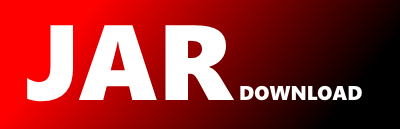
commonMain.aws.sdk.kotlin.services.datazone.model.DataSourceSummary.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datazone-jvm Show documentation
Show all versions of datazone-jvm Show documentation
The AWS SDK for Kotlin client for DataZone
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datazone.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* The details of the data source.
*/
public class DataSourceSummary private constructor(builder: Builder) {
/**
* The timestamp of when the data source was created.
*/
public val createdAt: aws.smithy.kotlin.runtime.time.Instant? = builder.createdAt
/**
* The ID of the data source.
*/
public val dataSourceId: kotlin.String = requireNotNull(builder.dataSourceId) { "A non-null value must be provided for dataSourceId" }
/**
* The ID of the Amazon DataZone domain in which the data source exists.
*/
public val domainId: kotlin.String = requireNotNull(builder.domainId) { "A non-null value must be provided for domainId" }
/**
* Specifies whether the data source is enabled.
*/
public val enableSetting: aws.sdk.kotlin.services.datazone.model.EnableSetting? = builder.enableSetting
/**
* The ID of the environment in which the data source exists.
*/
public val environmentId: kotlin.String = requireNotNull(builder.environmentId) { "A non-null value must be provided for environmentId" }
/**
* The count of the assets created during the last data source run.
*/
public val lastRunAssetCount: kotlin.Int? = builder.lastRunAssetCount
/**
* The timestamp of when the data source run was last performed.
*/
public val lastRunAt: aws.smithy.kotlin.runtime.time.Instant? = builder.lastRunAt
/**
* The details of the error message that is returned if the operation cannot be successfully completed.
*/
public val lastRunErrorMessage: aws.sdk.kotlin.services.datazone.model.DataSourceErrorMessage? = builder.lastRunErrorMessage
/**
* The status of the last data source run.
*/
public val lastRunStatus: aws.sdk.kotlin.services.datazone.model.DataSourceRunStatus? = builder.lastRunStatus
/**
* The name of the data source.
*/
public val name: kotlin.String = requireNotNull(builder.name) { "A non-null value must be provided for name" }
/**
* The details of the schedule of the data source runs.
*/
public val schedule: aws.sdk.kotlin.services.datazone.model.ScheduleConfiguration? = builder.schedule
/**
* The status of the data source.
*/
public val status: aws.sdk.kotlin.services.datazone.model.DataSourceStatus = requireNotNull(builder.status) { "A non-null value must be provided for status" }
/**
* The type of the data source.
*/
public val type: kotlin.String = requireNotNull(builder.type) { "A non-null value must be provided for type" }
/**
* The timestamp of when the data source was updated.
*/
public val updatedAt: aws.smithy.kotlin.runtime.time.Instant? = builder.updatedAt
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datazone.model.DataSourceSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DataSourceSummary(")
append("createdAt=$createdAt,")
append("dataSourceId=$dataSourceId,")
append("domainId=$domainId,")
append("enableSetting=$enableSetting,")
append("environmentId=$environmentId,")
append("lastRunAssetCount=$lastRunAssetCount,")
append("lastRunAt=$lastRunAt,")
append("lastRunErrorMessage=$lastRunErrorMessage,")
append("lastRunStatus=$lastRunStatus,")
append("name=*** Sensitive Data Redacted ***,")
append("schedule=*** Sensitive Data Redacted ***,")
append("status=$status,")
append("type=$type,")
append("updatedAt=$updatedAt")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = createdAt?.hashCode() ?: 0
result = 31 * result + (dataSourceId.hashCode())
result = 31 * result + (domainId.hashCode())
result = 31 * result + (enableSetting?.hashCode() ?: 0)
result = 31 * result + (environmentId.hashCode())
result = 31 * result + (lastRunAssetCount ?: 0)
result = 31 * result + (lastRunAt?.hashCode() ?: 0)
result = 31 * result + (lastRunErrorMessage?.hashCode() ?: 0)
result = 31 * result + (lastRunStatus?.hashCode() ?: 0)
result = 31 * result + (name.hashCode())
result = 31 * result + (schedule?.hashCode() ?: 0)
result = 31 * result + (status.hashCode())
result = 31 * result + (type.hashCode())
result = 31 * result + (updatedAt?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DataSourceSummary
if (createdAt != other.createdAt) return false
if (dataSourceId != other.dataSourceId) return false
if (domainId != other.domainId) return false
if (enableSetting != other.enableSetting) return false
if (environmentId != other.environmentId) return false
if (lastRunAssetCount != other.lastRunAssetCount) return false
if (lastRunAt != other.lastRunAt) return false
if (lastRunErrorMessage != other.lastRunErrorMessage) return false
if (lastRunStatus != other.lastRunStatus) return false
if (name != other.name) return false
if (schedule != other.schedule) return false
if (status != other.status) return false
if (type != other.type) return false
if (updatedAt != other.updatedAt) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datazone.model.DataSourceSummary = Builder(this).apply(block).build()
public class Builder {
/**
* The timestamp of when the data source was created.
*/
public var createdAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ID of the data source.
*/
public var dataSourceId: kotlin.String? = null
/**
* The ID of the Amazon DataZone domain in which the data source exists.
*/
public var domainId: kotlin.String? = null
/**
* Specifies whether the data source is enabled.
*/
public var enableSetting: aws.sdk.kotlin.services.datazone.model.EnableSetting? = null
/**
* The ID of the environment in which the data source exists.
*/
public var environmentId: kotlin.String? = null
/**
* The count of the assets created during the last data source run.
*/
public var lastRunAssetCount: kotlin.Int? = null
/**
* The timestamp of when the data source run was last performed.
*/
public var lastRunAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The details of the error message that is returned if the operation cannot be successfully completed.
*/
public var lastRunErrorMessage: aws.sdk.kotlin.services.datazone.model.DataSourceErrorMessage? = null
/**
* The status of the last data source run.
*/
public var lastRunStatus: aws.sdk.kotlin.services.datazone.model.DataSourceRunStatus? = null
/**
* The name of the data source.
*/
public var name: kotlin.String? = null
/**
* The details of the schedule of the data source runs.
*/
public var schedule: aws.sdk.kotlin.services.datazone.model.ScheduleConfiguration? = null
/**
* The status of the data source.
*/
public var status: aws.sdk.kotlin.services.datazone.model.DataSourceStatus? = null
/**
* The type of the data source.
*/
public var type: kotlin.String? = null
/**
* The timestamp of when the data source was updated.
*/
public var updatedAt: aws.smithy.kotlin.runtime.time.Instant? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datazone.model.DataSourceSummary) : this() {
this.createdAt = x.createdAt
this.dataSourceId = x.dataSourceId
this.domainId = x.domainId
this.enableSetting = x.enableSetting
this.environmentId = x.environmentId
this.lastRunAssetCount = x.lastRunAssetCount
this.lastRunAt = x.lastRunAt
this.lastRunErrorMessage = x.lastRunErrorMessage
this.lastRunStatus = x.lastRunStatus
this.name = x.name
this.schedule = x.schedule
this.status = x.status
this.type = x.type
this.updatedAt = x.updatedAt
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datazone.model.DataSourceSummary = DataSourceSummary(this)
/**
* construct an [aws.sdk.kotlin.services.datazone.model.DataSourceErrorMessage] inside the given [block]
*/
public fun lastRunErrorMessage(block: aws.sdk.kotlin.services.datazone.model.DataSourceErrorMessage.Builder.() -> kotlin.Unit) {
this.lastRunErrorMessage = aws.sdk.kotlin.services.datazone.model.DataSourceErrorMessage.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.datazone.model.ScheduleConfiguration] inside the given [block]
*/
public fun schedule(block: aws.sdk.kotlin.services.datazone.model.ScheduleConfiguration.Builder.() -> kotlin.Unit) {
this.schedule = aws.sdk.kotlin.services.datazone.model.ScheduleConfiguration.invoke(block)
}
internal fun correctErrors(): Builder {
if (dataSourceId == null) dataSourceId = ""
if (domainId == null) domainId = ""
if (environmentId == null) environmentId = ""
if (name == null) name = ""
if (status == null) status = DataSourceStatus.SdkUnknown("no value provided")
if (type == null) type = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy