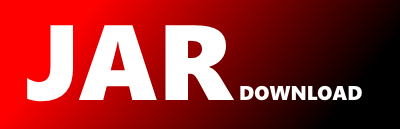
commonMain.aws.sdk.kotlin.services.datasync.model.DescribeLocationS3Response.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* DescribeLocationS3Response
*/
public class DescribeLocationS3Response private constructor(builder: Builder) {
/**
* The ARNs of the DataSync agents deployed on your Outpost when using working with Amazon S3 on Outposts.
*
* For more information, see [Deploy your DataSync agent on Outposts](https://docs.aws.amazon.com/datasync/latest/userguide/deploy-agents.html#outposts-agent).
*/
public val agentArns: List? = builder.agentArns
/**
* The time that the Amazon S3 location was created.
*/
public val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* The ARN of the Amazon S3 location.
*/
public val locationArn: kotlin.String? = builder.locationArn
/**
* The URL of the Amazon S3 location that was described.
*/
public val locationUri: kotlin.String? = builder.locationUri
/**
* Specifies the Amazon Resource Name (ARN) of the Identity and Access Management (IAM) role that DataSync uses to access your S3 bucket.
*
* For more information, see [Accessing S3 buckets](https://docs.aws.amazon.com/datasync/latest/userguide/create-s3-location.html#create-s3-location-access).
*/
public val s3Config: aws.sdk.kotlin.services.datasync.model.S3Config? = builder.s3Config
/**
* When Amazon S3 is a destination location, this is the storage class that you chose for your objects.
*
* Some storage classes have behaviors that can affect your Amazon S3 storage costs. For more information, see [Storage class considerations with Amazon S3 transfers](https://docs.aws.amazon.com/datasync/latest/userguide/create-s3-location.html#using-storage-classes).
*/
public val s3StorageClass: aws.sdk.kotlin.services.datasync.model.S3StorageClass? = builder.s3StorageClass
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.DescribeLocationS3Response = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeLocationS3Response(")
append("agentArns=$agentArns,")
append("creationTime=$creationTime,")
append("locationArn=$locationArn,")
append("locationUri=$locationUri,")
append("s3Config=$s3Config,")
append("s3StorageClass=$s3StorageClass")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = agentArns?.hashCode() ?: 0
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (locationArn?.hashCode() ?: 0)
result = 31 * result + (locationUri?.hashCode() ?: 0)
result = 31 * result + (s3Config?.hashCode() ?: 0)
result = 31 * result + (s3StorageClass?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeLocationS3Response
if (agentArns != other.agentArns) return false
if (creationTime != other.creationTime) return false
if (locationArn != other.locationArn) return false
if (locationUri != other.locationUri) return false
if (s3Config != other.s3Config) return false
if (s3StorageClass != other.s3StorageClass) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.DescribeLocationS3Response = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ARNs of the DataSync agents deployed on your Outpost when using working with Amazon S3 on Outposts.
*
* For more information, see [Deploy your DataSync agent on Outposts](https://docs.aws.amazon.com/datasync/latest/userguide/deploy-agents.html#outposts-agent).
*/
public var agentArns: List? = null
/**
* The time that the Amazon S3 location was created.
*/
public var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ARN of the Amazon S3 location.
*/
public var locationArn: kotlin.String? = null
/**
* The URL of the Amazon S3 location that was described.
*/
public var locationUri: kotlin.String? = null
/**
* Specifies the Amazon Resource Name (ARN) of the Identity and Access Management (IAM) role that DataSync uses to access your S3 bucket.
*
* For more information, see [Accessing S3 buckets](https://docs.aws.amazon.com/datasync/latest/userguide/create-s3-location.html#create-s3-location-access).
*/
public var s3Config: aws.sdk.kotlin.services.datasync.model.S3Config? = null
/**
* When Amazon S3 is a destination location, this is the storage class that you chose for your objects.
*
* Some storage classes have behaviors that can affect your Amazon S3 storage costs. For more information, see [Storage class considerations with Amazon S3 transfers](https://docs.aws.amazon.com/datasync/latest/userguide/create-s3-location.html#using-storage-classes).
*/
public var s3StorageClass: aws.sdk.kotlin.services.datasync.model.S3StorageClass? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.DescribeLocationS3Response) : this() {
this.agentArns = x.agentArns
this.creationTime = x.creationTime
this.locationArn = x.locationArn
this.locationUri = x.locationUri
this.s3Config = x.s3Config
this.s3StorageClass = x.s3StorageClass
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.DescribeLocationS3Response = DescribeLocationS3Response(this)
/**
* construct an [aws.sdk.kotlin.services.datasync.model.S3Config] inside the given [block]
*/
public fun s3Config(block: aws.sdk.kotlin.services.datasync.model.S3Config.Builder.() -> kotlin.Unit) {
this.s3Config = aws.sdk.kotlin.services.datasync.model.S3Config.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy