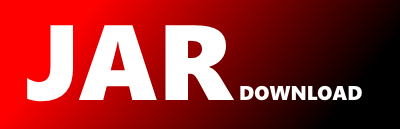
commonMain.aws.sdk.kotlin.services.datasync.model.DescribeLocationFsxOntapResponse.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
public class DescribeLocationFsxOntapResponse private constructor(builder: Builder) {
/**
* The time that the location was created.
*/
public val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* The ARN of the FSx for ONTAP file system.
*/
public val fsxFilesystemArn: kotlin.String? = builder.fsxFilesystemArn
/**
* The ARN of the FSx for ONTAP file system location.
*/
public val locationArn: kotlin.String? = builder.locationArn
/**
* The uniform resource identifier (URI) of the FSx for ONTAP file system location.
*/
public val locationUri: kotlin.String? = builder.locationUri
/**
* Specifies the data transfer protocol that DataSync uses to access your Amazon FSx file system.
*/
public val protocol: aws.sdk.kotlin.services.datasync.model.FsxProtocol? = builder.protocol
/**
* The security groups that DataSync uses to access your FSx for ONTAP file system.
*/
public val securityGroupArns: List? = builder.securityGroupArns
/**
* The ARN of the storage virtual machine (SVM) on your FSx for ONTAP file system where you're copying data to or from.
*/
public val storageVirtualMachineArn: kotlin.String? = builder.storageVirtualMachineArn
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.DescribeLocationFsxOntapResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeLocationFsxOntapResponse(")
append("creationTime=$creationTime,")
append("fsxFilesystemArn=$fsxFilesystemArn,")
append("locationArn=$locationArn,")
append("locationUri=$locationUri,")
append("protocol=$protocol,")
append("securityGroupArns=$securityGroupArns,")
append("storageVirtualMachineArn=$storageVirtualMachineArn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = creationTime?.hashCode() ?: 0
result = 31 * result + (fsxFilesystemArn?.hashCode() ?: 0)
result = 31 * result + (locationArn?.hashCode() ?: 0)
result = 31 * result + (locationUri?.hashCode() ?: 0)
result = 31 * result + (protocol?.hashCode() ?: 0)
result = 31 * result + (securityGroupArns?.hashCode() ?: 0)
result = 31 * result + (storageVirtualMachineArn?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeLocationFsxOntapResponse
if (creationTime != other.creationTime) return false
if (fsxFilesystemArn != other.fsxFilesystemArn) return false
if (locationArn != other.locationArn) return false
if (locationUri != other.locationUri) return false
if (protocol != other.protocol) return false
if (securityGroupArns != other.securityGroupArns) return false
if (storageVirtualMachineArn != other.storageVirtualMachineArn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.DescribeLocationFsxOntapResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The time that the location was created.
*/
public var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ARN of the FSx for ONTAP file system.
*/
public var fsxFilesystemArn: kotlin.String? = null
/**
* The ARN of the FSx for ONTAP file system location.
*/
public var locationArn: kotlin.String? = null
/**
* The uniform resource identifier (URI) of the FSx for ONTAP file system location.
*/
public var locationUri: kotlin.String? = null
/**
* Specifies the data transfer protocol that DataSync uses to access your Amazon FSx file system.
*/
public var protocol: aws.sdk.kotlin.services.datasync.model.FsxProtocol? = null
/**
* The security groups that DataSync uses to access your FSx for ONTAP file system.
*/
public var securityGroupArns: List? = null
/**
* The ARN of the storage virtual machine (SVM) on your FSx for ONTAP file system where you're copying data to or from.
*/
public var storageVirtualMachineArn: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.DescribeLocationFsxOntapResponse) : this() {
this.creationTime = x.creationTime
this.fsxFilesystemArn = x.fsxFilesystemArn
this.locationArn = x.locationArn
this.locationUri = x.locationUri
this.protocol = x.protocol
this.securityGroupArns = x.securityGroupArns
this.storageVirtualMachineArn = x.storageVirtualMachineArn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.DescribeLocationFsxOntapResponse = DescribeLocationFsxOntapResponse(this)
/**
* construct an [aws.sdk.kotlin.services.datasync.model.FsxProtocol] inside the given [block]
*/
public fun protocol(block: aws.sdk.kotlin.services.datasync.model.FsxProtocol.Builder.() -> kotlin.Unit) {
this.protocol = aws.sdk.kotlin.services.datasync.model.FsxProtocol.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy