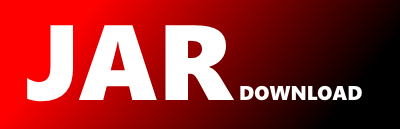
commonMain.aws.sdk.kotlin.services.datasync.model.CreateLocationFsxOpenZfsRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
public class CreateLocationFsxOpenZfsRequest private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) of the FSx for OpenZFS file system.
*/
public val fsxFilesystemArn: kotlin.String? = builder.fsxFilesystemArn
/**
* The type of protocol that DataSync uses to access your file system.
*/
public val protocol: aws.sdk.kotlin.services.datasync.model.FsxProtocol? = builder.protocol
/**
* The ARNs of the security groups that are used to configure the FSx for OpenZFS file system.
*/
public val securityGroupArns: List? = builder.securityGroupArns
/**
* A subdirectory in the location's path that must begin with `/fsx`. DataSync uses this subdirectory to read or write data (depending on whether the file system is a source or destination location).
*/
public val subdirectory: kotlin.String? = builder.subdirectory
/**
* The key-value pair that represents a tag that you want to add to the resource. The value can be an empty string. This value helps you manage, filter, and search for your resources. We recommend that you create a name tag for your location.
*/
public val tags: List? = builder.tags
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.CreateLocationFsxOpenZfsRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateLocationFsxOpenZfsRequest(")
append("fsxFilesystemArn=$fsxFilesystemArn,")
append("protocol=$protocol,")
append("securityGroupArns=$securityGroupArns,")
append("subdirectory=$subdirectory,")
append("tags=$tags")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = fsxFilesystemArn?.hashCode() ?: 0
result = 31 * result + (protocol?.hashCode() ?: 0)
result = 31 * result + (securityGroupArns?.hashCode() ?: 0)
result = 31 * result + (subdirectory?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateLocationFsxOpenZfsRequest
if (fsxFilesystemArn != other.fsxFilesystemArn) return false
if (protocol != other.protocol) return false
if (securityGroupArns != other.securityGroupArns) return false
if (subdirectory != other.subdirectory) return false
if (tags != other.tags) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.CreateLocationFsxOpenZfsRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The Amazon Resource Name (ARN) of the FSx for OpenZFS file system.
*/
public var fsxFilesystemArn: kotlin.String? = null
/**
* The type of protocol that DataSync uses to access your file system.
*/
public var protocol: aws.sdk.kotlin.services.datasync.model.FsxProtocol? = null
/**
* The ARNs of the security groups that are used to configure the FSx for OpenZFS file system.
*/
public var securityGroupArns: List? = null
/**
* A subdirectory in the location's path that must begin with `/fsx`. DataSync uses this subdirectory to read or write data (depending on whether the file system is a source or destination location).
*/
public var subdirectory: kotlin.String? = null
/**
* The key-value pair that represents a tag that you want to add to the resource. The value can be an empty string. This value helps you manage, filter, and search for your resources. We recommend that you create a name tag for your location.
*/
public var tags: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.CreateLocationFsxOpenZfsRequest) : this() {
this.fsxFilesystemArn = x.fsxFilesystemArn
this.protocol = x.protocol
this.securityGroupArns = x.securityGroupArns
this.subdirectory = x.subdirectory
this.tags = x.tags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.CreateLocationFsxOpenZfsRequest = CreateLocationFsxOpenZfsRequest(this)
/**
* construct an [aws.sdk.kotlin.services.datasync.model.FsxProtocol] inside the given [block]
*/
public fun protocol(block: aws.sdk.kotlin.services.datasync.model.FsxProtocol.Builder.() -> kotlin.Unit) {
this.protocol = aws.sdk.kotlin.services.datasync.model.FsxProtocol.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy