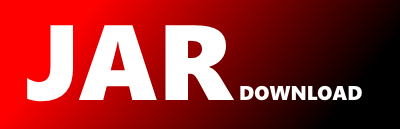
commonMain.aws.sdk.kotlin.services.datasync.model.DescribeLocationAzureBlobResponse.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
public class DescribeLocationAzureBlobResponse private constructor(builder: Builder) {
/**
* The access tier that you want your objects or files transferred into. This only applies when using the location as a transfer destination. For more information, see [Access tiers](https://docs.aws.amazon.com/datasync/latest/userguide/creating-azure-blob-location.html#azure-blob-access-tiers).
*/
public val accessTier: aws.sdk.kotlin.services.datasync.model.AzureAccessTier? = builder.accessTier
/**
* The ARNs of the DataSync agents that can connect with your Azure Blob Storage container.
*/
public val agentArns: List? = builder.agentArns
/**
* The authentication method DataSync uses to access your Azure Blob Storage. DataSync can access blob storage using a shared access signature (SAS).
*/
public val authenticationType: aws.sdk.kotlin.services.datasync.model.AzureBlobAuthenticationType? = builder.authenticationType
/**
* The type of blob that you want your objects or files to be when transferring them into Azure Blob Storage. Currently, DataSync only supports moving data into Azure Blob Storage as block blobs. For more information on blob types, see the [Azure Blob Storage documentation](https://learn.microsoft.com/en-us/rest/api/storageservices/understanding-block-blobs--append-blobs--and-page-blobs).
*/
public val blobType: aws.sdk.kotlin.services.datasync.model.AzureBlobType? = builder.blobType
/**
* The time that your Azure Blob Storage transfer location was created.
*/
public val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* The ARN of your Azure Blob Storage transfer location.
*/
public val locationArn: kotlin.String? = builder.locationArn
/**
* The URL of the Azure Blob Storage container involved in your transfer.
*/
public val locationUri: kotlin.String? = builder.locationUri
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.DescribeLocationAzureBlobResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeLocationAzureBlobResponse(")
append("accessTier=$accessTier,")
append("agentArns=$agentArns,")
append("authenticationType=$authenticationType,")
append("blobType=$blobType,")
append("creationTime=$creationTime,")
append("locationArn=$locationArn,")
append("locationUri=$locationUri")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = accessTier?.hashCode() ?: 0
result = 31 * result + (agentArns?.hashCode() ?: 0)
result = 31 * result + (authenticationType?.hashCode() ?: 0)
result = 31 * result + (blobType?.hashCode() ?: 0)
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (locationArn?.hashCode() ?: 0)
result = 31 * result + (locationUri?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeLocationAzureBlobResponse
if (accessTier != other.accessTier) return false
if (agentArns != other.agentArns) return false
if (authenticationType != other.authenticationType) return false
if (blobType != other.blobType) return false
if (creationTime != other.creationTime) return false
if (locationArn != other.locationArn) return false
if (locationUri != other.locationUri) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.DescribeLocationAzureBlobResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The access tier that you want your objects or files transferred into. This only applies when using the location as a transfer destination. For more information, see [Access tiers](https://docs.aws.amazon.com/datasync/latest/userguide/creating-azure-blob-location.html#azure-blob-access-tiers).
*/
public var accessTier: aws.sdk.kotlin.services.datasync.model.AzureAccessTier? = null
/**
* The ARNs of the DataSync agents that can connect with your Azure Blob Storage container.
*/
public var agentArns: List? = null
/**
* The authentication method DataSync uses to access your Azure Blob Storage. DataSync can access blob storage using a shared access signature (SAS).
*/
public var authenticationType: aws.sdk.kotlin.services.datasync.model.AzureBlobAuthenticationType? = null
/**
* The type of blob that you want your objects or files to be when transferring them into Azure Blob Storage. Currently, DataSync only supports moving data into Azure Blob Storage as block blobs. For more information on blob types, see the [Azure Blob Storage documentation](https://learn.microsoft.com/en-us/rest/api/storageservices/understanding-block-blobs--append-blobs--and-page-blobs).
*/
public var blobType: aws.sdk.kotlin.services.datasync.model.AzureBlobType? = null
/**
* The time that your Azure Blob Storage transfer location was created.
*/
public var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ARN of your Azure Blob Storage transfer location.
*/
public var locationArn: kotlin.String? = null
/**
* The URL of the Azure Blob Storage container involved in your transfer.
*/
public var locationUri: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.DescribeLocationAzureBlobResponse) : this() {
this.accessTier = x.accessTier
this.agentArns = x.agentArns
this.authenticationType = x.authenticationType
this.blobType = x.blobType
this.creationTime = x.creationTime
this.locationArn = x.locationArn
this.locationUri = x.locationUri
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.DescribeLocationAzureBlobResponse = DescribeLocationAzureBlobResponse(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy