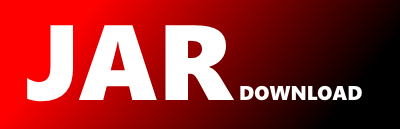
commonMain.aws.sdk.kotlin.services.datazone.model.NotificationOutput.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datazone-jvm Show documentation
Show all versions of datazone-jvm Show documentation
The AWS SDK for Kotlin client for DataZone
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datazone.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* The details of a notification generated in Amazon DataZone.
*/
public class NotificationOutput private constructor(builder: Builder) {
/**
* The action link included in the notification.
*/
public val actionLink: kotlin.String = requireNotNull(builder.actionLink) { "A non-null value must be provided for actionLink" }
/**
* The timestamp of when a notification was created.
*/
public val creationTimestamp: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.creationTimestamp) { "A non-null value must be provided for creationTimestamp" }
/**
* The identifier of a Amazon DataZone domain in which the notification exists.
*/
public val domainIdentifier: kotlin.String = requireNotNull(builder.domainIdentifier) { "A non-null value must be provided for domainIdentifier" }
/**
* The identifier of the notification.
*/
public val identifier: kotlin.String = requireNotNull(builder.identifier) { "A non-null value must be provided for identifier" }
/**
* The timestamp of when the notification was last updated.
*/
public val lastUpdatedTimestamp: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.lastUpdatedTimestamp) { "A non-null value must be provided for lastUpdatedTimestamp" }
/**
* The message included in the notification.
*/
public val message: kotlin.String = requireNotNull(builder.message) { "A non-null value must be provided for message" }
/**
* The metadata included in the notification.
*/
public val metadata: Map? = builder.metadata
/**
* The status included in the notification.
*/
public val status: aws.sdk.kotlin.services.datazone.model.TaskStatus? = builder.status
/**
* The title of the notification.
*/
public val title: kotlin.String = requireNotNull(builder.title) { "A non-null value must be provided for title" }
/**
* The topic of the notification.
*/
public val topic: aws.sdk.kotlin.services.datazone.model.Topic? = builder.topic
/**
* The type of the notification.
*/
public val type: aws.sdk.kotlin.services.datazone.model.NotificationType = requireNotNull(builder.type) { "A non-null value must be provided for type" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datazone.model.NotificationOutput = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("NotificationOutput(")
append("actionLink=*** Sensitive Data Redacted ***,")
append("creationTimestamp=$creationTimestamp,")
append("domainIdentifier=$domainIdentifier,")
append("identifier=$identifier,")
append("lastUpdatedTimestamp=$lastUpdatedTimestamp,")
append("message=*** Sensitive Data Redacted ***,")
append("metadata=$metadata,")
append("status=$status,")
append("title=*** Sensitive Data Redacted ***,")
append("topic=$topic,")
append("type=$type")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = actionLink.hashCode()
result = 31 * result + (creationTimestamp.hashCode())
result = 31 * result + (domainIdentifier.hashCode())
result = 31 * result + (identifier.hashCode())
result = 31 * result + (lastUpdatedTimestamp.hashCode())
result = 31 * result + (message.hashCode())
result = 31 * result + (metadata?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (title.hashCode())
result = 31 * result + (topic?.hashCode() ?: 0)
result = 31 * result + (type.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as NotificationOutput
if (actionLink != other.actionLink) return false
if (creationTimestamp != other.creationTimestamp) return false
if (domainIdentifier != other.domainIdentifier) return false
if (identifier != other.identifier) return false
if (lastUpdatedTimestamp != other.lastUpdatedTimestamp) return false
if (message != other.message) return false
if (metadata != other.metadata) return false
if (status != other.status) return false
if (title != other.title) return false
if (topic != other.topic) return false
if (type != other.type) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datazone.model.NotificationOutput = Builder(this).apply(block).build()
public class Builder {
/**
* The action link included in the notification.
*/
public var actionLink: kotlin.String? = null
/**
* The timestamp of when a notification was created.
*/
public var creationTimestamp: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The identifier of a Amazon DataZone domain in which the notification exists.
*/
public var domainIdentifier: kotlin.String? = null
/**
* The identifier of the notification.
*/
public var identifier: kotlin.String? = null
/**
* The timestamp of when the notification was last updated.
*/
public var lastUpdatedTimestamp: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The message included in the notification.
*/
public var message: kotlin.String? = null
/**
* The metadata included in the notification.
*/
public var metadata: Map? = null
/**
* The status included in the notification.
*/
public var status: aws.sdk.kotlin.services.datazone.model.TaskStatus? = null
/**
* The title of the notification.
*/
public var title: kotlin.String? = null
/**
* The topic of the notification.
*/
public var topic: aws.sdk.kotlin.services.datazone.model.Topic? = null
/**
* The type of the notification.
*/
public var type: aws.sdk.kotlin.services.datazone.model.NotificationType? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datazone.model.NotificationOutput) : this() {
this.actionLink = x.actionLink
this.creationTimestamp = x.creationTimestamp
this.domainIdentifier = x.domainIdentifier
this.identifier = x.identifier
this.lastUpdatedTimestamp = x.lastUpdatedTimestamp
this.message = x.message
this.metadata = x.metadata
this.status = x.status
this.title = x.title
this.topic = x.topic
this.type = x.type
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datazone.model.NotificationOutput = NotificationOutput(this)
/**
* construct an [aws.sdk.kotlin.services.datazone.model.Topic] inside the given [block]
*/
public fun topic(block: aws.sdk.kotlin.services.datazone.model.Topic.Builder.() -> kotlin.Unit) {
this.topic = aws.sdk.kotlin.services.datazone.model.Topic.invoke(block)
}
internal fun correctErrors(): Builder {
if (actionLink == null) actionLink = ""
if (creationTimestamp == null) creationTimestamp = Instant.fromEpochSeconds(0)
if (domainIdentifier == null) domainIdentifier = ""
if (identifier == null) identifier = ""
if (lastUpdatedTimestamp == null) lastUpdatedTimestamp = Instant.fromEpochSeconds(0)
if (message == null) message = ""
if (title == null) title = ""
if (type == null) type = NotificationType.SdkUnknown("no value provided")
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy