.datasync.model.DiscoveryServerConfiguration.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy