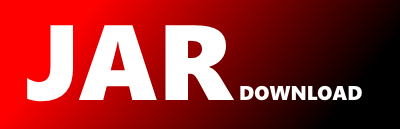
commonMain.aws.sdk.kotlin.services.datasync.model.Ec2Config.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* The subnet and security groups that DataSync uses to connect to one of your Amazon EFS file system's [mount targets](https://docs.aws.amazon.com/efs/latest/ug/accessing-fs.html).
*/
public class Ec2Config private constructor(builder: Builder) {
/**
* Specifies the Amazon Resource Names (ARNs) of the security groups associated with an Amazon EFS file system's mount target.
*/
public val securityGroupArns: List = requireNotNull(builder.securityGroupArns) { "A non-null value must be provided for securityGroupArns" }
/**
* Specifies the ARN of a subnet where DataSync creates the [network interfaces](https://docs.aws.amazon.com/datasync/latest/userguide/datasync-network.html#required-network-interfaces) for managing traffic during your transfer.
*
* The subnet must be located:
* + In the same virtual private cloud (VPC) as the Amazon EFS file system.
* + In the same Availability Zone as at least one mount target for the Amazon EFS file system.
*
* You don't need to specify a subnet that includes a file system mount target.
*/
public val subnetArn: kotlin.String = requireNotNull(builder.subnetArn) { "A non-null value must be provided for subnetArn" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.Ec2Config = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Ec2Config(")
append("securityGroupArns=$securityGroupArns,")
append("subnetArn=$subnetArn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = securityGroupArns.hashCode()
result = 31 * result + (subnetArn.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Ec2Config
if (securityGroupArns != other.securityGroupArns) return false
if (subnetArn != other.subnetArn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.Ec2Config = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Specifies the Amazon Resource Names (ARNs) of the security groups associated with an Amazon EFS file system's mount target.
*/
public var securityGroupArns: List? = null
/**
* Specifies the ARN of a subnet where DataSync creates the [network interfaces](https://docs.aws.amazon.com/datasync/latest/userguide/datasync-network.html#required-network-interfaces) for managing traffic during your transfer.
*
* The subnet must be located:
* + In the same virtual private cloud (VPC) as the Amazon EFS file system.
* + In the same Availability Zone as at least one mount target for the Amazon EFS file system.
*
* You don't need to specify a subnet that includes a file system mount target.
*/
public var subnetArn: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.Ec2Config) : this() {
this.securityGroupArns = x.securityGroupArns
this.subnetArn = x.subnetArn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.Ec2Config = Ec2Config(this)
internal fun correctErrors(): Builder {
if (securityGroupArns == null) securityGroupArns = emptyList()
if (subnetArn == null) subnetArn = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy