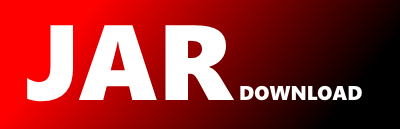
commonMain.aws.sdk.kotlin.services.datasync.model.NetAppOntapCluster.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* The information that DataSync Discovery collects about an on-premises storage system cluster.
*/
public class NetAppOntapCluster private constructor(builder: Builder) {
/**
* The number of CIFS shares in the cluster.
*/
public val cifsShareCount: kotlin.Long? = builder.cifsShareCount
/**
* The storage space that's being used in the cluster without accounting for compression or deduplication.
*/
public val clusterBlockStorageLogicalUsed: kotlin.Long? = builder.clusterBlockStorageLogicalUsed
/**
* The total storage space that's available in the cluster.
*/
public val clusterBlockStorageSize: kotlin.Long? = builder.clusterBlockStorageSize
/**
* The storage space that's being used in a cluster.
*/
public val clusterBlockStorageUsed: kotlin.Long? = builder.clusterBlockStorageUsed
/**
* The amount of space in the cluster that's in cloud storage (for example, if you're using data tiering).
*/
public val clusterCloudStorageUsed: kotlin.Long? = builder.clusterCloudStorageUsed
/**
* The name of the cluster.
*/
public val clusterName: kotlin.String? = builder.clusterName
/**
* The number of LUNs (logical unit numbers) in the cluster.
*/
public val lunCount: kotlin.Long? = builder.lunCount
/**
* The performance data that DataSync Discovery collects about the cluster.
*/
public val maxP95Performance: aws.sdk.kotlin.services.datasync.model.MaxP95Performance? = builder.maxP95Performance
/**
* The number of NFS volumes in the cluster.
*/
public val nfsExportedVolumes: kotlin.Long? = builder.nfsExportedVolumes
/**
* Indicates whether DataSync Discovery recommendations for the cluster are ready to view, incomplete, or can't be determined.
*
* For more information, see [Recommendation statuses](https://docs.aws.amazon.com/datasync/latest/userguide/discovery-job-statuses.html#recommendation-statuses-table).
*/
public val recommendationStatus: aws.sdk.kotlin.services.datasync.model.RecommendationStatus? = builder.recommendationStatus
/**
* The Amazon Web Services storage services that DataSync Discovery recommends for the cluster. For more information, see [Recommendations provided by DataSync Discovery](https://docs.aws.amazon.com/datasync/latest/userguide/discovery-understand-recommendations.html).
*/
public val recommendations: List? = builder.recommendations
/**
* The universally unique identifier (UUID) of the cluster.
*/
public val resourceId: kotlin.String? = builder.resourceId
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.NetAppOntapCluster = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("NetAppOntapCluster(")
append("cifsShareCount=$cifsShareCount,")
append("clusterBlockStorageLogicalUsed=$clusterBlockStorageLogicalUsed,")
append("clusterBlockStorageSize=$clusterBlockStorageSize,")
append("clusterBlockStorageUsed=$clusterBlockStorageUsed,")
append("clusterCloudStorageUsed=$clusterCloudStorageUsed,")
append("clusterName=$clusterName,")
append("lunCount=$lunCount,")
append("maxP95Performance=$maxP95Performance,")
append("nfsExportedVolumes=$nfsExportedVolumes,")
append("recommendationStatus=$recommendationStatus,")
append("recommendations=$recommendations,")
append("resourceId=$resourceId")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = cifsShareCount?.hashCode() ?: 0
result = 31 * result + (clusterBlockStorageLogicalUsed?.hashCode() ?: 0)
result = 31 * result + (clusterBlockStorageSize?.hashCode() ?: 0)
result = 31 * result + (clusterBlockStorageUsed?.hashCode() ?: 0)
result = 31 * result + (clusterCloudStorageUsed?.hashCode() ?: 0)
result = 31 * result + (clusterName?.hashCode() ?: 0)
result = 31 * result + (lunCount?.hashCode() ?: 0)
result = 31 * result + (maxP95Performance?.hashCode() ?: 0)
result = 31 * result + (nfsExportedVolumes?.hashCode() ?: 0)
result = 31 * result + (recommendationStatus?.hashCode() ?: 0)
result = 31 * result + (recommendations?.hashCode() ?: 0)
result = 31 * result + (resourceId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as NetAppOntapCluster
if (cifsShareCount != other.cifsShareCount) return false
if (clusterBlockStorageLogicalUsed != other.clusterBlockStorageLogicalUsed) return false
if (clusterBlockStorageSize != other.clusterBlockStorageSize) return false
if (clusterBlockStorageUsed != other.clusterBlockStorageUsed) return false
if (clusterCloudStorageUsed != other.clusterCloudStorageUsed) return false
if (clusterName != other.clusterName) return false
if (lunCount != other.lunCount) return false
if (maxP95Performance != other.maxP95Performance) return false
if (nfsExportedVolumes != other.nfsExportedVolumes) return false
if (recommendationStatus != other.recommendationStatus) return false
if (recommendations != other.recommendations) return false
if (resourceId != other.resourceId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.NetAppOntapCluster = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The number of CIFS shares in the cluster.
*/
public var cifsShareCount: kotlin.Long? = null
/**
* The storage space that's being used in the cluster without accounting for compression or deduplication.
*/
public var clusterBlockStorageLogicalUsed: kotlin.Long? = null
/**
* The total storage space that's available in the cluster.
*/
public var clusterBlockStorageSize: kotlin.Long? = null
/**
* The storage space that's being used in a cluster.
*/
public var clusterBlockStorageUsed: kotlin.Long? = null
/**
* The amount of space in the cluster that's in cloud storage (for example, if you're using data tiering).
*/
public var clusterCloudStorageUsed: kotlin.Long? = null
/**
* The name of the cluster.
*/
public var clusterName: kotlin.String? = null
/**
* The number of LUNs (logical unit numbers) in the cluster.
*/
public var lunCount: kotlin.Long? = null
/**
* The performance data that DataSync Discovery collects about the cluster.
*/
public var maxP95Performance: aws.sdk.kotlin.services.datasync.model.MaxP95Performance? = null
/**
* The number of NFS volumes in the cluster.
*/
public var nfsExportedVolumes: kotlin.Long? = null
/**
* Indicates whether DataSync Discovery recommendations for the cluster are ready to view, incomplete, or can't be determined.
*
* For more information, see [Recommendation statuses](https://docs.aws.amazon.com/datasync/latest/userguide/discovery-job-statuses.html#recommendation-statuses-table).
*/
public var recommendationStatus: aws.sdk.kotlin.services.datasync.model.RecommendationStatus? = null
/**
* The Amazon Web Services storage services that DataSync Discovery recommends for the cluster. For more information, see [Recommendations provided by DataSync Discovery](https://docs.aws.amazon.com/datasync/latest/userguide/discovery-understand-recommendations.html).
*/
public var recommendations: List? = null
/**
* The universally unique identifier (UUID) of the cluster.
*/
public var resourceId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.NetAppOntapCluster) : this() {
this.cifsShareCount = x.cifsShareCount
this.clusterBlockStorageLogicalUsed = x.clusterBlockStorageLogicalUsed
this.clusterBlockStorageSize = x.clusterBlockStorageSize
this.clusterBlockStorageUsed = x.clusterBlockStorageUsed
this.clusterCloudStorageUsed = x.clusterCloudStorageUsed
this.clusterName = x.clusterName
this.lunCount = x.lunCount
this.maxP95Performance = x.maxP95Performance
this.nfsExportedVolumes = x.nfsExportedVolumes
this.recommendationStatus = x.recommendationStatus
this.recommendations = x.recommendations
this.resourceId = x.resourceId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.NetAppOntapCluster = NetAppOntapCluster(this)
/**
* construct an [aws.sdk.kotlin.services.datasync.model.MaxP95Performance] inside the given [block]
*/
public fun maxP95Performance(block: aws.sdk.kotlin.services.datasync.model.MaxP95Performance.Builder.() -> kotlin.Unit) {
this.maxP95Performance = aws.sdk.kotlin.services.datasync.model.MaxP95Performance.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy