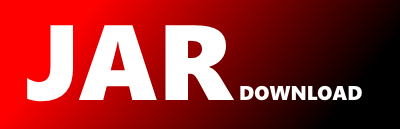
commonMain.aws.sdk.kotlin.services.datazone.model.AcceptSubscriptionRequestResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datazone-jvm Show documentation
Show all versions of datazone-jvm Show documentation
The AWS SDK for Kotlin client for DataZone
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datazone.model
import aws.smithy.kotlin.runtime.time.Instant
public class AcceptSubscriptionRequestResponse private constructor(builder: Builder) {
/**
* The timestamp that specifies when the subscription request was accepted.
*/
public val createdAt: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.createdAt) { "A non-null value must be provided for createdAt" }
/**
* Specifies the Amazon DataZone user that accepted the specified subscription request.
*/
public val createdBy: kotlin.String = requireNotNull(builder.createdBy) { "A non-null value must be provided for createdBy" }
/**
* Specifies the reason for accepting the subscription request.
*/
public val decisionComment: kotlin.String? = builder.decisionComment
/**
* The unique identifier of the Amazon DataZone domain where the specified subscription request was accepted.
*/
public val domainId: kotlin.String = requireNotNull(builder.domainId) { "A non-null value must be provided for domainId" }
/**
* The identifier of the subscription request.
*/
public val id: kotlin.String = requireNotNull(builder.id) { "A non-null value must be provided for id" }
/**
* Specifies the reason for requesting a subscription to the asset.
*/
public val requestReason: kotlin.String = requireNotNull(builder.requestReason) { "A non-null value must be provided for requestReason" }
/**
* Specifes the ID of the Amazon DataZone user who reviewed the subscription request.
*/
public val reviewerId: kotlin.String? = builder.reviewerId
/**
* Specifies the status of the subscription request.
*/
public val status: aws.sdk.kotlin.services.datazone.model.SubscriptionRequestStatus = requireNotNull(builder.status) { "A non-null value must be provided for status" }
/**
* Specifies the asset for which the subscription request was created.
*/
public val subscribedListings: List = requireNotNull(builder.subscribedListings) { "A non-null value must be provided for subscribedListings" }
/**
* Specifies the Amazon DataZone users who are subscribed to the asset specified in the subscription request.
*/
public val subscribedPrincipals: List = requireNotNull(builder.subscribedPrincipals) { "A non-null value must be provided for subscribedPrincipals" }
/**
* Specifies the timestamp when subscription request was updated.
*/
public val updatedAt: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.updatedAt) { "A non-null value must be provided for updatedAt" }
/**
* Specifies the Amazon DataZone user who updated the subscription request.
*/
public val updatedBy: kotlin.String? = builder.updatedBy
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datazone.model.AcceptSubscriptionRequestResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("AcceptSubscriptionRequestResponse(")
append("createdAt=$createdAt,")
append("createdBy=$createdBy,")
append("decisionComment=*** Sensitive Data Redacted ***,")
append("domainId=$domainId,")
append("id=$id,")
append("requestReason=*** Sensitive Data Redacted ***,")
append("reviewerId=$reviewerId,")
append("status=$status,")
append("subscribedListings=$subscribedListings,")
append("subscribedPrincipals=$subscribedPrincipals,")
append("updatedAt=$updatedAt,")
append("updatedBy=$updatedBy")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = createdAt.hashCode()
result = 31 * result + (createdBy.hashCode())
result = 31 * result + (decisionComment?.hashCode() ?: 0)
result = 31 * result + (domainId.hashCode())
result = 31 * result + (id.hashCode())
result = 31 * result + (requestReason.hashCode())
result = 31 * result + (reviewerId?.hashCode() ?: 0)
result = 31 * result + (status.hashCode())
result = 31 * result + (subscribedListings.hashCode())
result = 31 * result + (subscribedPrincipals.hashCode())
result = 31 * result + (updatedAt.hashCode())
result = 31 * result + (updatedBy?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AcceptSubscriptionRequestResponse
if (createdAt != other.createdAt) return false
if (createdBy != other.createdBy) return false
if (decisionComment != other.decisionComment) return false
if (domainId != other.domainId) return false
if (id != other.id) return false
if (requestReason != other.requestReason) return false
if (reviewerId != other.reviewerId) return false
if (status != other.status) return false
if (subscribedListings != other.subscribedListings) return false
if (subscribedPrincipals != other.subscribedPrincipals) return false
if (updatedAt != other.updatedAt) return false
if (updatedBy != other.updatedBy) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datazone.model.AcceptSubscriptionRequestResponse = Builder(this).apply(block).build()
public class Builder {
/**
* The timestamp that specifies when the subscription request was accepted.
*/
public var createdAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Specifies the Amazon DataZone user that accepted the specified subscription request.
*/
public var createdBy: kotlin.String? = null
/**
* Specifies the reason for accepting the subscription request.
*/
public var decisionComment: kotlin.String? = null
/**
* The unique identifier of the Amazon DataZone domain where the specified subscription request was accepted.
*/
public var domainId: kotlin.String? = null
/**
* The identifier of the subscription request.
*/
public var id: kotlin.String? = null
/**
* Specifies the reason for requesting a subscription to the asset.
*/
public var requestReason: kotlin.String? = null
/**
* Specifes the ID of the Amazon DataZone user who reviewed the subscription request.
*/
public var reviewerId: kotlin.String? = null
/**
* Specifies the status of the subscription request.
*/
public var status: aws.sdk.kotlin.services.datazone.model.SubscriptionRequestStatus? = null
/**
* Specifies the asset for which the subscription request was created.
*/
public var subscribedListings: List? = null
/**
* Specifies the Amazon DataZone users who are subscribed to the asset specified in the subscription request.
*/
public var subscribedPrincipals: List? = null
/**
* Specifies the timestamp when subscription request was updated.
*/
public var updatedAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Specifies the Amazon DataZone user who updated the subscription request.
*/
public var updatedBy: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datazone.model.AcceptSubscriptionRequestResponse) : this() {
this.createdAt = x.createdAt
this.createdBy = x.createdBy
this.decisionComment = x.decisionComment
this.domainId = x.domainId
this.id = x.id
this.requestReason = x.requestReason
this.reviewerId = x.reviewerId
this.status = x.status
this.subscribedListings = x.subscribedListings
this.subscribedPrincipals = x.subscribedPrincipals
this.updatedAt = x.updatedAt
this.updatedBy = x.updatedBy
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datazone.model.AcceptSubscriptionRequestResponse = AcceptSubscriptionRequestResponse(this)
internal fun correctErrors(): Builder {
if (createdAt == null) createdAt = Instant.fromEpochSeconds(0)
if (createdBy == null) createdBy = ""
if (domainId == null) domainId = ""
if (id == null) id = ""
if (requestReason == null) requestReason = ""
if (status == null) status = SubscriptionRequestStatus.SdkUnknown("no value provided")
if (subscribedListings == null) subscribedListings = emptyList()
if (subscribedPrincipals == null) subscribedPrincipals = emptyList()
if (updatedAt == null) updatedAt = Instant.fromEpochSeconds(0)
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy