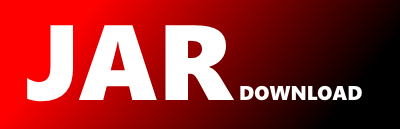
commonMain.aws.sdk.kotlin.services.datasync.model.DescribeAgentResponse.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* DescribeAgentResponse
*/
public class DescribeAgentResponse private constructor(builder: Builder) {
/**
* The ARN of the agent.
*/
public val agentArn: kotlin.String? = builder.agentArn
/**
* The time that the agent was [activated](https://docs.aws.amazon.com/datasync/latest/userguide/activate-agent.html).
*/
public val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* The type of [service endpoint](https://docs.aws.amazon.com/datasync/latest/userguide/choose-service-endpoint.html) that your agent is connected to.
*/
public val endpointType: aws.sdk.kotlin.services.datasync.model.EndpointType? = builder.endpointType
/**
* The last time that the agent was communicating with the DataSync service.
*/
public val lastConnectionTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastConnectionTime
/**
* The name of the agent.
*/
public val name: kotlin.String? = builder.name
/**
* The platform-related details about the agent, such as the version number.
*/
public val platform: aws.sdk.kotlin.services.datasync.model.Platform? = builder.platform
/**
* The network configuration that the agent uses when connecting to a [VPC service endpoint](https://docs.aws.amazon.com/datasync/latest/userguide/choose-service-endpoint.html#choose-service-endpoint-vpc).
*/
public val privateLinkConfig: aws.sdk.kotlin.services.datasync.model.PrivateLinkConfig? = builder.privateLinkConfig
/**
* The status of the agent.
* + If the status is `ONLINE`, the agent is configured properly and ready to use.
* + If the status is `OFFLINE`, the agent has been out of contact with DataSync for five minutes or longer. This can happen for a few reasons. For more information, see [What do I do if my agent is offline?](https://docs.aws.amazon.com/datasync/latest/userguide/troubleshooting-datasync-agents.html#troubleshoot-agent-offline)
*/
public val status: aws.sdk.kotlin.services.datasync.model.AgentStatus? = builder.status
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.DescribeAgentResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeAgentResponse(")
append("agentArn=$agentArn,")
append("creationTime=$creationTime,")
append("endpointType=$endpointType,")
append("lastConnectionTime=$lastConnectionTime,")
append("name=$name,")
append("platform=$platform,")
append("privateLinkConfig=$privateLinkConfig,")
append("status=$status")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = agentArn?.hashCode() ?: 0
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (endpointType?.hashCode() ?: 0)
result = 31 * result + (lastConnectionTime?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (platform?.hashCode() ?: 0)
result = 31 * result + (privateLinkConfig?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeAgentResponse
if (agentArn != other.agentArn) return false
if (creationTime != other.creationTime) return false
if (endpointType != other.endpointType) return false
if (lastConnectionTime != other.lastConnectionTime) return false
if (name != other.name) return false
if (platform != other.platform) return false
if (privateLinkConfig != other.privateLinkConfig) return false
if (status != other.status) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.DescribeAgentResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ARN of the agent.
*/
public var agentArn: kotlin.String? = null
/**
* The time that the agent was [activated](https://docs.aws.amazon.com/datasync/latest/userguide/activate-agent.html).
*/
public var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The type of [service endpoint](https://docs.aws.amazon.com/datasync/latest/userguide/choose-service-endpoint.html) that your agent is connected to.
*/
public var endpointType: aws.sdk.kotlin.services.datasync.model.EndpointType? = null
/**
* The last time that the agent was communicating with the DataSync service.
*/
public var lastConnectionTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The name of the agent.
*/
public var name: kotlin.String? = null
/**
* The platform-related details about the agent, such as the version number.
*/
public var platform: aws.sdk.kotlin.services.datasync.model.Platform? = null
/**
* The network configuration that the agent uses when connecting to a [VPC service endpoint](https://docs.aws.amazon.com/datasync/latest/userguide/choose-service-endpoint.html#choose-service-endpoint-vpc).
*/
public var privateLinkConfig: aws.sdk.kotlin.services.datasync.model.PrivateLinkConfig? = null
/**
* The status of the agent.
* + If the status is `ONLINE`, the agent is configured properly and ready to use.
* + If the status is `OFFLINE`, the agent has been out of contact with DataSync for five minutes or longer. This can happen for a few reasons. For more information, see [What do I do if my agent is offline?](https://docs.aws.amazon.com/datasync/latest/userguide/troubleshooting-datasync-agents.html#troubleshoot-agent-offline)
*/
public var status: aws.sdk.kotlin.services.datasync.model.AgentStatus? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.DescribeAgentResponse) : this() {
this.agentArn = x.agentArn
this.creationTime = x.creationTime
this.endpointType = x.endpointType
this.lastConnectionTime = x.lastConnectionTime
this.name = x.name
this.platform = x.platform
this.privateLinkConfig = x.privateLinkConfig
this.status = x.status
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.DescribeAgentResponse = DescribeAgentResponse(this)
/**
* construct an [aws.sdk.kotlin.services.datasync.model.Platform] inside the given [block]
*/
public fun platform(block: aws.sdk.kotlin.services.datasync.model.Platform.Builder.() -> kotlin.Unit) {
this.platform = aws.sdk.kotlin.services.datasync.model.Platform.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.datasync.model.PrivateLinkConfig] inside the given [block]
*/
public fun privateLinkConfig(block: aws.sdk.kotlin.services.datasync.model.PrivateLinkConfig.Builder.() -> kotlin.Unit) {
this.privateLinkConfig = aws.sdk.kotlin.services.datasync.model.PrivateLinkConfig.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy