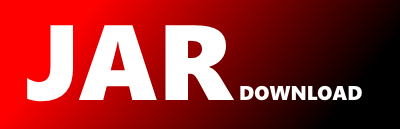
commonMain.aws.sdk.kotlin.services.datasync.model.TaskReportConfig.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datasync.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Specifies how you want to configure a task report, which provides detailed information about for your DataSync transfer.
*
* For more information, see [Task reports](https://docs.aws.amazon.com/datasync/latest/userguide/task-reports.html).
*/
public class TaskReportConfig private constructor(builder: Builder) {
/**
* Specifies the Amazon S3 bucket where DataSync uploads your task report. For more information, see [Task reports](https://docs.aws.amazon.com/datasync/latest/userguide/task-reports.html#task-report-access).
*/
public val destination: aws.sdk.kotlin.services.datasync.model.ReportDestination? = builder.destination
/**
* Specifies whether your task report includes the new version of each object transferred into an S3 bucket. This only applies if you [enable versioning on your bucket](https://docs.aws.amazon.com/AmazonS3/latest/userguide/manage-versioning-examples.html). Keep in mind that setting this to `INCLUDE` can increase the duration of your task execution.
*/
public val objectVersionIds: aws.sdk.kotlin.services.datasync.model.ObjectVersionIds? = builder.objectVersionIds
/**
* Specifies the type of task report that you want:
* + `SUMMARY_ONLY`: Provides necessary details about your task, including the number of files, objects, and directories transferred and transfer duration.
* + `STANDARD`: Provides complete details about your task, including a full list of files, objects, and directories that were transferred, skipped, verified, and more.
*/
public val outputType: aws.sdk.kotlin.services.datasync.model.ReportOutputType? = builder.outputType
/**
* Customizes the reporting level for aspects of your task report. For example, your report might generally only include errors, but you could specify that you want a list of successes and errors just for the files that DataSync attempted to delete in your destination location.
*/
public val overrides: aws.sdk.kotlin.services.datasync.model.ReportOverrides? = builder.overrides
/**
* Specifies whether you want your task report to include only what went wrong with your transfer or a list of what succeeded and didn't.
* + `ERRORS_ONLY`: A report shows what DataSync was unable to transfer, skip, verify, and delete.
* + `SUCCESSES_AND_ERRORS`: A report shows what DataSync was able and unable to transfer, skip, verify, and delete.
*/
public val reportLevel: aws.sdk.kotlin.services.datasync.model.ReportLevel? = builder.reportLevel
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datasync.model.TaskReportConfig = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("TaskReportConfig(")
append("destination=$destination,")
append("objectVersionIds=$objectVersionIds,")
append("outputType=$outputType,")
append("overrides=$overrides,")
append("reportLevel=$reportLevel")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = destination?.hashCode() ?: 0
result = 31 * result + (objectVersionIds?.hashCode() ?: 0)
result = 31 * result + (outputType?.hashCode() ?: 0)
result = 31 * result + (overrides?.hashCode() ?: 0)
result = 31 * result + (reportLevel?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as TaskReportConfig
if (destination != other.destination) return false
if (objectVersionIds != other.objectVersionIds) return false
if (outputType != other.outputType) return false
if (overrides != other.overrides) return false
if (reportLevel != other.reportLevel) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datasync.model.TaskReportConfig = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Specifies the Amazon S3 bucket where DataSync uploads your task report. For more information, see [Task reports](https://docs.aws.amazon.com/datasync/latest/userguide/task-reports.html#task-report-access).
*/
public var destination: aws.sdk.kotlin.services.datasync.model.ReportDestination? = null
/**
* Specifies whether your task report includes the new version of each object transferred into an S3 bucket. This only applies if you [enable versioning on your bucket](https://docs.aws.amazon.com/AmazonS3/latest/userguide/manage-versioning-examples.html). Keep in mind that setting this to `INCLUDE` can increase the duration of your task execution.
*/
public var objectVersionIds: aws.sdk.kotlin.services.datasync.model.ObjectVersionIds? = null
/**
* Specifies the type of task report that you want:
* + `SUMMARY_ONLY`: Provides necessary details about your task, including the number of files, objects, and directories transferred and transfer duration.
* + `STANDARD`: Provides complete details about your task, including a full list of files, objects, and directories that were transferred, skipped, verified, and more.
*/
public var outputType: aws.sdk.kotlin.services.datasync.model.ReportOutputType? = null
/**
* Customizes the reporting level for aspects of your task report. For example, your report might generally only include errors, but you could specify that you want a list of successes and errors just for the files that DataSync attempted to delete in your destination location.
*/
public var overrides: aws.sdk.kotlin.services.datasync.model.ReportOverrides? = null
/**
* Specifies whether you want your task report to include only what went wrong with your transfer or a list of what succeeded and didn't.
* + `ERRORS_ONLY`: A report shows what DataSync was unable to transfer, skip, verify, and delete.
* + `SUCCESSES_AND_ERRORS`: A report shows what DataSync was able and unable to transfer, skip, verify, and delete.
*/
public var reportLevel: aws.sdk.kotlin.services.datasync.model.ReportLevel? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datasync.model.TaskReportConfig) : this() {
this.destination = x.destination
this.objectVersionIds = x.objectVersionIds
this.outputType = x.outputType
this.overrides = x.overrides
this.reportLevel = x.reportLevel
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datasync.model.TaskReportConfig = TaskReportConfig(this)
/**
* construct an [aws.sdk.kotlin.services.datasync.model.ReportDestination] inside the given [block]
*/
public fun destination(block: aws.sdk.kotlin.services.datasync.model.ReportDestination.Builder.() -> kotlin.Unit) {
this.destination = aws.sdk.kotlin.services.datasync.model.ReportDestination.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.datasync.model.ReportOverrides] inside the given [block]
*/
public fun overrides(block: aws.sdk.kotlin.services.datasync.model.ReportOverrides.Builder.() -> kotlin.Unit) {
this.overrides = aws.sdk.kotlin.services.datasync.model.ReportOverrides.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy